Chapters
-
Course Code
Subscribe to download the code!
Subscribe to download the code!
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Environment Variables
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
With a Subscription, click any sentence in the script to jump to that part of the video!
Login SubscribeOne big part of Symfony's configuration system that we have not talked about yet is: environment variables. To show them off, let's implement a real feature in our app.
Go to sentry.io
. If you've never used Sentry before, it's a cloud-based error monitoring tool: it's a really great way to track and debug errors on production. Not that those ever happen. Ahem. They also have excellent integration with Symfony.
If you don't already have an account, sign up - it's free. Once you do, you'll end up on a "Getting Started" page that looks something like this. I'll select Symfony from the list. Ok: it wants us to install some sentry/sentry-symfony
package.
Installing sentry/sentry-symfony & Contrib Recipes
Before you do, make sure you've committed all your changes to Git.
git add .
git commit -m "your commit message here..."
I committed before hitting record, so I'm good to go. I like to do this before installing a new package so I can see what its recipe does.
Back on the docs, copy the composer require
line, move over, and paste:
composer require sentry/sentry-symfony
It's downloading some packages and... interesting! It says:
The package for this recipe comes from the contrib repository, which is open to community contributions. Do you want to execute this recipe?
There are actually two places that recipes come from. The first is the main, official recipe repository, which is heavily-guarded for quality. Every recipe we've installed so far has been from that. The second is a "contrib" repository. That repository is less guarded for quality to make it easier for recipes from the community to be added, though the recipe still requires approval from a core Symfony member or an author of the package itself. The point is: if you're cautious, you can check a contrib recipe before you install it.
I'm going to say yes permanently by saying p
. Ok, what did the recipe do? Run:
git status
It modified the normal stuff - like composer.json
, composer.lock
, symfony.lock
and config/bundles.php
because this package contains a bundle: SentryBundle
:
Show Lines
|
// ... lines 1 - 2 |
return [ | |
Show Lines
|
// ... lines 4 - 12 |
Sentry\SentryBundle\SentryBundle::class => ['all' => true], | |
]; |
Hello Environment Variables & .env
The recipe also updated .env
and added a new config file. Let's go see what's going on.
First, open up .env
and scroll to the bottom. Woh! This has a new section that sets an environment variable called SENTRY_DSN
:
Show Lines
|
// ... lines 1 - 22 |
###> sentry/sentry-symfony ### | |
SENTRY_DSN= | |
###< sentry/sentry-symfony ### |
Environment variables are not a Symfony or PHP concept: they're values that you can pass to any process on your computer to configure that process's behavior. Symfony supports reading environment variables, which we'll see in a minute. But setting them can be a pain: it's different for every operating system. For that reason, when Symfony loads, it reads this file and sets anything here as an environment variable for you.
Reading Environment Variables with %env()%
So... if we're setting a SENTRY_DSN
environment variable... what's using that? Go into config/packages/
and open the shiny new sentry.yaml
file, which, not surprisingly, configures the new SentryBundle. Check this out: it has a dsn
key set to a very strange value: %env(SENTRY_DSN)%
:
sentry: | |
dsn: '%env(SENTRY_DSN)%' |
This... kind of looks like a parameter, right? It has percent signs on both sides, just like how, in cache.yaml
, we referenced the cache_adapter
parameter with %cache_adapter%
:
framework: | |
cache: | |
Show Lines
|
// ... lines 3 - 14 |
app: '%cache_adapter%' | |
Show Lines
|
// ... lines 16 - 20 |
And... it is sort of a parameter, but with a special super-power: when you surround something by %env()%
, it tells Symfony to read the SENTRY_DSN
environment value.
Why Environment Variables?
So... why are we setting an environment variable in .env
and then reading it here? Well, the SENTRY_DSN
string will be a sensitive value: if someone got access to it, they would be able to send information to our Sentry account.
Look back at the setup guide and skip down to the DSN part. Technically, we could copy this value and paste it right into sentry.yaml
. The problem is that this file will be committed to git... and it's generally a bad idea to commit sensitive values to your repository.
To avoid that, this bundle correctly recommended that we use an environment variable: we'll store the environment variable somewhere else, then read it here.
.env & .env.local
And, as we talked about, it is possible to set a real SENTRY_DSN
environment variable on your system. But... since that's a pain, Symfony allows us to instead define any environment variables we need in .env
if we want to... which we will.
But... this .env
file is also committed to the repository: you can see that in the terminal if you run:
git status
So if we pasted the SENTRY_DSN
value here, we would have the same problem: the sensitive value would be committed to the repository.
Here's the deal: the .env
file is meant to store non-sensitive default values for your environment variables - usually values that are good for local development. This works because after Symfony loads .env
, it looks for another file called .env.local
.
We don't have that yet, so let's create it: .env.local
.
Anything you put in this file will override the values in .env
. Let's add our real value here: SENTRY_DSN=
then paste:
SENTRY_DSN=https://7f45741877f3498eab0ae2bee6463d57@o372370.ingest.sentry.io/5186941 |
Perfect! In .env
, we set SENTRY_DSN
to a non-sensitive default:
Show Lines
|
// ... lines 1 - 22 |
###> sentry/sentry-symfony ### | |
SENTRY_DSN= | |
###< sentry/sentry-symfony ### |
in this case empty quotes means "don't send data to Sentry": and in .env.local
we override that to the real value:
SENTRY_DSN=https://7f45741877f3498eab0ae2bee6463d57@o372370.ingest.sentry.io/5186941 |
If you're confused why this is better, there's one more thing I need to tell you. Open up .gitignore
: the .env.local
file is ignored from Git:
Show Lines
|
// ... line 1 |
###> symfony/framework-bundle ### | |
/.env.local | |
Show Lines
|
// ... lines 4 - 18 |
Check it out: at your terminal, run:
git status
It does not see .env.local
: our sensitive value will not be committed. To see the final environment variable values, we can run:
php bin/console about
This gives us a bunch of info about our app including, at the bottom, a list of the environment variables being loaded from the .env
files. It's working perfectly.
Seeing it Work!
So let's... see if Sentry works! In the show()
controller, throw a very realistic new \Exception()
:
bad stuff happened!
Show Lines
|
// ... lines 1 - 11 |
class QuestionController extends AbstractController | |
{ | |
Show Lines
|
// ... lines 14 - 41 |
public function show($slug, MarkdownHelper $markdownHelper) | |
{ | |
if ($this->isDebug) { | |
$this->logger->info('We are in debug mode!'); | |
} | |
throw new \Exception('bad stuff happened!'); | |
Show Lines
|
// ... lines 48 - 62 |
} | |
} |
When we installed SentryBundle, it did add some services to the container. But the main purpose of those services isn't for us to interact with them directly: it's for them to hook into Symfony. The bundle's services are set up to listen for errors and send them to Sentry.
So all we need to do is... refresh! There's our error. Back on Sentry, I should be able to go to sentry.io
and... yep! It takes me over to the SymfonyCasts issues and we have a new entry: Exception: bad stuff happened!
Tip
If you don't see your logs showing up in Sentry, there could be some connection error that's being hidden from you. If you want to debug, check out Ryan's comment about this: https://bit.ly/sentry-debug
Next, how do you handle setting environment variables when you deploy? It's time to check out a cool new system called the secrets vault.
37 Comments
Hey @lhapaipai!
Thanks for posting your solution! This shouldn't (unless we're missing something) be needed if you're using the latest Sentry - 3.4. Did it install a lower version for you? Or are you using version 3.4?
Cheers!

