Chapters
-
Course Code
Subscribe to download the code!
-
This Video
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Bundles!
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Hey friends! Welcome to Symfony 5 Fundamentals! I cannot emphasize enough, how important this course is to make you super productive in Symfony. And, well, I also think you're going to love it. Because we're going to really explore how your app works: services, configuration, environment, environment variables and more! These will be the tools that you'll need for everything else that you'll do in Symfony. After putting some work in now, anything else you build will feel much easier.
Ok! Let's go unlock some potential! The best way to do that - of course - is to download the course code from this page and code along with me. If you followed our first course, you rock! The code is basically where that course finished. But I recommend downloading the new code because I did make a few small tweaks.
After you unzip the download, you'll find a start/
directory with the same code that you see here. Head down to the fancy README.md
for all the instructions on how you get your project set up... and, of course, a poem about magic.
The last step in the setup will be to find a terminal, move into the project and use the symfony
executable to start a handy development web server. If you don't have this symfony
binary, you can download it at https://symfony.com/download. I'll run:
symfony serve -d
to start a web server at localhost:8000
. The -d
means run as a "daemon" - a fancy way of saying that this runs in the background and I can keep using my terminal. You can run symfony server:stop
later to stop it.
Ok! Spin over to your browser and go to https://localhost:8000 to see... Cauldron Overflow! Our question & answer site dedicated to Witches and Wizards. It's a totally untapped market.
Services do Everything
One of the things we learned at the end of the first course is that all the work in a Symfony app - like rendering a template, logging something, executing database queries, making API calls - everything is done by one of many useful objects floating around. We call these objects services. There's a router service, logger service, service for rendering Twig templates and many more. Simply put, a service is a fancy word for an object that does work.
And because services do work, they're tools! If you know how to get access to these objects, then you're very powerful. How do we access them? The primary way is by something called autowiring. Open up src/Controller/QuestionController.php
and find the homepage()
method:
Show Lines
|
// ... lines 1 - 9 |
class QuestionController extends AbstractController | |
{ | |
/** | |
* @Route("/", name="app_homepage") | |
*/ | |
public function homepage(Environment $twigEnvironment) | |
{ | |
/* | |
// fun example of using the Twig service directly! | |
$html = $twigEnvironment->render('question/homepage.html.twig'); | |
return new Response($html); | |
*/ | |
return $this->render('question/homepage.html.twig'); | |
} | |
Show Lines
|
// ... lines 26 - 42 |
} |
We commented out the code that used it, but by adding an argument type-hinted with Environment
, we signaled to Symfony that we wanted it to pass us the Twig service object:
Show Lines
|
// ... lines 1 - 7 |
use Twig\Environment; | |
class QuestionController extends AbstractController | |
{ | |
Show Lines
|
// ... lines 12 - 14 |
public function homepage(Environment $twigEnvironment) | |
{ | |
Show Lines
|
// ... lines 17 - 24 |
} | |
Show Lines
|
// ... lines 26 - 42 |
} |
That's called autowiring.
And how did we know to use this exact Environment
type hint to get the Twig service? And what other service objects are floating around waiting for us to use them and claim ultimate programming glory? Find your terminal and run:
php bin/console debug:autowiring
Boom! This is our guide. Near the bottom, it says that if you type-hint a controller argument with Twig\Environment
, it will give us the Twig service. Another one is CacheInterface
: use that type-hint to get a useful caching object. This is your menu of what service objects are available and what type-hint to use in a controller argument to get them.
Hello Bundles!
But where do these services come from? Like, who added these to the system? The answer to that is... bundles. Back in your editor, open a new file: config/bundles.php
:
Show Lines
|
// ... lines 1 - 2 |
return [ | |
Symfony\Bundle\FrameworkBundle\FrameworkBundle::class => ['all' => true], | |
Sensio\Bundle\FrameworkExtraBundle\SensioFrameworkExtraBundle::class => ['all' => true], | |
Symfony\Bundle\TwigBundle\TwigBundle::class => ['all' => true], | |
Twig\Extra\TwigExtraBundle\TwigExtraBundle::class => ['all' => true], | |
Symfony\Bundle\WebProfilerBundle\WebProfilerBundle::class => ['dev' => true, 'test' => true], | |
Symfony\Bundle\MonologBundle\MonologBundle::class => ['all' => true], | |
Symfony\Bundle\DebugBundle\DebugBundle::class => ['dev' => true, 'test' => true], | |
Symfony\WebpackEncoreBundle\WebpackEncoreBundle::class => ['all' => true], | |
]; |
We'll see who uses this file later, but it returns an array with 8 class names that all have the word "Bundle" in them.
Ok, first, whenever you install one of these "bundle" things, the Flex recipe system automatically updates this file for you and adds the new bundle. For example, in the first course, when we installed this WebpackEncoreBundle
, its recipe added this line:
Show Lines
|
// ... lines 1 - 2 |
return [ | |
Show Lines
|
// ... lines 4 - 10 |
Symfony\WebpackEncoreBundle\WebpackEncoreBundle::class => ['all' => true], | |
]; |
The point is, this is not a file that you normally need to think about.
But... what is a bundle? Very simply: bundles are Symfony plugins. They're PHP libraries with special integration with Symfony.
And, the main reason that you add a bundle to your app is because bundles give you services! In fact, every single service that you see in the debug:autowiring
list comes from one of these eight bundles. You can kind of guess that the Twig\Environment
service down here comes from TwigBundle
:
Show Lines
|
// ... lines 1 - 2 |
return [ | |
Show Lines
|
// ... lines 4 - 5 |
Symfony\Bundle\TwigBundle\TwigBundle::class => ['all' => true], | |
Show Lines
|
// ... lines 7 - 11 |
]; |
So if I removed that TwigBundle
line and ran the command again, the Twig service would be gone.
And yes, bundles can give you other things like routes, controllers, translations and more. But the main point of a bundle is that it gives you more services, more tools.
Need a new tool in your app to... talk to an API or parse Markdown into HTML? If you can find a bundle that does that, you get that tool for free.
In fact, let's do exactly that next.
56 Comments
Hey pavelvondrasek!
Thanks for sharing! It looks like newer Node versions have a problem with @babel/preset-env
at version 7.9 or lower (which is a dependency of @symfony/webpack-encore. So by upgrading Encore, you also upgraded @babel/preset-env... and fixed the issue :). I was able to repeat it locally.
We'll do an audit of our courses that use @babel/preset-env 7.9 or lower and get those upgraded so others don't hit the same issue.
Thanks for the report!
You are most welcome, Ryan. And by the way, I really love the course, keep up the good work :-) Thank you
Hi i have the same error. Please i dont understand how you fix it i try yarrn upgrad but its the same for me, nothing change
edit : its ok for me. My way step by step :
-open project and run composer install
-yarn install
-yarn upgrade
-yarn add @babel/core --dev
-yarn add webpack
-yarn encore dev --watch and its ok
Thanks for all !
Awesome! Glad it's working for you now and Thanks for posting the step by step!


