Chapters
-
Course Code
Subscribe to download the code!
Subscribe to download the code!
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Setting up Webpack Encore
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
So let's get Webpack Encore installed so we can get a proper CSS and JavaScript build system set up.
Installing Encore
Find your terminal and run:
composer require "encore:^1.14"
Tip
If you're using version 2 or higher of symfony/webpack-encore-bundle
, be sure to also
run:
composer require symfony/stimulus-bundle
The recipe needed to integrate the Symfony UX libraries was moved to this new bundle.
This really installs WebpackEncoreBundle, which will give us a few Twig helper functions. But its true superpower is its recipe.
The Encore Recipe Files
After Composer finishes, run:
git status
Ok: it modified several files, most of which make sense. The .gitignore
file is now ignoring the node_modules/
directory and the bundle was automatically enabled in config/bundles.php
.
There are also several new config files, which mostly aren't too important. What is important is that it created a package.json
file. Let's go check that out. This defines several things that our Webpack Encore setup needs, like Encore itself and stimulus
, which we'll talk more about later.
The other hugely important file the recipe added was webpack.config.js
- with a basic setup. The recipe also added this assets/
directory. There are a few things in here related to Stimulus: the "controllers" stuff and bootstrap.js
. We'll talk about those soon.
To download the dependencies described in package.json
, find your terminal and run:
yarn install
Oh, and if you're totally new to Encore and want to dig into it deeper, check out our Webpack Encore: Frontend like a Pro! course.
The Entry Files and First Build
Right now, our app has a pretty standard Webpack Encore setup. We have one entry which is this assets/app.js
file. That means that when we build our assets in a few minutes, Webpack will only look at this file to figure out all the JavaScript and CSS needed in the project. It will then output the final, built CSS and JavaScript files into a public/build/
directory.
Of course, when it reads this file, it will follow any imports, like this import of ./styles/app.css
... which is a dummy file that sets the background-color
to gray.
So, once we have executed Encore and built the assets - which we'll do in a minute - we're going to need script
and link
tags that point to those new files.
encore_entry_link_tags() & encore_entry_script_tags()
Let's add those to our base layout: templates/base.html.twig
.
I already have a couple of link tags up here in the stylesheets
block. Add {{ encore_entry_link_tags() }}
and pass it app
, which is name of that entry.
Show Lines
|
// ... lines 1 - 2 |
<head> | |
Show Lines
|
// ... lines 4 - 5 |
{% block stylesheets %} | |
Show Lines
|
// ... lines 7 - 9 |
{{ encore_entry_link_tags('app') }} | |
{% endblock %} | |
Show Lines
|
// ... lines 12 - 15 |
</head> | |
Show Lines
|
// ... lines 17 - 76 |
This will render the built version of the app.css
file plus any other CSS that our JavaScript imports. And actually, for performance reasons, Webpack may split that built app.css
into multiple files: we'll see that in a few minutes. This function takes care of including all the link tags we need.
Do the same thing in the javascripts
block: {{ encore_entry_script_tags() }}
and pass app
.
Show Lines
|
// ... lines 1 - 2 |
<head> | |
Show Lines
|
// ... lines 4 - 12 |
{% block javascripts %} | |
{{ encore_entry_script_tags('app') }} | |
{% endblock %} | |
</head> | |
Show Lines
|
// ... lines 17 - 76 |
Like with the styles, this will render the built version of app.js
plus any JavaScript it imports. And it may also be split into multiple files.
The New <script>
defer Attribute
Oh, and you're seeing a recent change in Symfony's TwigBundle recipe for base.html.twig
. The javascripts
block used to live down here at the bottom of the page. Now it lives up in the head
in new projects. Normally... that would not be a good idea, because when your browser sees a script
tag, it stops rendering the page until it can download and execute that JavaScript.
But thanks to a new feature in WebpackEncoreBundle - you can see it in config/packages/webpack_encore.yaml
, here it is script_attributes
- every script tag rendered by Encore will have a defer
attribute. That basically means that the JavaScript isn't executed until after the page loads, very similar to having the scripts
at the bottom of the page. But with the new setup, our browser does start downloading the files slightly earlier. And this plays nicer with Turbo - the topic of our next tutorial.
If you want to learn more about this change, check out a blog post I wrote on Symfony.com.
Next: let's use Encore to build our assets, see "code splitting" in action and import a third-party CSS package.
50 Comments
...Package sensio/framework-extra-bundle is abandoned, you should avoid using it. Use Symfony instead.
Generating optimized autoload files
78 packages you are using are looking for funding.
Use the `composer fund` command to find out more!
Symfony operations: 1 recipe (e7b2756031f9cac8a16f25d37c761953)
- Configuring symfony/stimulus-bundle (>=2.13): From github.com/symfony/recipes:main
Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 255
!!
!! Fatal error: Declaration of Symfony\Component\String\Slugger\AsciiSlugger::getLocale() must be compatible with Symfony\Contracts\Translation\LocaleAwareInterface::getLocale(): string in /Users/alphacentauri/Documents/symfony/code-stimulus/start/vendor/symfony/string/Slugger/AsciiSlugger.php on line 93
!! PHP Fatal error: Declaration of Symfony\Component\String\Slugger\AsciiSlugger::getLocale() must be compatible with Symfony\Contracts\Translation\LocaleAwareInterface::getLocale(): string in /Users/alphacentauri/Documents/symfony/code-stimulus/start/vendor/symfony/string/Slugger/AsciiSlugger.php on line 93
!! Symfony\Component\ErrorHandler\Error\FatalError {#4148
!! #message: "Compile Error: Declaration of Symfony\Component\String\Slugger\AsciiSlugger::getLocale() must be compatible with Symfony\Contracts\Translation\LocaleAwareInterface::getLocale(): string"
!! #code: 0
!! #file: "./vendor/symfony/string/Slugger/AsciiSlugger.php"
!! #line: 93
!! -error: array:4 [
!! "type" => 64
!! "message" => "Declaration of Symfony\Component\String\Slugger\AsciiSlugger::getLocale() must be compatible with Symfony\Contracts\Translation\LocaleAwareInterface::getLocale(): string"
!! "file" => "/Users/alphacentauri/Documents/symfony/code-stimulus/start/vendor/symfony/string/Slugger/AsciiSlugger.php"
!! "line" => 93
!! ]
!! }
!!
Script @auto-scripts was called via post-update-cmd
Failed to install packages for ./composer.json.
Hey Hans,
Try to manually remove the cache dir, i.e. run: rm -rf var/cache/
and try again. Btw, if you're trying to use composer.json file from the Vladyslav comment above - you may need to drop your composer.lock file first before installing the dependencies probably, something that is worth trying. Otherwise, you may need to update the dependencies, the current set of dependencies is causing some conflicts.
I hope that helps!
Cheers!
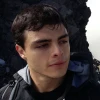
Hey @Vladyslav-Chernyshov
Would it work if you just run composer upgrade
before installing the package?
Thanks for sharing your solution. Cheers!
Unfortunately not. Only if you'll replace all "5.2.*"
to "5.*"
. Version 5.4.*
needed.
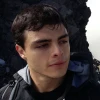
Got it, the version constraint needs to be updated too