Indeed, it seems that some dependencies may not be installed properly with plain composer require sentry/sentry-symfony
. For example, after executing it, I obtained the following dependency conflict:
`
Using version ^4.0 for sentry/sentry-symfony
...
Problem 1
- sentry/sentry-symfony[4.0.0, ..., 4.0.1] require symfony/config^3.4.43||^4.4.11||^5.0.11 -> found symfony/config[v3.4.43, ..., v3.4.47, v4.4.11, ..., v4.4.19, v5.0.11, ..., v5.2.3] but the package is fixed to v5.0.8 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
- Root composer.json requires sentry/sentry-symfony ^4.0 -> satisfiable by sentry/sentry-symfony[4.0.0, 4.0.1].
`
Fortunately, there is the nice option composer require sentry/sentry-symfony --with-all-dependencies
which automatically tries to resolve the conflict. However, this eventually ended up with
`
Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 255
!! Symfony\Component\ErrorHandler\Error\ClassNotFoundError {#5566
!! #message: """
!! Attempted to load class "Psr17Factory" from namespace "Nyholm\Psr7\Factory".\n
!! Did you forget a "use" statement for another namespace?
!! """
!! #code: 0
!! #file: "./var/cache/dev/ContainerGeyvHju/App_KernelDevDebugContainer.php"
!! #line: 1345
!! trace: {
!! ./var/cache/dev/ContainerGeyvHju/App_KernelDevDebugContainer.php:1345 {
!! ContainerGeyvHju\App_KernelDevDebugContainer->getHubInterfaceService()
!! ›
!! › $c = ($this->privates['nyholm.psr7.psr17_factory'] ?? ($this->privates['nyholm.psr7.psr17_factory'] = new \Nyholm\Psr7\Factory\Psr17Factory()));
!! ›
!! }
!! ./var/cache/dev/ContainerGeyvHju/App_KernelDevDebugContainer.php:1297 { …}
!! ./var/cache/dev/ContainerGeyvHju/App_KernelDevDebugContainer.php:623 { …}
!! ./vendor/symfony/event-dispatcher/EventDispatcher.php:245 { …}
!! ./vendor/symfony/event-dispatcher/EventDispatcher.php:76 { …}
!! ./vendor/symfony/event-dispatcher/Debug/TraceableEventDispatcher.php:290 { …}
!! ./vendor/symfony/event-dispatcher/Debug/TraceableEventDispatcher.php:145 { …}
!! ./vendor/symfony/console/Application.php:926 { …}
!! ./vendor/symfony/framework-bundle/Console/Application.php:96 { …}
!! ./vendor/symfony/console/Application.php:264 { …}
!! ./vendor/symfony/framework-bundle/Console/Application.php:82 { …}
!! ./vendor/symfony/console/Application.php:140 { …}
!! ./bin/console:42 { …}
!! }
!! }
!! 2021-02-09T12:57:08+00:00 [critical] Uncaught Error: Class 'Nyholm\Psr7\Factory\Psr17Factory' not found
!!
`
which seems to suggest that composer require nyholm/psr7
might be a good idea here, for some reason that I do not understand...
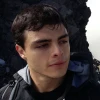
Hey pavelvondrasek
Thanks for your input. When you update all of the dependencies of the sentry/sentry-symfony
library, one of its dependencies requires nyholm/psr7
but for some reason you have to install it manually. Installing that library does not cause any problems, you can do it and keep going with this course, or, you can just install sentry/sentry-symfony
3.4.4 version, that's the version which is compatible with the course code
Cheers!


