Chapters
-
Course Code
Compatible PHP versions: ^7.1.3
Compatible PHP versions: ^7.1.3
- This Video
- Subtitles
- Course Script
Hello Webpack Encore
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Yo friends! It's Webpack time! Yeeeeeeah! Well, maybe not super "yeeeeeeah!" if you are the person responsible for installing and configuring Webpack... Cause, woh, yea, that can be tough! Unless... you're using Webpack Encore! More about that in a few minutes.
Why all the Webpack Buzz?
But first, I want you to know why you should care about Webpack... like super please-let-me-start-using-webpack-right-now-and-never-stop-using-it kind of care. Sure, technically, Webpack is just a tool to build & compile your JavaScript and CSS files. But, it will revolutionize the way you write JavaScript.
The reason is right on their homepage! In PHP, we organize our code into small files that work together. But then, traditionally, in JavaScript, we just smash all our code into one gigantic file. Or, if we do split them up, it's still a pain because we then need to remember to go add a new script
tag to every page that needs it... and those script tags need to be in just the right order. If they're not, kaboom! And even if you do have a build system like Gulp, you still need to manage keeping all of the files listed there and in the right order. How can our code be so nicely organized in PHP, but such a disaster in JavaScript?
Webpack changes this. Suppose we have an index.js
file but we want to organize a function into a different, bar.js
file. Thanks to Webpack, you can "export" that function as a value from bar.js
and then import and use it in index.js
. Yes, we can organize our code into small pieces! Webpack's job is to read index.js
, parse through all of the import
statements it finds, and output one JavaScript file that has everything inside of it. Webpack is a huge over-achiever.
So let's get to it! To import or... Webpack the maximum amount of knowledge from this tutorial, download the course code from this page and code along with me. After you unzip the file, you'll find a start/
directory that has the same code I have here: a Symfony 4 app. Open up the README.md
file for all the setup details.
The last step will be to open a terminal, move into the project and start a web server. I'm going to use the Symfony local web server, which you can get from https://symfony.com/download. Run it with:
symfony serve
Then, swing back over to your browser and open up https://localhost:8000 to see... The Space Bar! An app we've been working on throughout our Symfony series. And, we did write some JavaScript and CSS in that series... but we kept it super traditional: the JavaScript is pretty boring, and there are multiple files but each has its own script
tag in my templates.
This is not the way I really code. So, let's do this correctly.
Installing WebpackEncoreBundle + Recipe
So even though both Webpack and Encore are Node libraries, if you're using Symfony, you'll install Encore via composer... well... sort of. Open a new terminal tab and run:
composer require "encore:^1.8"
This downloads a small bundle called WebpackEncoreBundle. Actually, Encore itself can be used with any framework or any language! But, it works super well with Symfony, and this thin bundle is part of the reason.
This bundle also has a Flex recipe - oooooOOOOooo - which gives us some files to get started! If you want to use Webpack from outside of a Symfony app, you would just need these files in your app.
Back in the editor, check out package.json
:
{ | |
"devDependencies": { | |
"@symfony/webpack-encore": "^0.27.0", | |
"core-js": "^3.0.0", | |
"webpack-notifier": "^1.6.0" | |
}, | |
"license": "UNLICENSED", | |
"private": true, | |
"scripts": { | |
"dev-server": "encore dev-server", | |
"dev": "encore dev", | |
"watch": "encore dev --watch", | |
"build": "encore production --progress" | |
} | |
} |
This is the composer.json
file of the Node world. It requires Encore itself plus two optional packages that we'll use:
{ | |
"devDependencies": { | |
"@symfony/webpack-encore": "^0.27.0", | |
"core-js": "^3.0.0", | |
"webpack-notifier": "^1.6.0" | |
}, | |
Show Lines
|
// ... lines 7 - 14 |
} |
Installing Encore via Yarn
To actually download these, go back to your terminal and run:
yarn
Or... yarn install
if you're less lazy than me - it's the same thing. Node has two package managers - "Yarn" and "npm" - I know, kinda weird - but you can install and use whichever you want. Anyways, this is downloading our 3 libraries and their dependencies into Node's version of the vendor/
directory: node_modules/
.
And... done! Congrats! You now have a gigantic node_modules/
directory... because JavaScript has tons of dependencies. Oh, the recipe also updated our .gitignore
file to ignore node_modules/
:
Show Lines
|
// ... lines 1 - 22 |
###> symfony/webpack-encore-bundle ### | |
/node_modules/ | |
/public/build/ | |
Show Lines
|
// ... lines 26 - 27 |
###< symfony/webpack-encore-bundle ### | |
Show Lines
|
// ... lines 29 - 31 |
Just like with Composer, there is no reason to commit this stuff. This also ignores public/build/
, which is where Webpack will put our final, built files.