Hi All,
When I run symfony composer require symfony/stimulus-bundle
I have this error
Problem 1
- Root composer.json requires symfony/stimulus-bundle ^2.17 -> satisfiable by symfony/stimulus-bundle[v2.17.0, 2.x-dev].
- symfony/stimulus-bundle[v2.17.0, ..., 2.x-dev] require symfony/config ^5.4|^6.0|^7.0 -> found symfony/config[v5.4.0-BETA1, ..., 5.4.x-dev, v6.0.0-BETA1, ..., 6.4.x-dev, v7.0.0-BETA1, ..., 7.1.x-dev] but the package is fixed to v5.2.12 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
Use the option --with-all-dependencies (-W) to allow upgrades, downgrades and removals for packages currently locked to specific versions.
You can also try re-running composer require with an explicit version constraint, e.g. "composer require symfony/stimulus-bundle:*" to figure out if any version is installable, or "composer require symfony/stimulus-bundle:^2.1" if you know which you need.
Hey Amine,
That makes sense as you can see this course is based on Symfony v5.2. The symfony/stimulus-bundle requires at least Symfony 5.4, so to be able to install it - you need to upgrade your dependencies first. The error message already suggest you a possible solution: re-run that command with --with-all-dependencies
, but it still may not be enough, you need to make sure you allow upgrading to Symfony 5.4 in your composer.json file. This upgrade may also require more work from you, but in theory this minor upgrade should not contain any BC breaks.
Cheers!


Hi Victor,
That's true. I can show the site right now, but I still have one error (which I commented directly in the vendor).
- LocaleAwareInterface
- public function getLocale(); instead of public function getLocale(): string;
Hey Amine,
Glad to hear you got it working! Though it's a bad idea to do any changes in the vendor/ dir. Seems you need to upgrade more packages because their versions mismatch, take a look at the package version where you see that error, and at the interface it implements from for getLocale()
, I think you just need to update more packages :)
Cheers!
Git status is fine :) Buuuuuutt git not initialized and no docs about it ..... Houpe it help to create Best Of The Best Tutorial
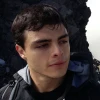
Hey Mepcuk
Yea, you're right, we don't say anything about git in the README, though, it's quite simple, you only have to run git init
on the root of your project and answer the questions. I hope it helps
Cheers!
Thanks a lot, i know... but if this tutorial will watch young developer, it maybe create him a problem. Just all tuto is step by step, there are look missing small step..
Thanks for reply 😉
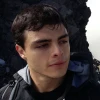
Yea, I agree. I'm going to talk about it with the team. Thanks for your feedback!


