Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Automatically Authenticating after Registration
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
Automatically Authenticating after Registration¶
After registration, let’s log the user in automatically. Create a private function called authenticateUser inside RegisterController. Normally, authentication happens automatically, but we can also trigger it manually:
// src/Yoda/UserBundle/Entity/Controller/RegisterController.php
// ...
use Symfony\Component\Security\Core\Authentication\Token\UsernamePasswordToken;
private function authenticateUser(User $user)
{
$providerKey = 'secured_area'; // your firewall name
$token = new UsernamePasswordToken($user, null, $providerKey, $user->getRoles());
$this->container->get('security.context')->setToken($token);
}
This code might look strange, and I don’t want you to worry about it too much. The basic idea is that we create a token, which holds details about the user, and then pass this into Symfony’s security system.
Call this method right after registration:
// src/Yoda/UserBundle/Entity/Controller/RegisterController.php
// ...
if ($form->isValid()) {
// .. code that saves the user, sets the flash message
$this->authenticateUser($user);
$url = $this->generateUrl('event');
return $this->redirect($url);
}
Try it out! After registration, we’re redirected back to the homepage. But if you check the web debug toolbar, you’ll see that we’re also authenticated as Padmé. Sweet!
Redirecting back to the original URL
Suppose an anonymous user tries to access a page and is redirected to /login. Then, they register for a new account. After registration, wouldn’t it be nice to redirect them back to the page they were originally trying to access?
Yes! And that’s possible because Symfony stores the original, protected URL in the session:
$key = '_security.'.$providerKey.'.target_path';
$session = $this->getRequest()->getSession();
// get the URL to the last page, or fallback to the homepage
if ($session->has($key)) {
$url = $session->get($key)
$session->remove($key);
} else {
$url = $this->generateUrl('homepage');
}
The session storage key used here is pretty internal, and could change in the future. So use with caution!
4 Comments
Thanks! You're absolutely right: though the old way will still work until Symfony 3.0 (but you'll start to see these pesky deprecation warnings). The above code will remove those warnings.
Cheers!
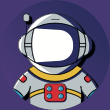
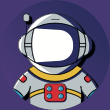
use Symfony\Component\Security\Core\Authentication\Token\UsernamePasswordToken;
I see we were missing that use statement in the code block. I've just fixed that.
Thanks!
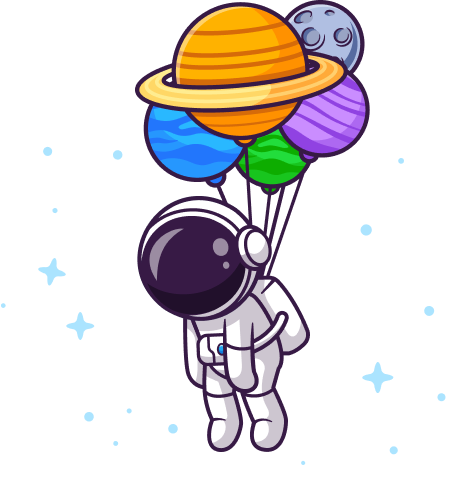
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1" // 4.1.0
}
}
Since symfony 2.6, the code in the <strong>authenticateUser</strong> method should be:
Read more in this announcement http://symfony.com/blog/new-in-symfony-2-6-security-component-improvements.