Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Saving Users
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
Saving Users¶
Now that the error is gone, try logging in! Wait, but our user table is empty. So we can see the bad password message, but we can’t actually log in yet.
But we’re pros, so it’s no problem. Let’s copy the LoadEvents fixtures class (LoadEvents.php) into the UserBundle, rename it to LoadUsers, and update the namespaces:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
namespace Yoda\UserBundle\DataFixtures\ORM;
use Doctrine\Common\DataFixtures\FixtureInterface;
use Doctrine\Common\Persistence\ObjectManager;
use Yoda\UserBundle\Entity\User;
class LoadUsers implements FixtureInterface
{
public function load(ObjectManager $manager)
{
// todo
}
}
Saving users is almost easy: just create the object, give it a username and then persist and flush it.
The tricky part is that darn password field, which needs to be encoded with bcrypt:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
// ...
use Yoda\UserBundle\Entity\User;
// ...
public function load(ObjectManager $manager)
{
$user = new User();
$user->setUsername('darth');
// todo - fill in this encoded password... ya know... somehow...
$user->setPassword('');
$manager->persist($user);
// the queries aren't done until now
$manager->flush();
}
ContainerAwareInterface for Fixtures¶
But what’s cool is that Symfony gives us an object that can do all that encoding for us. To get it, first make the fixture implement the ContainerAwareInterface:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
// ...
use Symfony\Component\DependencyInjection\ContainerAwareInterface;
class LoadUsers implements FixtureInterface, ContainerAwareInterface
{
// ...
}
This requires one new method - setContainer. In it, we’ll store the $container variable onto a new $container property:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
// ...
use Symfony\Component\DependencyInjection\ContainerAwareInterface;
use Symfony\Component\DependencyInjection\ContainerInterface;
class LoadUsers implements FixtureInterface, ContainerAwareInterface
{
private $container;
// ...
public function setContainer(ContainerInterface $container = null)
{
$this->container = $container;
}
}
Because we implement this interface, Symfony calls this method and passes us the container object before calling load. Remember that the container is the array-like object that holds all the useful objects in the system. We can see a list of those object by running the container:debug console task:
php app/console container:debug
Encoding the Password¶
Let’s create a helper function called encodePassword to you know encode the password! This step may look strange, but stay with me. First, we ask Symfony for a special “encoder” object that knows how to encrypt our passwords. Remember the bcrypt config we put in security.yml? Yep, this object will use that.
After we grab the encoder, we just call encodePassword(), grab a sandwich and let it do all the work:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
// ...
private function encodePassword(User $user, $plainPassword)
{
$encoder = $this->container->get('security.encoder_factory')
->getEncoder($user)
;
return $encoder->encodePassword($plainPassword, $user->getSalt());
}
Behind the scenes, it takes the plain-text password, generates a random salt, then encrypts the whole thing using bcrypt. Ok, so let’s set this onto the password property:
// src/Yoda/UserBundle/DataFixtures/ORM/LoadUsers.php
// ...
public function load(ObjectManager $manager)
{
$user = new User();
$user->setUsername('darth');
$user->setPassword($this->encodePassword($user, 'darthpass'));
$manager->persist($user);
// the queries aren't done until now
$manager->flush();
}
Try it! Reload the fixtures from the command line:
php app/console doctrine:fixtures:load
Let’s use the query console task to look at what the user looks like:
php app/console doctrine:query:sql "SELECT * FROM yoda_user"
array (size=1)
0 =>
array (size=3)
'id' => string '1' (length=1)
'username' => string 'user' (length=4)
'password' => string '$2y$13$BoVE3I5dmVkBjRp.l6uwyOI8Z8Ngokiaa.OUUuHoDbGDBdMRMUrmC' (length=60)
Nice! We can see the encoded password, which for bcrypt, also includes the randomly-generated salt. You do need to store the salt for each user, but with bcrypt, it happens automatically. Symfony requires us to have a getSalt function on our User, but it’s totally not needed with bcrypt.
Back at the browser, we can login! Behind the scenes, here’s basically what’s happening:
- A User entity is loaded from the database for the given username;
- The plain-text password we entered is encoded with bcrypt;
- The encoded version of the submitted password is compared with the saved password field. If they match, then you now have access to roam about this fully armed and operational battle station!
14 Comments
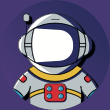
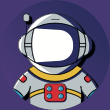
Damn, I'm such a noob!
I finally solve the problem, by replacing the "DELETE" method with "GET". And boom, it worked like a charm!
I hope someone will find this helpful! ... and I'm glad I've "enlighted" myself!
But high-five KNP, just in case! :)
Hey Dan,
Actually, you did it right, but there's more robust solution with a form which sends with DELETE method.
The "@Method("DELETE")" annotation means that you can send only DELETE request to this route, i.e. you can't send DELETE request with a link because it's just a GET request. So there're a few solutions:
1. Change "@Method("DELETE")" to the @Method("GET") because you delete posts by clicking a link. - Like you did
2. Replace delete link in the template with a <form method="DELETE"></form>, because you can send DELETE requests only with form. Of course, you need to add a submit button to this form and also generate the action attribute which points to the deleteAction().