When i do Yarn Watch all i get is..
PS C:\xampp\htdocs\symfonyux> yarn watch
Debugger listening on ws://127.0.0.1:60101/eeffdea6-e21c-4850-b402-7b92f3d497ae
For help, see: https://nodejs.org/en/docs/inspector
Debugger attached.
yarn run v1.22.22
When
$ encore dev --watch
Debugger listening on ws://127.0.0.1:60104/4908a24e-7845-4f25-8087-cebb74f54ed1
For help, see: https://nodejs.org/en/docs/inspector
Debugger attached.
Debugger ending on ws://127.0.0.1:60104/4908a24e-7845-4f25-8087-cebb74f54ed1
For help, see: https://nodejs.org/en/docs/inspector
Waiting for the debugger to disconnect...
No build directory is made but when i did do yarn install prior i did get allot of warnings.
info No lockfile found.
[1/4] Resolving packages...
warning @symfony/webpack-encore > webpack-dev-server > rimraf@3.0.2: Rimraf versions prior to v4 are no longer supported
warning @symfony/webpack-encore > clean-webpack-plugin > del > rimraf@2.7.1: Rimraf versions prior to v4 are no longer supported
warning @symfony/webpack-encore > webpack-dev-server > rimraf > glob@7.2.3: Glob versions prior to v9 are no longer supported
warning @symfony/webpack-encore > clean-webpack-plugin > del > rimraf > glob@7.2.3: Glob versions prior to v9 are no longer supported
warning @symfony/webpack-encore > clean-webpack-plugin > del > globby > glob@7.2.3: Glob versions prior to v9 are no longer supported
warning @symfony/webpack-encore > webpack-dev-server > webpack-dev-middleware > memfs@3.6.0: this will be v4
warning @symfony/webpack-encore > webpack-dev-server > rimraf > glob > inflight@1.0.6: This module is not supported, and leaks memory. Do not use it. Check out lru-cache if you want a good and tested way to coalesce async requests by a key value, which is much more comprehensive and powerful.
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve@0.5.3: See https://github.com/lydell/source-map-resolve#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > urix@0.1.0: Please see https://github.com/lydell/urix#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve > urix@0.1.0: Please see https://github.com/lydell/urix#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve > resolve-url@0.2.1: https://github.com/lydell/resolve-url#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve > source-map-url@0.4.1: See https://github.com/lydell/source-map-url#deprecated
warning @symfony/webpack-encore > css-minimizer-webpack-plugin > cssnano > cssnano-preset-default > postcss-svgo > svgo > stable@0.1.8: Modern JS already guarantees Array#sort() is a stable sort, so this library is deprecated. See the compatibility table on MDN: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort#browser_compatibility
[2/4] Fetching packages...
[3/4] Linking dependencies...
[4/4] Building fresh packages...
success Saved lockfile.
Done in 34.90s.
Any suggestions.
Hey Stuart,
I see you're on Windows, I personally haven't had an experience with Yarn and Node on it, but Windows might be the problem. I wonder if Node / Yarn works in your other projects? Also, I see your Yarn version is v1.22.22, but what version of NodeJS do you have? node --version
should show it to you.
Seeing some warnings during the yarn install
is OK, and as I see from your output the install command was successfully finished and created the lock file without errors (warnings no count).
So, you need to install deps with the yarn install
first, and then run the yarn watch
, it still does not work for you? Could you try just yarn dev
or yarn build
commands? Do you have any errors executing them? Do you see the build dir after them? Probably you have problems on Windows with the listener that watches changes.
Cheers!