Is there a way to see the subtitles in Spanish for this course?
Hello Francis G.
Sorry, but not yet. We are working to release more translations, but it's still pretty complex and time-eating work so can't say anything about eta on new translations.
PS as a little inside I can say that we are working on some translation feature that will help to make it faster
Cheers!


Ok so what am I supposed to do after downloading the code?
'symfony serve' does not work in directory with course code, same when pasted downloaded code into directory with first course's code
finally when 'composer install' in directory, two huge red rectangles appear in terminal saying:
In FileLoader.php line 173:
!!
!! The routing file "/home/.../cauldron_overflow/con
!! fig/routes/framework.yaml" contains unsupported keys for "when@dev": "_erro
!! rs". Expected one of: "resource", "type", "prefix", "path", "host", "scheme
!! s", "methods", "defaults", "requirements", "options", "condition", "control
!! ler", "name_prefix", "trailing_slash_on_root", "locale", "format", "utf8",
!! "exclude", "stateless" in /home/.../cauldron_overflo
!! w/config/routes/framework.yaml (which is loaded in resource "/home/.../cauldron_overflow/config/routes/framework.yaml").
!! In YamlFileLoader.php line 244:
!!
!! The routing file "/home/.../cauldron_overflow/con
!! fig/routes/framework.yaml" contains unsupported keys for "when@dev": "_erro
!! rs". Expected one of: "resource", "type", "prefix", "path", "host", "scheme
!! s", "methods", "defaults", "requirements", "options", "condition", "control
!! ler", "name_prefix", "trailing_slash_on_root", "locale", "format", "utf8",
!! "exclude", "stateless".
Hey Jan,
Take a look into README file of the downloaded code ;) There you will find some some more required instructions along with "composer install". Then, after all the required steps, try to run the website. If still no success - let us know!
Also, I just re-downloaded the course code and tried to execute "composer install" in the start/ directory - no errors for me. I'm on PHP 7.4.25, what version of PHP do you use?
Cheers!