bin/console about did not show the env variables. I got it through bin/console debug:container --env-vars.


The ./bin/console debug:dotenv command worked best for me.
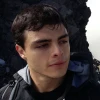
Hey Joao P.
That's odd. What's the out put of the command?
What version Symfony are you using?


$ bin/console about
-------------------- ---------------------------------
Symfony
-------------------- ---------------------------------
Version 5.1.2
Long-Term Support No
End of maintenance 01/2021
End of life 01/2021
-------------------- ---------------------------------
Kernel
-------------------- ---------------------------------
Type App\Kernel
Environment dev
Debug true
Charset UTF-8
Cache directory ./var/cache/dev (1.8 MiB)
Log directory ./var/log (142 KiB)
-------------------- ---------------------------------
PHP
-------------------- ---------------------------------
Version 7.4.8
Architecture 64 bits
Intl locale n/a
Timezone UTC (2020-07-28T15:26:51+00:00)
OPcache false
APCu true
Xdebug false
-------------------- ---------------------------------
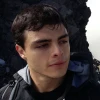
Ha! You made me dig. They removed the env var section since Symfony 5.1. You can read the details here https://github.com/symfony/...
They recommend to do what you did in case you want to see a specific env var. Cheers!

I spent some time trying to figure out why I didn't get a sentry.yaml, in my config/packages. Tried creating one, but that didn't work. Doesn't look like I need one as I was able to get the 'bad stuff happens' error without it. Not too sure why though.
The configure Symfony SDK page was even telling me to add:
when@prod:
sentry:
dsn: '%env(SENTRY_DSN)%'
# Specify a fixed sample rate
traces_sample_rate: 1.0
# Set a sampling rate for profiling - this is relative to traces_sample_rate
profiles_sample_rate: 1.0
but none of that seemed to be necessary so far. Not even the pecl install excimer
Hey Didi,
When you install a package in Symfony app using Composer - it executes a special plugging called Symfony Flex that executes some recipes, that's why some extra files may pop into your project. Usually, Symfony Flex will ask you if you want to execute the recipe, but if you reply "No" - the recipe won't be executed and so no config files are created. Probably you reply no during the composer require
? Or it also might be configured in your composer.json globally to ignore installing such recipes. Those are the most common problems if the recipe exists in symfony/recipes or symfony/recipes-contib but isn't executed. the solution might be to create the necessary config files manually or remove the package and install it again, allowing its recipe execution in the CLI. Also, some errors that happen during the composer require
command also may cause problems with further recipe execution.
I hope this clarifies things!
Cheers!