The 2nd solution is more robust, because with the 1st one you can accidently go directly to the delete action and remove a post which you don't want to delete.
Cheers!
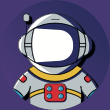
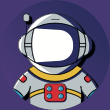
Hi Victor, thanks for replying!
I tried your second solution too, but I couldn't figure out how to add a different id for each delete_form form.
Tried something like:
// loop through posts
// ...
<a href="{{ form(delete_form, { 'id':post.id }) }}">Delete</a>
// ...
// endloop
And I used both deleteAction(Request $request, $id) and createDeleteForm($id) generated by CRUD.
I ended up with just one delete button, and I needed one for each post.
It's simple enough - just create a separate form for each post row as you do with links ;)
{% for post in posts %}
<form action="{{ path('post_delete', {id: post.id}) }}" method="DELETE">
<input type="submit">
</form>
{% endfor %}
i.e. use its own separate form for each product row.
Cheers!
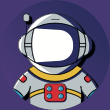
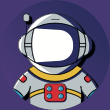
I'll give it a try. Thanks a lot!
And one more question if you don't mind to answer it.
I have, for this part of my template, the need to hide some links (edit and delete) if the current logged in user is not the owner of the respective post (I have a ManyToOne relationship)
Like:
Users
-------
id username
1 abc
2 def
Posts
-------
id body user_id
1 ... 1
2 ... 1
3 ... 2
How to achieve this?
You could easily check permissions with something like {% if app.user.id == post.user.id %}
- then show links, but let's use something cooler! Look at <a href="http://symfony.com/doc/current/security/voters.html">Symfony Voters</a> - it's exactly what you need. then you can check whether the current user has access to a post with is_granted()
Twig function. And hey, we have a free course on it: <a href="https://knpuniversity.com/screencast/symfony-voters">Symfony Security Voters</a>. And one more useful screencast about voters with a nice example inside: https://knpuniversity.com/screencast/new-in-symfony3/voter .
Cheers!
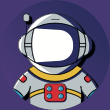
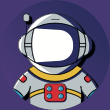
I'm following this tutorial. But I get lost in "Let’s copy the LoadEvents fixtures class (LoadEvents.php)".
Hey there!
Yea, I realize that's a little vague - sorry about that :). In this step, I copy the entire DataFixtures directory from EventBundle (which contains the LoadEvents.php file we created earlier) and copy it into UserBundle. I just did this to save me time from creating the DataFixtures/ORM directory in UserBundle and creating the new LoadUsers.php file by hand.
I hope that helps!
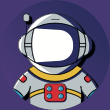
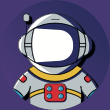
Little Question, If I get the $25 monthly acces. I get "Access to the full KnpUniversity course library". So that includes this tutorial in particular??? Or the current month tutorials??? Cause I want THIS course in particular. And also the episode 1(cause I check thats includes the datafixture part) But I'm not sure if I get them with the monthly acces.
You'll get access to *everything* while your subscription is active - including this and episode 1... and also all of the newer Symfony 3 tutorials if you want those :)
Of course :) - I always keep an eye on the comments!
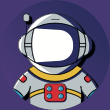
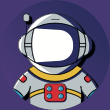
and I have ALL THE POWERRRRRR!!! I got my suscription :D. This is gonna be a hell of a good weekend.
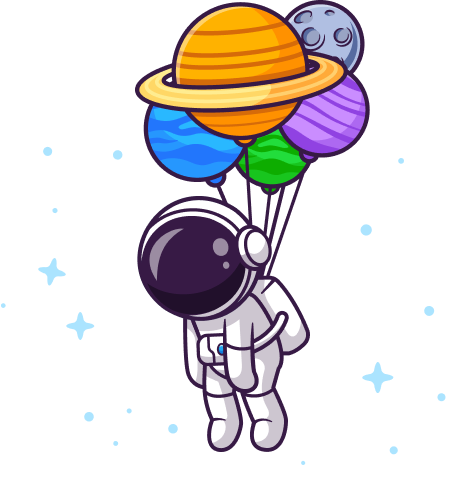
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1" // 4.1.0
}
}
Hey KNP! I'm sorry for bothering you again!
So I need to create a custom deleteAction() method. My reliable friend, google, didn't cared about my problem, unfortunately. Muf! Muf!
Anyway, here's what I've wrote until now:
`
/**
`
And in my twig template:
<br />// for post in posts<br />// ...<br /><a href="{{ path('post_delete', { 'id':post.id }) }}">Delete</a><br />// ...<br />// endfor<br />
But I'm getting the following error:
<br />No route found for "GET /post/13": Method Not Allowed (Allow: DELETE)<br />
I also copied and adapted to my case, the code from a generated CRUD. It didn't worked!
Help, I'm in panic!