Hey Victor,
I've read README file, the problem was it didn't work before 'composer install' and after that I got those reports.
I actually managed to solve that by replacing '_errors' in cauldron_overflow/config/routes/framework.yaml for 'path' and now the website is running, I just don't know why the website didn't have problems with this '_errors' thing in the last course when that was there.
I am using PHP 8.0.14 version.
Hey Jan,
Thank you for sharing your solution with others! Hm, not sure why, I wasn't able to reproduce this locally, probably you did some Symfony Flex recipe updates? Or Composer dependencies update? It might be caused by this. Anyway, I'm happy to hear you're unblocked now and can follow the course, well done!
Cheers!


Hi, after download the files and turn on server in terminal i got an error. Help ! :)
`
: require(C:\Symfony/vendor/autoload.php): Failed to open stream: No such file or directory in
on line
: Uncaught Error: Failed opening required
'C:\Symfony/vendor/autoload.php' (include_path='C:\xampp\php\PEAR') in
C:\Symfony\config\bootstrap.php:5
Stack trace:
#0 C:\Symfony\public\index.php(7): require()
#1 {main}
thrown in
on line
`
Hey @Piotr!
Ah, sorry about troubles! But, I know this error :). Try running:
composer install
And check out the README.md file at the root of the downloaded code (e.g. the "start/" directory): it contains all the commands you need to get things going. If you still have any issues, let us know!
Cheers!


It helps. Thank you weaverryan!


Hit there. I have just started this tutorial and I am getting the same error again as the end of the previous tutorial (Chap 17) which I could not find any solution. Please help.
<blockquote>yarn install</blockquote>
Result:PS C:\Users\Anonymous31\Documents\GitHub\Symfony_learnings\Fundamentals2> yarn install<br />yarn install v1.22.15<br />[1/4] Resolving packages...<br />[2/4] Fetching packages...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />error An unexpected error occurred: "https://registry.yarnpkg.com/@babel/core/-/core-7.13.1.tgz: tunneling socket could not be established, cause=getaddrinfo ENOTF<br />OUND 10809".<br />info If you think this is a bug, please open a bug report with the information provided in "C:\\Users\\Anonymous31\\Documents\\GitHub\\Symfony_learnings\\Fundament<br />als2\\yarn-error.log".<br />info Visit https://yarnpkg.com/en/docs/cli/install for documentation about this command.<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />info There appears to be trouble with your network connection. Retrying...<br />
Hey Mo31!
I just tried to reproduce this issue locally, downloaded the course code and "cd" into start/ directory where I were able to execute "composer install" and "yarn install" successfully. I have version of Yarn that is closed to yours I think, mine is v1.22.17 - so I don't think it might be related to Yarn version. Yeah, I agree with Vladimir, this definitely sounds like a VPN issue if you use it. But, I suppose you're using it for accessing SymfonyCasts resources, i.e. watch our videos, but it's not needed for Yarn at all. Could you turn off your VPN and tried to execute that "yarn install" command without VPN? You need to install the JS vendors somehow, and it's only one-time action. As soon as those vendors will be installed, i.e. when "yarn install" finished successfully - you will be able to turn on the VPN again to watch the videos further.
If even with turned off VPN you have the same issue - could you try to connect to a different VPN region (several regions) and execute that "yarn install" again? It might be so that some regions will allow you to install the vendors and the command will be executed successfully eventually.
Let me know if this helps!
Cheers!


After I run
composer install
it shows the following message. I did download the course code and tried with ON/OFF vpn, I got the same result with different servers.
`C:\Users\Anonymous\Documents\GitHub\Symfony_learnings\servicesConfig\start>composer install
[Composer\Exception\NoSslException]
The openssl extension is required for SSL/TLS protection but is not available. If you can not enable the openssl extension, you can disable this error, at you
r own risk, by setting the 'disable-tls' option to true.
install [--prefer-source] [--prefer-dist] [--prefer-install PREFER-INSTALL] [--dry-run] [--dev] [--no-suggest] [--no-dev] [--no-autoloader] [--no-scripts] [--no-pr
ogress] [--no-install] [-v|vv|vvv|--verbose] [-o|--optimize-autoloader] [-a|--classmap-authoritative] [--apcu-autoloader] [--apcu-autoloader-prefix APCU-AUTOLOADER
-PREFIX] [--ignore-platform-req IGNORE-PLATFORM-REQ] [--ignore-platform-reqs] [--] [<packages>]...
`
Hey Mo31,
Wait, let's deal with the "yarn install" error first. Or did you execute it successfully already?
Cheers!