I replyed y to composer require; But it never created the file sentry.yaml withsentry:<br /> dsn: '%env(resolve:SENTRY_DSN)%'
Whenever I try to create it myself I get: There is no extension able to load the configuration for "sentry"
Without the sentry.yaml file I get through this video just fine and get the error in sentry.io
But the next video when I try to use HubInterface from Sentry I get:
Cannot autowire argument $sentryHub of "App\Controller\QuestionController::show()": it references interface "Sentry\State\HubInterface" but no such service exists. Did you create a class that implements this interface?
I tried to remove sentry and reinstall it. I never get any errors in the install, and is says: Using version ^4.13 for sentry/sentry-symfony.
I'm on a Mac, not sure if that could be an issue and I has a flag when following the steps from Sentry.io configure documentation.
Currently stuck unnable to move foward with the tutorial
Hey Didi,
What exactly version of sentry/sentry-symfony
did you install? The composer require sentry/sentry-symfony
finished well without any errors?
The latest version should execute this recipe: https://github.com/symfony/recipes-contrib/blob/main/sentry/sentry-symfony/4.6 - which means it should create the packages/sentry.yaml config file with this content: https://github.com/symfony/recipes-contrib/blob/main/sentry/sentry-symfony/4.6/config/packages/sentry.yaml
If it was not created automatically, please check that you don't have any config that deny executing contrib recipes in your composer.json.
Whenever I try to create it myself I get: There is no extension able to load the configuration for "sentry"
Seems the bundle was not registered automatically, check your bundles.php file, you may need to manually register the bundle there. Also, just in case, try to clear the cache, it may help sometimes.
But the next video when I try to use HubInterface from Sentry I get:
That makes sense if the bundle isn't registered, you don't have access to the services from that bundle. You need to register it first.
Cheers!


Hello,
I can't install "composer require maker --dev". It tells me :
"Your requirements could not be resolved to an installable set of packages."
Problem 1
- Root composer.json requires symfony/maker-bundle ^1.44 -> satisfiable by symfony/maker-bundle[v1.44.0].
- symfony/maker-bundle v1.44.0 requires symfony/config ^5.4.7|^6.0 -> found symfony/config[v5.4.7, v5.4.8, v5.4.9, v6.0.0, ..., v6.1.0] but the package is fixed to v5.0.11 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
Use the option --with-all-dependencies (-W) to allow upgrades, downgrades and removals for packages currently locked to specific versions.
You can also try re-running composer require with an explicit version constraint, e.g. "composer require symfony/maker-bundle:*" to figure out if any version is installable, or "composer require symfony/maker-bundle:^2.1" if you know which you need.
"Installation failed, reverting ./composer.json and ./composer.lock to their original content."
--------------------
I check my composer.json with the folder "finish" of the course. I don't see any difference (except maker..).
I tried to change, in composer.lock, the version of symfony/config manually (with "version" : "v5.4.9") but it engenders the same errors -> always fixed to v5.0.11 (even if I run : composer update & clear the cache)
What can I do?
Thanks!
In fact I think it's not really free you have 14 days of trial, and then you should pay something!
Is it a 14 day trial now? It still looks like they have a free "Developer Plan". Do you see that as an option?
Cheers!