Node version is v22.3.0
I am also going to bring by version of PHP down to 7.4 I believe is better for Yarn.
Must be one of few that prefer PC for learning code..
Hey Stuart,
I don't think your PHP version is important to Yarn / Node as those are JS tools anyway. I just upgrade node and yarn locally to the latest:
$ yarn --version
1.22.22
$ node --version
v22.4.0
Then I downloaded the course code, moved to the finish/ directory, and ran next command:
$ composer install
$ yarn install
$ yarn watch
And indeed I see an issue with running yarn watch
on this course project code, the error is:
Running webpack ...
node:internal/crypto/hash:71
this[kHandle] = new _Hash(algorithm, xofLen);
^
Error: error:0308010C:digital envelope routines::unsupported
at new Hash (node:internal/crypto/hash:71:19)
at Object.createHash (node:crypto:130:10)
at BulkUpdateDecorator.hashFactory (/Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/util/createHash.js:144:18)
at BulkUpdateDecorator.digest (/Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/util/createHash.js:79:21)
at NormalModule._initBuildHash (/Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/NormalModule.js:757:53)
at /Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/NormalModule.js:795:10
at processResult (/Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/NormalModule.js:605:12)
at /Users/victor/Downloads/code-stimulus (1)/finish/node_modules/webpack/lib/NormalModule.js:692:5
at /Users/victor/Downloads/code-stimulus (1)/finish/node_modules/loader-runner/lib/LoaderRunner.js:399:11
at /Users/victor/Downloads/code-stimulus (1)/finish/node_modules/loader-runner/lib/LoaderRunner.js:251:18 {
opensslErrorStack: [ 'error:03000086:digital envelope routines::initialization error' ],
library: 'digital envelope routines',
reason: 'unsupported',
code: 'ERR_OSSL_EVP_UNSUPPORTED'
}
error Command failed with exit code 1.
info Visit https://yarnpkg.com/en/docs/cli/run for documentation about this command.
First of all, seems this tutorial works only on legacy Node v16 version, so I downgraded my Node js (actually installed legacy version along with the latest and temporarily switched to the v16, or v16.20.2 to be specified). And then instead of yarn watch
you need to run:
NODE_OPTIONS=--openssl-legacy-provider yarn watch
to fix a problem due to a change in the default OpenSSL version used by Node.js starting from version 17. This change can cause issues with Webpack, which relies on certain cryptographic functions.
So this way yarn commands work for me, but I'm on Mac. You can try this on Windows, but if it will not work - you will need to export that NODE_OPTIONS=--openssl-legacy-provider
env var somehow in the CLI and then run yarn commands. AI suggests me that this way should work on Windows:
set NODE_OPTIONS=--openssl-legacy-provider
yarn watch
But once again, you would probably need to downgrade your Node version to v16.
I understand that it's not an ideal solution for you but still a valid workaround. We will try to fix this properly for other Node versions, but I don't have any estimations for this. I hope that helps!
Cheers!


Thank you for all your time and assistance. I'll try out your suggestions and let you know.
Much appreciated 👏
I'm ridiculously excited about not needing this step and using AssetMapper instead. In the meantime, is it possible to use both AssetMapper and WebpackEncore in the same project, while migrating to just AssetMapper?
I know, I need to be patient and wait for https://symfonycasts.com/screencast/asset-mapper/stimulus -- can't wait!
Hey @Tac-Tacelosky!
I'm ridiculously excited about not needing this step
There are a few rough edges still (which we talk about in the tutorial), but yea, it's fun :).
In the meantime, is it possible to use both AssetMapper and WebpackEncore in the same project, while migrating to just AssetMapper?
Probably not. Well, it definitely is possible, but I think it will be too easy to "cross wires" both in the code and mentally for this to be worth it. Migrating is both simple but tedious:
A) Most items (everything you actually USE in your JavaScript, so everything except for encore, & other build toolds) in package.json
should be added to importmap.php
via importmap:require
B) All ./relative
imports need to change to ./relative.js
C) CSS needs to be updated to add a link
tag to base.html.twig
(and anywhere you're importing CSS from inside a JS file needs to be reworked).
... and I think that might be it. I did it on ux.symfony.com and Jolicode did it on a fun app they built - https://github.com/jolicode/qotd/pull/53. The reason I won't typically recommend that people switch (unless they really want to) is that it's a bunch of work that takes you from a functional system to a functional system :P. Not a lot of immediate gain. But if the build system is giving you a headache, then it could totally be worth it.
Cheers!


I was using PHP 8.0 (which the composer,json seems to support) but the flex recipes wouldn't run. This prevented me from running `yarn install` because the package.json didn't exist (along with other config changes to the project).
Important note for everyone, be sure to use PHP 7.4 and then run the `composer install` first before moving on through the tutorial.
Hey Chris,
We're sorry for this. Yes, this course should work on PHP 8, you're right. We will take a closer look why it does not work. Could you share a bit more information what exactly was wrong on PHP 8? Did you have any errors in the console?
P.s. thanks for sharing a temporary workaround with others suggesting using PHP 7.4!
Cheers!