Hi Victor,
I tried with an another computer, "composer install" worked fine, "yarn install" sends the same error with or without vpn.
Hey Mo31,
Hm, somethings isn't quite right with your Yarn setup I suppose. But good news that "composer install" worked! Unfortunately, can't help too much on this - it does not related to the course project code actually, it's more user-specific/connection-specific error.
Please, try to google that error on the internet - I think there should be a solution for "info There appears to be trouble with your network connection. Retrying...".
I hope this helps!
Cheers!


Okay, I will keep searching. Hopefully I will find a solution.
Thanks.
Cheers!


Hi Victor. I am gonna try it right away and feedback you.
Cheers!
Hi Timera M.
I saw your message related to yarn at another chapter, I guess you have internet issues, but anyways, try another terminal app
PS: BTW I see you are windows user, so I'd recommend to use WSL for development, you will have better and stable setup with it =)
Cheers!


Hi @sadikoff , thanks for your reply.
I am windows user. I tried with CMD, and I got the same error. I'll consider the WSL solution too. As I am in China, I use a VPN to connect. Can this fact be the reason of the problem.
Yep VPN can be a problem, try another VPN or... I even don't know what else I can suggest in this case.
Cheers!


okay. thanks.
Cheers!


Dear, i just start second tutorial... i copy files to folder, and make steps from Readme file.... i have error, and within web viewer i have no page visible...
Error message:
Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 255
!! PHP Fatal error: Uncaught Symfony\Component\Dotenv\Exception\PathException: Unable to read the "/home/krolgrz/SymfonyProjects/02_NextStep/.env" environment file. in /home/krolgrz/SymfonyProjects/02_NextStep/vendor/symfony/dotenv/Dotenv.php:505
!! Stack trace:
!! #0 /home/krolgrz/SymfonyProjects/02_NextStep/vendor/symfony/dotenv/Dotenv.php(60): Symfony\Component\Dotenv\Dotenv->doLoad()
!! #1 /home/krolgrz/SymfonyProjects/02_NextStep/vendor/symfony/dotenv/Dotenv.php(80): Symfony\Component\Dotenv\Dotenv->load()
!! #2 /home/krolgrz/SymfonyProjects/02_NextStep/config/bootstrap.php(17): Symfony\Component\Dotenv\Dotenv->loadEnv()
!! #3 /home/krolgrz/SymfonyProjects/02_NextStep/bin/console(30): require('...')
!! #4 {main}
!! thrown in /home/krolgrz/SymfonyProjects/02_NextStep/vendor/symfony/dotenv/Dotenv.php on line 505
!!
Script @auto-scripts was called via post-update-cmd
I work on Debian GNU 11.0, with PHP 8.0
What i did wrong? :) Please help
Best regards.
Hey Grzegorz K.!
Ah, that's no fun! Sorry about the issues! Ok, let's see what's going on.
The key part of the errors is here:
Unable to read the "/home/krolgrz/SymfonyProjects/02_NextStep/.env"
You should have a .env file at the root of your project... but for some reason you don't. I just downloaded the course code from this page, unzipped and checked out the start/ directory: that file IS there. Is it possible that you were moving some files around and left this .env file behind? All you need in there is this:
###> symfony/framework-bundle ###
APP_ENV=dev
APP_SECRET=c28f3d37eba278748f3c0427b313e86a
#TRUSTED_PROXIES=127.0.0.0/8,10.0.0.0/8,172.16.0.0/12,192.168.0.0/16
#TRUSTED_HOSTS='^(localhost|example\.com)$'
###< symfony/framework-bundle ###
But I'm worried that if this file is missing for some reason, some other files might be too. Let me know what you find out :).
Cheers!


Doubled :( sorry
Double message, double love? :)
Those are a lot of deprecations. Is it possible they are coming from third party libraries?


After installing the new code (the one written along the "Charming Development" tutorial works well!) and starting the app there is a php error:
Parse error: syntax error, unexpected '?' in ... \cauldron-overflow\public\index.php on line 15
I have upgraded my PHP version to 7.4.21 to fulfil the composer.json requirement and also re-installed composer after that to be on the safe side.
Hey Ralf B.!
Hmm, this is interesting! I downloaded the course code also - here is line 15 - the line causing the problem:
if ($trustedProxies = $_SERVER['TRUSTED_PROXIES'] ?? $_ENV['TRUSTED_PROXIES'] ?? false) {
So the "?" in the error seems to be the "??" - which is PHP's "null coalescing operator". That was introduced in PHP 7.0... which tells me - somehow - I think something is running your code using PHP 5.x. Can you double-check that the PHP that's being used is the correct 7.4.21 version? Also, if you haven't already, stop and restart the Symfony web server. It's definitely odd that you didn't have problems with the "Charming Development" tutorial - but you are here! That's a mystery. Btw, I tried the code locally just now with PHP 7.3, and I can get it to parse this file.
Let me know what you find out :).
Cheers!