Hello every one,
following this tutorial I have an issue as soon as i tried the composer require sentry :/ , here is what i got :
<spoiler><blockquote>`Installation failed, reverting ./composer.json to its original content.
[ErrorException]
Undefined index: extra
`</blockquote></spoiler>
if it help here is my composer.json :
<spoiler><blockquote>
`
{
"type": "project",
"license": "proprietary",
"require": {
"php": "^7.2.5",
"ext-ctype": "*",
"ext-iconv": "*",
"knplabs/knp-markdown-bundle": "^1.8",
"sensio/framework-extra-bundle": "^5.5",
"symfony/asset": "5.0.*",
"symfony/console": "5.0.*",
"symfony/debug-bundle": "5.0.*",
"symfony/dotenv": "5.0.*",
"symfony/flex": "^1.3.1",
"symfony/framework-bundle": "5.0.*",
"symfony/monolog-bundle": "^3.0",
"symfony/profiler-pack": "*",
"symfony/twig-pack": "^1.0",
"symfony/var-dumper": "5.0.*",
"symfony/webpack-encore-bundle": "^1.7",
"symfony/yaml": "5.0.*"
},
"require-dev": {
"symfony/profiler-pack": "^1.0"
},
"config": {
"preferred-install": {
"*": "dist"
},
"sort-packages": true,
"platform": {}
},
"autoload": {
"psr-4": {
"App\\": "src/"
}
},
"autoload-dev": {
"psr-4": {
"App\\Tests\\": "tests/"
}
},
"replace": {
"paragonie/random_compat": "2.*",
"symfony/polyfill-ctype": "*",
"symfony/polyfill-iconv": "*",
"symfony/polyfill-php72": "*",
"symfony/polyfill-php71": "*",
"symfony/polyfill-php70": "*",
"symfony/polyfill-php56": "*"
},
"scripts": {
"auto-scripts": {
"cache:clear": "symfony-cmd",
"assets:install %PUBLIC_DIR%": "symfony-cmd"
},
"post-install-cmd": [
"@auto-scripts"
],
"post-update-cmd": [
"@auto-scripts"
]
},
"conflict": {
"symfony/symfony": "*"
},
"extra": {
"symfony": {
"allow-contrib": false,
"require": "5.0.*"
}
}
}
`
</blockquote></spoiler>
also, i'm no longer able to make "composer update" too, i manage to update it with a composer self-update, but this did not solve my issue, (after a quick search, it seems thats some folks are having the same on a drupal bundle named zaporylie)
does someone have any clues to find a way out of this issue?


I solved it temporarly by editing a vendor file, thing that i think is not recommandable at all :/ so i'll keep an eye on your answer, if some one need the way to procede to the require sentry here is my modification :
Go to [vendor\symfony\flex\src\cache.php] at line 92, then change [
<br /> if('dev-master' === $ version))<br />
to
<br />if('dev-master' === $ version && isset($composerJson['extra']))<br />
as said here : <a href="https://github.com/composer/composer/issues/8047">link to github issue<a>
Hey Yacine R.
Yep that's not recommended at all =) The current solution is update flex library to latest version. You can do it with command:composer update symfony/flex --no-plugins --no-scripts
Cheers!


thank you, it worked out perfectly sadikoff
When I try to install the Sentry package, I get this error:
`
Executing script security-checker security:check [KO]
[KO]
Script security-checker security:check returned with error code 1
!!
!! In ErrorChunk.php line 65:
!!
!! fopen(https://52.49.93.188/check_lock): failed to open stream: Failed to pa
!! rse address "0"
!!
!!
!! In NativeResponse.php line 115:
!!
!! fopen(https://52.49.93.188/check_lock): failed to open stream: Failed to pa
!! rse address "0"
!!
!!
!! security:check [--format FORMAT] [--end-point END-POINT] [--timeout TIMEOUT] [--token TOKEN] [--] [<lockfile>]
!!
!!
Script @auto-scripts was called via post-update-cmd
Installation failed, reverting ./composer.json to its original content.
`

I also had a security checker error when I tried to install sentry. It turned out that in between installing Symfony in the first place and trying to install Sentry, there was a patch for a security flaw in Symfony released. I think Composer (by default) won't let you do much until you update to patch the vulnerability. All I needed to do was composer update
to update the Symfony components before I installed Sentry.
I didn't get quite the same error as you (though it does say Script security-checker security:check returned with error code 1
) but it's similar, so I thought I'd mention it in case it helped anyone else.
Hey Mohammed
Can you try it again? This error looks not related to project code, probably some internal security checker connection error. Which shouldn't be permanent
Cheers!