No problem. I've just downloaded the project code again. Using the "start" directory.
PHP 8.0.16 - Composer version 2.2.6 2022-02-04 17:00:38 (MacOS 12.2.1, PHP, etc, installed via brew)
`composer install` produces the new "trust" prompt from composer for symfony/flex - I choose 'y'
Composer seems to run just fine. The post-install scripts run (cache:clear, assets:install)
```
~/Downloads/code-stimulus/start
❯ composer require encore
Using version ^1.14 for symfony/webpack-encore-bundle
./composer.json has been updated
Running composer update symfony/webpack-encore-bundle
Loading composer repositories with package information
Updating dependencies
Lock file operations: 1 install, 0 updates, 0 removals
- Locking symfony/webpack-encore-bundle (v1.14.0)
Writing lock file
Installing dependencies from lock file (including require-dev)
Package operations: 1 install, 0 updates, 0 removals
- Installing symfony/webpack-encore-bundle (v1.14.0): Extracting archive
Generating optimized autoload files
75 packages you are using are looking for funding.
Use the `composer fund` command to find out more!
Run composer recipes at any time to see the status of your Symfony recipes.
Executing script cache:clear [OK]
Executing script assets:install public [OK]
```
No evidence the flex recipes have run. No package.json, the bundles.php has not been modified.
However, running the same steps from PHP 7.4.28 and everything works as expected.
I hope this helps.
Hey Chris N.!
Sorry for the short reply - Victor wanted to continue helping you with this, but he's currently unavailable. But I'm happy to fill in :).
This is *super* weird. I just tried it myself and it works. However, 12 days ago (so 2 days after you posted this), we added the little change to composer.json so that Flex is already trusted (without it needing to ask you). But even without this, I can't imagine what difference PHP 7.4 would make vs PHP 8 for this - that is a mystery.
Cheers!


I agree, super weird. I've not had any other Flex related issues - but then, I don't install a fresh project that often. Maybe just a weird combination of packages and bad timing. Composer, Symfony, etc, have all had updates since I first posted this. If I get change, I'll try it again and will only post if it still fails.
Thanks all. Keep up the great work.


Hi,
I have a symfony app with webpack 0.30.0, what is the correct procedure to install stymulus? I am attaching my package.json
{
"devDependencies": {
"@symfony/webpack-encore": "^0.30.0",
"autocomplete.js": "^0.37.1",
"autoprefixer": "^9.8.4",
"axios": "^0.20.0",
"bootstrap": "^4.5.0",
"core-js": "^3.0.0",
"eslint": "^7.6.0",
"eslint-plugin-vue": "^7.0.0-beta.2",
"jquery": "^3.5.1",
"node-sass": "4.13.0",
"popper.js": "^1.16.1",
"postcss-loader": "^3.0.0",
"regenerator-runtime": "^0.13.2",
"sass-loader": "8.0.0",
"vue": "^2.6.11",
"vue-loader": "15.9.1",
"vue-template-compiler": "^2.6.11",
"webpack-notifier": "^1.6.0"
},
"license": "UNLICENSED",
"private": true,
"scripts": {
"dev-server": "encore dev-server",
"dev": "encore dev",
"watch": "encore dev --watch",
"build": "encore production --progress"
},
"dependencies": {
"@sweetalert2/themes": "3.2.0",
"bootstrap-vue": "^2.17.1",
"chart": "^0.1.2",
"chart.js": "^2.9.3",
"color": "^3.1.2",
"css-loader": "^4.2.1",
"eslint-config-airbnb-base": "^14.2.0",
"eslint-plugin-import": "^2.22.0",
"flag-icon-css": "^3.5.0",
"font-awesome": "^4.7.0",
"lodash": "^4.17.20",
"material-design-icons": "^3.0.1",
"material-design-icons-iconfont": "^5.0.1",
"perfect-scrollbar": "^1.5.0",
"progressbar.js": "^1.1.0",
"react": "^16.13.1",
"react-dom": "^16.13.1",
"select2": "^4.0.13",
"sweetalert2": "^9.17.0",
"themify-icons": "^1.0.0",
"ti-icons": "^0.1.2",
"typicons.font": "^2.0.9",
"validate-color": "^2.1.0",
"vue-sweetalert-icons": "^4.3.0",
"vue-sweetalert2": "3.0.8",
"vuedraggable": "^2.24.3",
"x-editable": "^1.5.1"
},
"browserslist": [
"> 5%"
]
}
Thanks
Hey Marco,
First of all, commit all the changes in the project to have a clear repo. Then, upgrade your symfony/webpack-encore-bundle to the latest available version. You can do it with Composer, execute:
$ composer update symfony/webpack-encore-bundle
And make sure you have the latest version installed, currently I see it's v1.12.0.
Then, upgrade its recipe, you can do this with Composer again:
$ composer recipe:install symfony/webpack-encore-bundle --force
And after this use git diff to check the changes, you may want to revert some default code to your custom that you had before, but you want to keep the new changes related to Stimulus. After you validate the changes and sync them together - it should work. Don't forget to run "yarn install" and "yarn watch" after to make sure you pull new Node JS dependencies.
I hope this helps!
Cheers!