Hi Ryan!
PHP version is 7.4.21. I double-checked everything, restarted the web server, rebooted the machine. Same error PHP message which for me looked like something being wrong before line 15.
In a next attempt I installed the code in a subdirectory of my C:\xampp\htdocs to find out what happens when using XAMPP apache web server and:
it WORKS, both with XAMPP and Symfony web server!
There is as well no problem when installed in other directories on drive C: whereas the error occured with the code installed on a second hard drive D:
Could this have been the reason - wrong hard drive?
Thanks so far and best regards,
Ralf
Hey Ralf B.
That's too odd. Perhaps you had an old PHP installation in the other hard drive? or perhaps some files didn't install correctly, hence, when you installed again it started working
I'm not sure what went wrong there :)
Cheers!


Composer install throws me a problem:Environment variable not found: "SENTRY_DSN".
Hvae no idea how to deal with it. Logger on site says:
` Uncaught PHP Exception Symfony\Component\DependencyInjection\Exception\EnvNotFoundException: "Environment variable not found: "SENTRY_DSN"." at D:\cauldron_overflow\vendor\symfony\dependency-injection\EnvVarProcessor.php line 171
{
"exception": {}
}`
Hey Jakub!
Do you get this error when you download the course code from this page and try to get things running? I just tried locally, and I can't repeat it :/.
The SENTRY_DSN env var is one that we introduce during this course (so it IS referenced in the "finish" code for the course, but not the start). We actually (eventually) put this SENTRY_DSN into the "secrets vault" - https://symfonycasts.com/sc... - which is what sets it as an env var.
Let me know which code you're working with, and I'm sure we can get to the bottom of this problem :).
Cheers!


Thank you! I had to make a mistake and copied bad folder. It works fine without any problems