Where I run: composer require sentry/sentry-symfony - I get an error: "Problem 1 - The requested PHP extension ext-http * is missing from your system. Install or enable PHP's http extension."
I tried searching for multiple ways to fix this, but nothing I found has worked. I don't know if I have messed up my project previously and something is missing or I have been too long with too little sleep. The top of my composer.json file is below.
{
"type": "project",
"license": "proprietary",
"require": {
"php": ">=7.2.5",
"ext-ctype": "*",
"ext-iconv": "*",
"airmoi/filemaker": "*",
"composer/package-versions-deprecated": "^1.11",
"doctrine/doctrine-bundle": "^2.1",
"doctrine/doctrine-migrations-bundle": "^3.0",
"doctrine/orm": "^2.7",
"sensio/framework-extra-bundle": "^5.5",
"symfony/asset": "5.1.*",
"symfony/console": "5.1.*",
"symfony/debug-bundle": "5.1.*",
"symfony/dotenv": "5.1.*",
"symfony/flex": "^1.3.1",
"symfony/framework-bundle": "5.1.*",
"symfony/monolog-bundle": "^3.0",
"symfony/stopwatch": "5.1.*",
"symfony/twig-bundle": "5.1.*",
"symfony/var-dumper": "5.1.*",
"symfony/webpack-encore-bundle": "^1.7",
"symfony/yaml": "5.1.*",
"twig/extra-bundle": "^2.12|^3.0",
"twig/twig": "^2.12|^3.0",
"ext-curl": "*",
"ext-http": "*"
},
"require-dev": {
"sensiolabs/security-checker": "^6.0",
"symfony/stopwatch": "^5.1",
"symfony/twig-bundle": "^5.1",
"symfony/web-profiler-bundle": "^5.1"
},
"config": {
"optimize-autoloader": true,
"preferred-install": {
"*": "dist"
},
"sort-packages": true
},
"autoload": {
"psr-4": {
"App\\": "src/"
}
},
"autoload-dev": {
"psr-4": {
"App\\Tests\\": "tests/"
}
},
"replace": {
"paragonie/random_compat": "2.*",
"symfony/polyfill-ctype": "*",
"symfony/polyfill-iconv": "*",
"symfony/polyfill-php72": "*",
"symfony/polyfill-php71": "*",
"symfony/polyfill-php70": "*",
"symfony/polyfill-php56": "*"
},
"scripts": {
"auto-scripts": {
"cache:clear": "symfony-cmd",
"assets:install %PUBLIC_DIR%": "symfony-cmd",
"security-checker security:check": "script"
},
"post-install-cmd": [
"@auto-scripts"
],
"post-update-cmd": [
"@auto-scripts"
]
},
"conflict": {
"symfony/symfony": "*"
},
"extra": {
"symfony": {
"allow-contrib": false,
"require": "5.1.*"
}
}
}
Hey Wade,
Did you write that "ext-http": "*"
in your composer.json? :) If you did it mistakenly - just remove this line and run the "composer require sentry/sentry-symfony" again - it should work without errors. But if you added that line on purpose, that makes sense that Composer fails as it requires that "http" extension to work properly.
I hope this helps!
Cheers!


Hi Victor, I didn't write it, but there was something PHP Storm flagged as needing it, so I clicked into the message where PHP Storm recommended to add it and it was added programmatically. There were several ext- items there that came form the same warning inside PHP Storm.
Hey Wade,
Ah, I see... Probably PhpStorm also suggests you what PHP extension you have to install? :) Well, first of all you can run Symfony requirements checker and it should say you what extensions you don't have that is required by Symfony. Also, it depends on the code of your project, some extensions might be needed for *your* code. Clearly, it says that you have to install "http" PHP extension. About how to do it - depends on your OS, probably better to good this for the OS you use.
Otherwise, you can try to remove it from your composer.json and figure out what code exactly requires this extension to be able to test if it still works for your system or you really need to install an extra extension.
I hope this helps!
Cheers!


I also cannot get the exceptions to send to Sentry. I cleared the cache and double-checked the credentials.
The errors I see in the console seem to all be related to the exception I am throwing. Is there a way to verify that the bundle is sending out the error to Sentry?
Hey i-man!
Hmm. Now 2 people have had an issue - let's see if we can figure this out :). The best way to check your config is to directly dump the Sentry "Hub" object - check out what we do in the next chapter - https://symfonycasts.com/screencast/symfony-fundamentals/secrets-vault#dumping-the-sentry-connection-details - that will tell you exactly what config Sentry is seeing. You should be able to see if it's misconfigured. If you see a NullTransport instead of an HttpTransport, then (for some reason) your SENTRY_DSN is set to an empty string (which disables sentry).
Unfortunately, Sentry can be a bit hard to debug because, if there is any error sending info to Sentry, Sentry hides the error. It does this for good reason: if Sentry fails for some reason on production, it's probably better for it to not take the whole site down. But it can make things tricky. If you think that your config is correct but you still don't see anything, you could also open this core class and add a dd($exception)
right before this return null: https://github.com/getsentry/sentry-php/blob/master/src/Transport/HttpTransport.php#L141 - but to get this to work, you will also need to set $this->delaySendingUntilShutdown = false;
right before this line: https://github.com/getsentry/sentry-php/blob/b2cfb8b9e524727db63592fd5183e7a48058bb73/src/Transport/HttpTransport.php#L127
If you do those 2 things, and there's a failure, you should see it. Unfortunately, while Sentry itself has support for logging (you can see logger code in there), the bundle doesn't integrate with Symfony well enough to make that a real logger - it is a NullLogger... which means it logs nowhere.
Let me know if that helps! I'm happy to keep debugging with you :).
Cheers!