I tried this , but afteryarn add bootstrap --dev
get message
<blockquote>error @symfony/webpack-encore@2.1.0: The engine "node" is incompatible with this module. Expected version "^12.13.0 || >=14.0.0". Got "12.5.0"
error Found incompatible module.</blockquote>
My configuration
node v16.16.0, php 8.1.6 npm 8.15.1
How can I solve this better ?
yarn install --ignore-engines
enable to run yarn watch
, but it not perfect i suppose.
Hey Pawel,
Hm, are you sure you have Node v16 installed? Because the error message says that you have v12.5.0. Please, double check the node version. And it seems like you have to upgrade it to the ^12.13.0 at least as it's said in the error message.
I think you can check it with "node --version" in your terminal.
Another possible solution - try to upgrade dependencies, it might help, but also may cause more issue.
Cheers!


yes, command "node -v" return value v16.16.0
Hey Pawel,
Ah, looks like the error is related to the "engine" key in that package.json of that @ symfony/webpack-encore package, for example see that file https://github.com/symfony/... . So, looks like the package installed in the project requires the node 12.5.0 explicitly, and most probably you either need to downgrade your NodeJS to that version, or upgrade dependencies that may cause some issues but probably might be worth to try, or just use that "--ignore-engines" option when you install Yarn deps - it should be OK for learning purposes when you work around the course project code to follow the tutorial.
I hope this helps!
Cheers!


`Running webpack ...
WARNING Webpack Encore requires version ^4.0.0 || ^5.0.0 of postcss-loader, but your version (3.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^4.0.0 || ^5.0.0 of postcss-loader, but your version (3.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^9.0.1 || ^10.0.0 || ^11.0.0 || ^12.0.0 of sass-loader, but your version (8.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^4.0.0 || ^5.0.0 of postcss-loader, but your version (3.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^9.0.1 || ^10.0.0 || ^11.0.0 || ^12.0.0 of sass-loader, but your version (8.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^4.0.0 || ^5.0.0 of postcss-loader, but your version (3.0.0) is too old. The related feature will probably not work correctly.
WARNING Webpack Encore requires version ^15.9.5 of vue-loader, but your version (15.9.1) is too old. The related feature will probably not work correctly.
RECOMMEND To create a smaller (and CSP-compliant) build, see https://symfony.com/doc/current/frontend/encore/vuejs.html#runtime-compiler-build
[webpack-cli] Error: Compiling RuleSet failed: Properties generator are unknown (at ruleSet[0].rules[0].oneOf[1]: [object Object])
at RuleSetCompiler.error (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:373:10)
at RuleSetCompiler.compileRule (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:196:15)
at /Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:154:9
at Array.map (<anonymous>)
at RuleSetCompiler.compileRules (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:153:16)
at RuleSetCompiler.compileRule (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:192:30)
at /Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:154:9
at Array.map (<anonymous>)
at RuleSetCompiler.compileRules (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:153:16)
at RuleSetCompiler.compileRule (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:184:30)
at /Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:154:9
at Array.map (<anonymous>)
at RuleSetCompiler.compileRules (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:153:16)
at RuleSetCompiler.compile (/Users/prest90/development/DataNet/node_modules/webpack/lib/rules/RuleSetCompiler.js:68:22)
at VueLoaderPlugin.apply (/Users/prest90/development/DataNet/node_modules/vue-loader/lib/plugin-webpack5.js:45:39)
at createCompiler (/Users/prest90/development/DataNet/node_modules/webpack/lib/webpack.js:74:12)
error Command failed with exit code 2.
info Visit https://yarnpkg.com/en/docs/cli/run for documentation about this command.
`
i tried but i get the following errors, unfortunately these packages are needed!
i have updated these packages and everything seems to work, now i have to do some testing.
Thank you very much for helping!


Hi, after updating on some older browsers I get the error ReferenceError: Can't find variable: globalThis. Is there any setting in webpack to solve the problem? Thank you
Hey Marco,
Hm, could you link me to the chapter where we have that "globalThis" variable? I can't find it, probably you made a misprint in yoir code? Try to do "git grep globalThis" to find that spot, or search in files with PhpStorm, it should help too. Most probably you just need to fix that misprint :)
Cheers!


I noticed that after the update(self.webpackChunk = self.webpackChunk || [])
has become(globalThis ["webpackChunk"] = globalThis ["webpackChunk"] || [])
could it be something related to Babel?
Hey Marco,
Hm, that file is autogenerated, and so different versions may generate a slightly different code. What exactly it should be - difficult to say, I'd suppose that the code you have in that file is correctly generated by the Webpack. I think you need to find a problem in a different place. First of all, make sure you upgraded your symfony/webpack-encore-bundle which is v1.12.0 for today, you can check it with "composer info symfony/webpack-encore-bundle". Then, sync its recipe in your project. You can do it by leveraging "symfony recipe:installl --force" command or manually, coping the latest changes in the recipe manually into your project from https://github.com/symfony/... . Then, make sure you use the same minimum constraints for the JS dependencies in package.json and install the deps with "yarn install". I'd suggest you to completely remove the node_modules/ directory first and install the fresh deps from scratch just in case. And finally, compile your assets with webpack, usually it's just "yarn watch" but depends on your project.
Also, after recompiling the assets, I'd recommend you to try in Chrome Incognito mode just in case the browser cached some JS files locally.
I hope this helps!
Cheers!