Hello,
I have downloaded course code and followed readme.md instructions, but it seems like my css and js files are not working
Hey Aleksander!
Ah, that’s no fun! Let’s see if we can get things working :).
If you can, open your browser’s debugging tools then click on the network tab. Now refresh. You should see a bunch of red requests for requests to the css and js files failing. If you click those (or right click and open in a new tab), what is the error you see? Is it a 404 error? “Connection error”? Is the error a big symfony error page? Or a plain looking error page?
That should help us figure out what’s going on. Also, is there anything special about your setup (docker, etc)?
Cheers!
Hello,
I've got an error when executing composer install
:
`Installing dependencies from lock file (including require-dev)
Verifying lock file contents can be installed on current platform.
Package operations: 48 installs, 0 updates, 0 removals
- Installing symfony/flex (v1.12.2): Extracting archive
- Installing doctrine/lexer (1.2.1): Extracting archive
- Installing psr/container (1.0.0): Extracting archive
- Installing symfony/polyfill-php80 (v1.22.1): Extracting archive
- Installing symfony/polyfill-php73 (v1.22.1): Extracting archive
- Installing symfony/polyfill-mbstring (v1.22.1): Extracting archive
- Installing symfony/polyfill-intl-normalizer (v1.22.1): Extracting archive
- Installing symfony/polyfill-intl-idn (v1.22.1): Extracting archive
- Installing symfony/mime (v5.0.11): Extracting archive
- Installing symfony/http-foundation (v5.0.11): Extracting archive
- Installing psr/event-dispatcher (1.0.0): Extracting archive
- Installing symfony/event-dispatcher-contracts (v2.2.0): Extracting archive
- Installing symfony/event-dispatcher (v5.0.11): Extracting archive
- Installing symfony/var-dumper (v5.0.11): Extracting archive
- Installing psr/log (1.1.3): Extracting archive
- Installing symfony/error-handler (v5.0.11): Extracting archive
- Installing symfony/http-kernel (v5.0.11): Extracting archive
- Installing symfony/routing (v5.0.11): Extracting archive
- Installing symfony/finder (v5.0.11): Extracting archive
- Installing symfony/filesystem (v5.0.11): Extracting archive
- Installing symfony/service-contracts (v2.2.0): Extracting archive
- Installing symfony/dependency-injection (v5.0.11): Extracting archive
- Installing symfony/config (v5.0.11): Extracting archive
- Installing symfony/var-exporter (v5.0.11): Extracting archive
- Installing psr/cache (1.0.1): Extracting archive
- Installing symfony/cache-contracts (v2.2.0): Extracting archive
- Installing symfony/cache (v5.0.11): Extracting archive
- Installing symfony/framework-bundle (v5.0.11): Extracting archive
- Installing doctrine/annotations (1.12.1): Extracting archive
- Installing sensio/framework-extra-bundle (v5.5.4): Extracting archive
- Installing symfony/console (v5.0.11): Extracting archive
- Installing twig/twig (v3.3.0): Extracting archive
- Installing symfony/translation-contracts (v2.3.0): Extracting archive
- Installing symfony/twig-bridge (v5.0.11): Extracting archive
- Installing symfony/debug-bundle (v5.0.11): Extracting archive
- Installing symfony/dotenv (v5.0.11): Extracting archive
- Installing monolog/monolog (2.2.0): Extracting archive
- Installing symfony/monolog-bridge (v5.0.11): Extracting archive
- Installing symfony/monolog-bundle (v3.6.0): Extracting archive
- Installing symfony/twig-bundle (v5.0.11): Extracting archive
- Installing symfony/web-profiler-bundle (v5.0.11): Extracting archive
- Installing symfony/stopwatch (v5.0.11): Extracting archive
- Installing symfony/profiler-pack (v1.0.5): Extracting archive
- Installing twig/extra-bundle (v3.3.0): Extracting archive
- Installing symfony/twig-pack (v1.0.1): Extracting archive
- Installing symfony/asset (v5.0.11): Extracting archive
- Installing symfony/webpack-encore-bundle (v1.8.0): Extracting archive
- Installing symfony/yaml (v5.0.11): Extracting archive
Generating autoload files
42 packages you are using are looking for funding.
Use thecomposer fund
command to find out more!
Synchronizing package.json with PHP packages
Don't forget to run npm install --force or yarn install --force to refresh your JavaScript dependencies!
Run composer recipes at any time to see the status of your Symfony recipes.
Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 1
!!
!! // Clearing the cache for the dev environment with debug true
!!
!!
!! In FileLoader.php line 173:
!!
!! Class "1\CommentController" does not exist in C:\Users\Antonio\dev\php\symfony\code-symfony-fundamentals\start\config/routes../../src/Controller/ (which is being imported from "C:\Users\Antonio\dev\php\symfony\code-symfony-fundamentals\start\config/routes/annotati
!! ons.yaml"). Make sure annotations are installed and enabled.
!!
!!
!! In AnnotationDirectoryLoader.php line 62:
!!
!! Class "1\CommentController" does not exist
!!
!!
!! cache:clear [--no-warmup] [--no-optional-warmers] [-h|--help] [-q|--quiet] [-v|vv|vvv|--verbose] [-V|--version] [--ansi] [--no-ansi] [-n|--no-interaction] [-e|--env ENV] [--no-debug] [--] <command>
!!
!!
Script @auto-scripts was called via post-install-cmd
`
This is my environment:
`PHP 8.0.0 (cli) (built: Nov 24 2020 22:02:58) ( NTS Visual C++ 2019 x64 )
Copyright (c) The PHP Group
Zend Engine v4.0.0-dev, Copyright (c) Zend Technologies
with Zend OPcache v8.0.0, Copyright (c), by Zend Technologies
with Xdebug v3.0.1, Copyright (c) 2002-2020, by Derick Rethans
Symfony CLI version v4.23.2 (c) 2017-2021 Symfony SAS
Composer version 2.0.9 2021-01-27 16:09:27
`How can I fix this?
Also, PHPStorm is complaining that "symfony/profiler-pack" is both in "require" and in "require-dev". Is that meant to be so?
Thank you very much.
Hey @Antonio!
Just a quick update - the code download should now work fine with php 8 :).
Cheers!
Hey Antonio P.!
Hmm. You are now the 2nd person to report this, but I can't repeat it by downloading the course code! I see you're using PHP 8 - I'll need to check with that version - it could be an issue there. I recently upgraded some packages to allow for PHP 8 support. It looks like that wasn't fully completed correctly :). i'll update when i'm able to replicate and fix. Sorry about the trouble!
Cheers!


C:\xampp\htdocs\cauldron_overflow>./bin/console debug:autowiring
'.' is not recognized as an internal or external command,
operable program or batch file.
Hey,
could you try running it through PHP? php bin/console debug:autowiring
that's due to your OS
Cheers!