I have been trying to figure this out for a while now. You share two files one from the master branch and one from a obscure branch. Both with the same name and location in the folder structure. The first file i should edit add line 140 when the file only has 136 lines ... ? And the second file does not exist in my workflow.
Sad that I can no longer continue on with the coarse as the use of some external tool is not working.
Hey ZoeF,
Sorry about that, Ryan forgot to put the commit hash in the link and the file was modified since his comment. I bet Ryan was talking about this return statement: https://github.com/getsentr...
I hope this helps!
As always, if you have any problems following the tutorial - let us know in the comments and we will help!
Cheers!


Thanks so much, that worked perfectly!
Once I was able to see the error the sentry code was throwing, it clearly pointed out that I had a cURL error. Specifically, it was a certificate problem with my PHP server. Once I added the cacert per the instructions here: https://www.mynotepaper.com... everything started working as expected and my error showed up in Sentry.
Thanks for the help!


I had the same problem and this solution worked for we as well. I suggest putting a note on the video, i-man. Thanks
Woo! Great job getting into that debugging :). That hidden error is *super* annoying. I get why they do it, but boy does that make things tricky!
Keep up the good work!
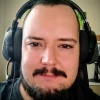
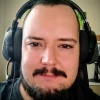
Hello, the Sentry is not working for me - I've done everything as you did in the video but my Sentry issues page is empty.
Hey MichaĆ,
Hm, do you have any errors? Please, double check your logs. Also, I'd recommend you to clear the cache and try again. And make sure that your Sentry credentials are matched the ones for your Sentry account.
Cheers!
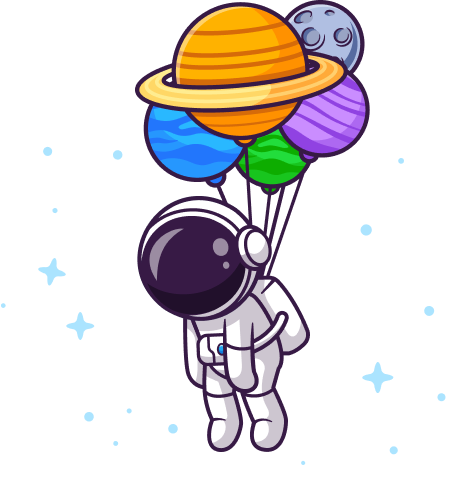
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.3.0 || ^8.0.0",
"ext-ctype": "*",
"ext-iconv": "*",
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"knplabs/knp-markdown-bundle": "^1.8", // 1.9.0
"sensio/framework-extra-bundle": "^6.0", // v6.2.1
"sentry/sentry-symfony": "^4.0", // 4.0.3
"symfony/asset": "5.0.*", // v5.0.11
"symfony/console": "5.0.*", // v5.0.11
"symfony/debug-bundle": "5.0.*", // v5.0.11
"symfony/dotenv": "5.0.*", // v5.0.11
"symfony/flex": "^1.3.1", // v1.21.6
"symfony/framework-bundle": "5.0.*", // v5.0.11
"symfony/monolog-bundle": "^3.0", // v3.6.0
"symfony/profiler-pack": "*", // v1.0.5
"symfony/routing": "5.1.*", // v5.1.11
"symfony/twig-pack": "^1.0", // v1.0.1
"symfony/var-dumper": "5.0.*", // v5.0.11
"symfony/webpack-encore-bundle": "^1.7", // v1.8.0
"symfony/yaml": "5.0.*" // v5.0.11
},
"require-dev": {
"symfony/maker-bundle": "^1.15", // v1.23.0
"symfony/profiler-pack": "^1.0" // v1.0.5
}
}
it works for me with this :
and