Fun with Symfony's Console Component
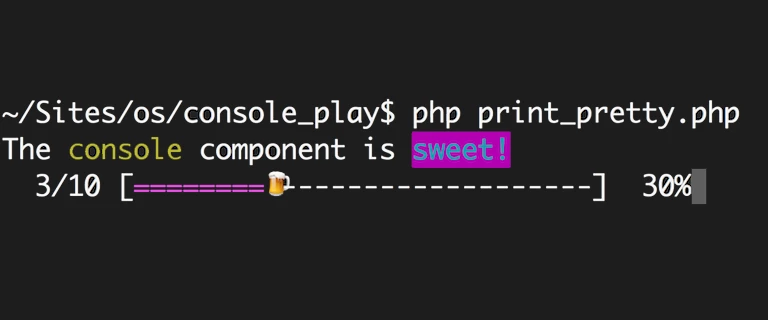
One of the best parts of using Symfony's Console component is all the output control you have to the CLI: colors, tables, progress bars etc. Usually, you create a command to do this. But what you may not know is that you can get to all this goodness in a single, flat PHP file
Using ConsoleOutput in a Flat File
Suppose you've started a new project and want to write a CLI script with some added
flare. First, just grab the symfony/console
component:
composer require symfony/console
Next, open up a flat php file, require the autoloader and create a new
ConsoleOutput
object:
// print_pretty.php
require __DIR__.'/vendor/autoload.php';
use Symfony\Component\Console\Output\ConsoleOutput;
use Symfony\Component\Console\Formatter\OutputFormatter;
$output = new ConsoleOutput();
$output->setFormatter(new OutputFormatter(true));
$output->writeln(
'The <comment>console</comment> component is'
.' <bg=magenta;fg=cyan;option=blink>sweet!</>'
);
The ConsoleOutput
class is the heart of all the styling control. And when used
with the OutputFormatter
, you're able to print text with built-in styles for
<comment>
, <info>
, <error>
and <question>
. Or, you can control the background
and foreground colors manually.
Ok, let's run this from the command line!
php print_pretty.php
That's right, it's blinking. You can also wrap up your custom colorings and options
(like our blinking cyan section) into a new style (e.g. <annoying_blinking>
).
See OutputFormatter
and OutputFormatterStyle
for details.
How about Table and a Progress Bar?
I'm so glad you asked! With the ConsoleOutput
object, using any of the other
Console Helpers
is easy! Let's build a table with a progress bar that shows how things are going:
// print_pretty.php
use Symfony\Component\Console\Helper\ProgressBar;
use Symfony\Component\Console\Helper\Table;
// ... all the other code from before
$rows = 10;
$progressBar = new ProgressBar($output, $rows);
$progressBar->setBarCharacter('<fg=magenta>=</>');
$progressBar->setProgressCharacter("\xF0\x9F\x8D\xBA");
$table = new Table($output);
for ($i = 0; $i<$rows; $i++) {
$table->addRow([
sprintf('Row <info># %s</info>', $i),
rand(0, 1000)
]);
usleep(300000);
$progressBar->advance();
}
$progressBar->finish();
$output->writeln('');
$table->render();
Moment of truth:
php print_pretty.php
Check out the animated gif below!
Tip
Yes, that is a beer icon :). You can find more icons here.
Just use the UTF-8 value - e.g. 0xF0 0x9F 0x8D 0xBA
or f09f8dba
and put it into
the \xF0\x9F\x8D\xBA
format.
That's just another trick hidden inside Symfony. If you want to learn more, check out our entire Symfony track!
Have fun!
6 Comments
I see that You have a problem with code formatting on this page : http://cl.ly/2E3j3a3X2D3Q
Ah, thank you! Change one thing in CSS, break something else :). I've just fixed this.
LOL! I think this is the best interpretation of the symfony progress bar :D