Running into issues during installation too.
My PHP version is 8.0.0 so the composer.json
wouldn't even let me proceed at first as it was fixed to 7. I changed the PHP requirement to "php": ">=7.2.5",
and that allowed Composer to install, but I keep running into this nasty error message:
<br />Executing script cache:clear [KO]<br /> [KO]<br />Script cache:clear returned with error code 1<br />!!<br />!! // Clearing the cache for the dev environment with debug<br />!! // true<br />!!<br />!!<br />!! In FileLoader.php line 173:<br />!!<br />!! Class "1\CommentController" does not exist in /Users/jack/Sites/cauldron_<br />!! overflow/config/routes/../../src/Controller/ (which is being imported from<br />!! "/Users/jack/Sites/cauldron_overflow/config/routes/annotations.yaml"). Ma<br />!! ke sure annotations are installed and enabled.<br />!!<br />!!<br />!! In AnnotationDirectoryLoader.php line 62:<br />!!<br />!! Class "1\CommentController" does not exist<br />!!<br />!!<br />
<b>ETA</b>: Sigh. I used brew to downgrade to PHP 7.4 and everything works fine. Heads-up for anyone using PHP 8!
Hey Jack,
Yeah, this course isn't ready for PHP 8 yet, that's why we show a little hint when you hover over the download course code link - it shows compatible PHP version.
For now, downgrading your PHP version to 7.x is the easiest solution to follow this course.
Cheers!


Hi there
i tried to install the application following the instructions on the readme.md file and i got this error when i run yarn install. any help ?
<blockquote>`
macbookpro@Leonardo cauldron_overflow % yarn install
yarn install v1.22.4
[1/4] 🔍 Resolving packages...
[2/4] 🚚 Fetching packages...
[3/4] 🔗 Linking dependencies...
warning " > bootstrap@4.4.1" has unmet peer dependency "popper.js@^1.16.0".
[4/4] 🔨 Building fresh packages...
[1/2] ⡀ fsevents
warning Error running install script for optional dependency: "/Users/macbookpro/cauldron_overflow/node_modules/fsevents: Command failed.
Exit code: 1
Command: node-gyp rebuild
Arguments:
Directory: /Users/macbookpro/cauldron_overflow/node_modules/fsevents
Output:
gyp info it worked if it ends with ok
gyp info using node-gyp@5.1.0
gyp info using node@14.15.0 | darwin | x64
gyp info find Python using Python version 2.7.16 found at \"/System/Library/Frameworks/Python.framework/Versions/2.7/Resources/Python.app/Contents/MacOS/Python\"
gyp WARN EACCES current user (\"macbookpro\") does not have permission to access the dev dir \"/Users/macbookpro/Library/Caches/node-gyp/14.15.0\"
gyp WARN EACCES attempting to reinstall using temporary dev dir \"/var/folders/f8/3wqfd1wd50d390h5sypm6b9m0000gn/T/.node-gyp\"
gyp info spawn /System/Library/Frameworks/Python.framework/Versions/2.7/Resources/Python.app/Contents/MacOS/Python
gyp info spawn args [
gyp info spawn args '/usr/local/lib/node_modules/npm/node_modules/node-gyp/gyp/gyp_main.py',
gyp info spawn args 'binding.gyp',
gyp info spawn args '-f',
gyp info spawn args 'make',
gyp info spawn args '-I',
gyp info spawn args '/Users/macbookpro/cauldron_overflow/node_modules/fsevents/build/config.gypi',
gyp info spawn args '-I',
gyp info spawn args '/usr/local/lib/node_modules/npm/node_modules/node-gyp/addon.gypi',
gyp info spawn args '-I',
gyp info spawn args '/var/folders/f8/3wqfd1wd50d390h5sypm6b9m0000gn/T/.node-gyp/14.15.0/include/node/common.gypi',
gyp info spawn args '-Dlibrary=shared_library',
gyp info spawn args '-Dvisibility=default',
gyp info spawn args '-Dnode_root_dir=/var/folders/f8/3wqfd1wd50d390h5sypm6b9m0000gn/T/.node-gyp/14.15.0',
gyp info spawn args '-Dnode_gyp_dir=/usr/local/lib/node_modules/npm/node_modules/node-gyp',
gyp info spawn args '-Dnode_lib_file=/var/folders/f8/3wqfd1wd50d390h5sypm6b9m0000gn/T/.node-gyp/14.15.0/<(target_arch)/node.lib',
gyp info spawn args '-Dmodule_root_dir=/Users/macbookpro/cauldron_overflow/node_modules/fsevents',
gyp info spawn args '-Dnode_engine=v8',
gyp info spawn args '--depth=.',
gyp info spawn args '--no-parallel',
gyp info spawn args '--generator-output',
gyp info spawn args 'build',
gyp info spawn args '-Goutput_dir=.'
gyp info spawn args ]
No receipt for 'com.apple.pkg.CLTools_Executables' found at '/'.
No receipt for 'com.apple.pkg.DeveloperToolsCLILeo' found at '/'.
No receipt for 'com.apple.pkg.DeveloperToolsCLI' found at '/'.
gyp: No Xcode or CLT version detected!
gyp ERR! configure error
gyp ERR! stack Error: gyp
failed with exit code: 1
gyp ERR! stack at ChildProcess.onCpExit (/usr/local/lib/node_modules/npm/node_modules/node-gyp/lib/configure.js:351:16)
gyp ERR! stack at ChildProcess.emit (events.js:315:20)
gyp ERR! stack at Process.ChildProcess._handle.onexit (internal/child_process.js:277:12)
gyp ERR! System Darwin 19.6.0
gyp ERR! command \"/usr/local/bin/node\" \"/usr/local/lib/node_modules/npm/node_modules/node-gyp/bin/node-gyp.js\" \"rebuild\"
gyp ERR! cwd /Users/macbookpro/cauldron_overflow/node_modules/fsevents
gyp ERR! node -v v14.15.0
gyp ERR! node-gyp -v v5.1.0
gyp ERR! not ok"
✨ Done in 23.98sI
`</blockquote>