Hi, thanks for the help you are giving me! I have compared the symfony/webpack-encore-bundle files with my project and I can't find any differences. Then I compared the @babel folder in node_modules with a working project and there are differences. I tried to replace this folder with the one of the working project but the yarn watch and yarn build scripts no longer work! Luckily I had made a backup and put everything back in place. Can @babel be updated without breaking everything? Before making updates I would like to know how to make a backup, thank you very much for your patience
Hey Marco,
Hm, are you sure the constraints you have in your packages.json for deps are exactly the same as in the recipe? If you think that Babel version installed in your project is the problem - try to figure out what version is installed on working project, and install the exact same version in your project, you can specify the exact version of Babel in packages.json. I only see this way of solving then
Cheers!


hi Victor,
the problem is that in the package.json file of the two projects there is no babel, only @babel/preset-react is present but in node_modules I have @babel, babel-loader etc ... I think they have been installed as a dependency of some other package.
Cheers!
Hey Marco,
Yes, you're right, most probably that was installed as a dependency of another direct dependency that you have in your packages.json file.
Cheers!


Hi .... I'm going crazy! When I install a package with yarn I get these warnings:
warning "@symfony/webpack-encore> @babel/preset-env> @babel/plugin-bugfix-v8-spread-parameters-in-optional-chaining@ 7.14.5" has incorrect peer dependency "@babel/core @^7.13.0 ".
`<br />warning "@symfony/webpack-encore> @babel/preset-env> @babel/plugin-proposal-class-static-block @7.14.5" has incorrect peer dependency "@babel/core @^7.12.0".
I think they could be the cause of the problem!
Thank you
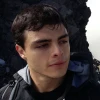
Hey Marco P.
Have you tried upgrading and clearing Yarn's cache? those warnings seems odd to me. I'd try deleting the node_modules
directory and reinstall it, in case nothing works
Cheers!


Hi,
I deleted the node_modules
directory and launched yarn run
... the errors remain. Fortunately, everything still seems to work! The problem is that since I updated webpack-encore-bundle
to make Stimulus work, after compiling with yarn build
I get all the .js files with the globalThis
variable as they were previously generated with self
. The application is run by several browsers and not all of them support globalThis
. Cheers!
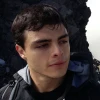
Ohh that makes sense. I think you're missing Babel's polyfill, try installing it in your project https://babeljs.io/docs/en/...
Cheers!


Hi Diego,
actually polifill was not installed, I ran: yarn add @babel/polyfill
...the error remains! I realized that the packages that give the error require a more up-to-date version of @babel/core
so I ran yarn add @babel/core
. Then I installed the packages that made the compilation fail: yarn add @babel/plugin-syntax-dynamic-import
, yarn add @babel/plugin-proposal-class-properties
, yarn add @babel/preset-env
. There are no more errors !!! ... but the GlobalThis
variable remained🙁
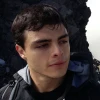
It seems like the GlobalThis is not part of Babel's polyfill. I found this library that may help you out, you can give it a try :)
https://www.npmjs.com/packa...
Cheers!