It looks like it's related to xcode cli tools. Most likely it needs to be either installed or updated. This sometimes happens after an OS update.
No receipt for 'com.apple.pkg.CLTools_Executables' found at '/'.
Try:sudo rm -rf /Library/Developer/CommandLineTools
Then:xcode-select --install
Hey Karim B.
Wow, that error is new to me. Did the packages were installed at the end? Check if you have a node_modules folder with many other folders. I'd update Yarn and Node and then try again
Cheers!
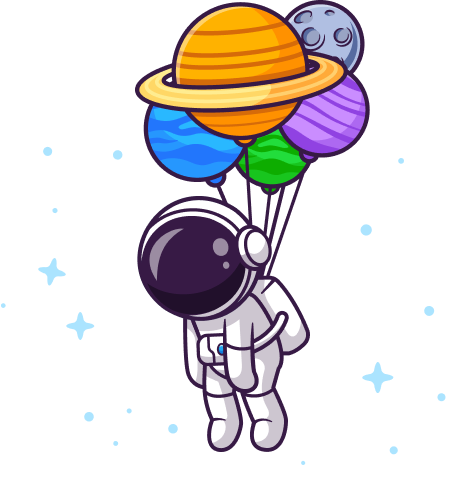
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.3.0 || ^8.0.0",
"ext-ctype": "*",
"ext-iconv": "*",
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"knplabs/knp-markdown-bundle": "^1.8", // 1.9.0
"sensio/framework-extra-bundle": "^6.0", // v6.2.1
"sentry/sentry-symfony": "^4.0", // 4.0.3
"symfony/asset": "5.0.*", // v5.0.11
"symfony/console": "5.0.*", // v5.0.11
"symfony/debug-bundle": "5.0.*", // v5.0.11
"symfony/dotenv": "5.0.*", // v5.0.11
"symfony/flex": "^1.3.1", // v1.21.6
"symfony/framework-bundle": "5.0.*", // v5.0.11
"symfony/monolog-bundle": "^3.0", // v3.6.0
"symfony/profiler-pack": "*", // v1.0.5
"symfony/routing": "5.1.*", // v5.1.11
"symfony/twig-pack": "^1.0", // v1.0.1
"symfony/var-dumper": "5.0.*", // v5.0.11
"symfony/webpack-encore-bundle": "^1.7", // v1.8.0
"symfony/yaml": "5.0.*" // v5.0.11
},
"require-dev": {
"symfony/maker-bundle": "^1.15", // v1.23.0
"symfony/profiler-pack": "^1.0" // v1.0.5
}
}
Hi, after running
yarn watch
which is the shortcut foryarn encore dev --watch
from the readme.md file, I bumped into issues:<br />webpack is watching the files…<br />ERROR Failed to compile with 1 errors<br />error in ./assets/js/app.js<br />Syntax Error: Error: Cannot find module <br />'./couldron_overflow/node_modules/@babel/compat-data/data/native-modules.json'<br />
Deleting the compat-data folder and running
yarn upgrade
can be helpful here (it did fixed the error for me). Cheers!