is in several files: runtime.js jsonp chunk loading, line 46 var chunkLoadingGlobal = globalThis ["webpackChunk"] = globalThis ["webpackChunk"] || []; but there are others
I have problems width encore instalation:
`/usr/bin/node /usr/local/lib/node_modules/yarn/bin/yarn.js install
yarn install v1.22.10
info No lockfile found.
warning package-lock.json found. Your project contains lock files generated by tools other than Yarn. It is advised not to mix package managers in order to avoid resolution inconsistencies caused by unsynchronized lock files. To clear this warning, remove package-lock.json.
[1/4] Resolving packages...
warning urix@0.1.0: Please see https://github.com/lydell/urix#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > urix@0.1.0: Please see https://github.com/lydell/urix#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve > urix@0.1.0: Please see https://github.com/lydell/urix#deprecated
warning @symfony/webpack-encore > resolve-url-loader > rework > css > source-map-resolve > resolve-url@0.2.1: https://github.com/lydell/resolve-url#deprecated
[2/4] Fetching packages...
error webpack-dev-server@4.0.0-beta.3: The engine "node" is incompatible with this module. Expected version ">= 12.13.0". Got "10.19.0"
error Found incompatible module.
info Visit https://yarnpkg.com/en/docs/cli/install for documentation about this command.
Process finished with exit code 1
`
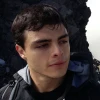
Hey Macarena I.
It seems like your Node version it's kind of old, try upgrading it to version 12.13 at least, and give it another shot.
Cheers!
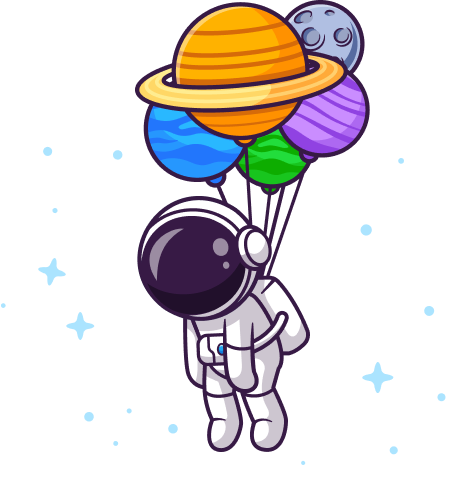
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=8.1",
"ext-ctype": "*",
"ext-iconv": "*",
"composer/package-versions-deprecated": "1.11.99.1", // 1.11.99.1
"doctrine/annotations": "^1.0", // 1.11.1
"doctrine/doctrine-bundle": "^2.2", // 2.2.3
"doctrine/doctrine-migrations-bundle": "^3.0", // 3.0.2
"doctrine/orm": "^2.8", // 2.8.1
"phpdocumentor/reflection-docblock": "^5.2", // 5.2.2
"sensio/framework-extra-bundle": "^5.6", // v5.6.1
"symfony/asset": "5.2.*", // v5.2.3
"symfony/console": "5.2.*", // v5.2.3
"symfony/dotenv": "5.2.*", // v5.2.3
"symfony/flex": "^1.3.1", // v1.21.6
"symfony/form": "5.2.*", // v5.2.3
"symfony/framework-bundle": "5.2.*", // v5.2.3
"symfony/property-access": "5.2.*", // v5.2.3
"symfony/property-info": "5.2.*", // v5.2.3
"symfony/proxy-manager-bridge": "5.2.*", // v5.2.3
"symfony/security-bundle": "5.2.*", // v5.2.3
"symfony/serializer": "5.2.*", // v5.2.3
"symfony/twig-bundle": "5.2.*", // v5.2.3
"symfony/ux-chartjs": "^1.1", // v1.2.0
"symfony/validator": "5.2.*", // v5.2.3
"symfony/webpack-encore-bundle": "^1.9", // v1.11.1
"symfony/yaml": "5.2.*", // v5.2.3
"twig/extra-bundle": "^2.12|^3.0", // v3.2.1
"twig/intl-extra": "^3.2", // v3.2.1
"twig/twig": "^2.12|^3.0" // v3.2.1
},
"require-dev": {
"doctrine/doctrine-fixtures-bundle": "^3.4", // 3.4.0
"symfony/debug-bundle": "^5.2", // v5.2.3
"symfony/maker-bundle": "^1.27", // v1.30.0
"symfony/monolog-bundle": "^3.0", // v3.6.0
"symfony/stopwatch": "^5.2", // v5.2.3
"symfony/var-dumper": "^5.2", // v5.2.3
"symfony/web-profiler-bundle": "^5.2" // v5.2.3
}
}
What JavaScript libraries does this tutorial use?
// package.json
{
"devDependencies": {
"@babel/preset-react": "^7.0.0", // 7.12.13
"@popperjs/core": "^2.9.1", // 2.9.1
"@symfony/stimulus-bridge": "^2.0.0", // 2.1.0
"@symfony/ux-chartjs": "file:vendor/symfony/ux-chartjs/Resources/assets", // 1.1.0
"@symfony/webpack-encore": "^1.0.0", // 1.0.4
"bootstrap": "^5.0.0-beta2", // 5.0.0-beta2
"core-js": "^3.0.0", // 3.8.3
"jquery": "^3.6.0", // 3.6.0
"react": "^17.0.1", // 17.0.1
"react-dom": "^17.0.1", // 17.0.1
"regenerator-runtime": "^0.13.2", // 0.13.7
"stimulus": "^2.0.0", // 2.0.0
"stimulus-autocomplete": "^2.0.1-phylor-6095f2a9", // 2.0.1-phylor-6095f2a9
"stimulus-use": "^0.24.0-1", // 0.24.0-1
"sweetalert2": "^10.13.0", // 10.14.0
"webpack-bundle-analyzer": "^4.4.0", // 4.4.0
"webpack-notifier": "^1.6.0" // 1.13.0
}
}
If you can't install
symfony/stimulus-bundle
because of unresolved dependencies use this composer.json instead:It's same but symfony version increased to 5.4.