Hello webpack.config.js
In fact, I'll show you why. At the root of your app, the recipe added the most important file of all webpack.config.js
:
var Encore = require('@symfony/webpack-encore'); | |
Encore | |
// directory where compiled assets will be stored | |
.setOutputPath('public/build/') | |
// public path used by the web server to access the output path | |
.setPublicPath('/build') | |
// only needed for CDN's or sub-directory deploy | |
//.setManifestKeyPrefix('build/') | |
/* | |
* ENTRY CONFIG | |
* | |
* Add 1 entry for each "page" of your app | |
* (including one that's included on every page - e.g. "app") | |
* | |
* Each entry will result in one JavaScript file (e.g. app.js) | |
* and one CSS file (e.g. app.css) if you JavaScript imports CSS. | |
*/ | |
.addEntry('app', './assets/js/app.js') | |
//.addEntry('page1', './assets/js/page1.js') | |
//.addEntry('page2', './assets/js/page2.js') | |
// When enabled, Webpack "splits" your files into smaller pieces for greater optimization. | |
.splitEntryChunks() | |
// will require an extra script tag for runtime.js | |
// but, you probably want this, unless you're building a single-page app | |
.enableSingleRuntimeChunk() | |
/* | |
* FEATURE CONFIG | |
* | |
* Enable & configure other features below. For a full | |
* list of features, see: | |
* https://symfony.com/doc/current/frontend.html#adding-more-features | |
*/ | |
.cleanupOutputBeforeBuild() | |
.enableBuildNotifications() | |
.enableSourceMaps(!Encore.isProduction()) | |
// enables hashed filenames (e.g. app.abc123.css) | |
.enableVersioning(Encore.isProduction()) | |
// enables @babel/preset-env polyfills | |
.configureBabel(() => {}, { | |
useBuiltIns: 'usage', | |
corejs: 3 | |
}) | |
// enables Sass/SCSS support | |
//.enableSassLoader() | |
// uncomment if you use TypeScript | |
//.enableTypeScriptLoader() | |
// uncomment to get integrity="..." attributes on your script & link tags | |
// requires WebpackEncoreBundle 1.4 or higher | |
//.enableIntegrityHashes() | |
// uncomment if you're having problems with a jQuery plugin | |
//.autoProvidejQuery() | |
// uncomment if you use API Platform Admin (composer req api-admin) | |
//.enableReactPreset() | |
//.addEntry('admin', './assets/js/admin.js') | |
; | |
module.exports = Encore.getWebpackConfig(); |
This is the configuration file that Encore reads. Actually, if you use Webpack by itself, you would have this exact same file! Encore is basically a configuration generator: you tell it how you want Webpack to behave and then, all the way at the bottom, say:
Please give me the standard Webpack config that will give me that behavior.
Encore makes things easy, but it's still true Webpack under-the-hood.
Most of the stuff in this file is for configuring some optional features that we'll talk about along the way - so ignore it all for now. The three super important things that we need to talk about are output path, public path and this addEntry()
thing:
var Encore = require('@symfony/webpack-encore'); | |
Encore | |
// directory where compiled assets will be stored | |
.setOutputPath('public/build/') | |
// public path used by the web server to access the output path | |
.setPublicPath('/build') | |
Show Lines
|
// ... lines 8 - 10 |
/* | |
* ENTRY CONFIG | |
* | |
* Add 1 entry for each "page" of your app | |
* (including one that's included on every page - e.g. "app") | |
* | |
* Each entry will result in one JavaScript file (e.g. app.js) | |
* and one CSS file (e.g. app.css) if you JavaScript imports CSS. | |
*/ | |
.addEntry('app', './assets/js/app.js') | |
//.addEntry('page1', './assets/js/page1.js') | |
//.addEntry('page2', './assets/js/page2.js') | |
Show Lines
|
// ... lines 23 - 65 |
; | |
Show Lines
|
// ... lines 67 - 68 |
Let's do that next, build our first Webpack'ed files and include them on the page.
68 Comments
Hey Mina,
Thank you for your interest in SymfonyCasts tutorials! I can't tell you when exactly this may happen, we have other tutorials in the queue that will be released soon. But I see what you mean. Unfortunately, this tutorial works only on PHP 7. But we added some notes to the video/scrips to make it easier for users to follow this course on a newer Symfony version. Feel free to download the latest Symfony version and follow this course on it. I understand it's not perfect because you will need to do more work, i.e. copy/paste some content from this tutorial, but that's still a valid case for older tutorials. As an alternative option, you can try to apply things from this tutorial in your personal project. In the future, we will definitely think about upgrading it, but not sure when exactly, too much good stuff prioritized first. :)
Thank you for your understanding and I hope I gave you some possible ideas on how to follow this course. And as always, of you get stuck somewhere following this course on a newer Symfony version - let us know in the comments below the video and our support team will help you ;)
Cheers!


Hi, I keep getting an error : "There is no extension able to load the configuration for "fos_user" " when trying to run cmd "composer install"
Same issue applies with any other composer require / install command.
I took the code from the download link too.
Can anyone help with this?
Thanks
Hey Jordan,
It seems you have a "fos_user:" config option somewhere in your config/ dir, most probably in config/packages/fos_user.yaml but it seems like you don't have that FOSUserBundle installed. You either should remove that config or install t he FOSUserBundle first so the config option could be read by that bundle.
Cheers!


Dont worry, this appeared because of an issue on my end! There was a mix up with code.
Thanks anyway!
Hey Jordan,
Great, thanks for confirming it was something related to your project code only.
Cheers!
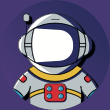
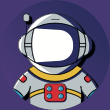
Very helpful tutorial for starting an example project.
A total disaster when you want to make a both interactive/seo project with a 100% Pagespeed score. {{parent}}, {{entry_link_tags}} and other stuff are totally useless and you have to make your head explode to find the best way to handling js/css/assets in general/ajax/static contents with maximun performances.
Hey Marcello,
Thank you for you feedback!
Performance optimization might be tough, yeah. Well, Webpack already do a lot of optimization for you behind the scene, but it also depends on how you write your JS code and what tools are using. For example, if you're working with jQuery - the compiled files won't be perfect for you then, better to get rid of jQuery if you can, that will increase the performance a lot. Though, if you have a big project, it might be very difficult to achieve 100% score I think.
Cheers!
Hi Marcello Kad Cadoni!
Just to follow up on what Victor said, I'm really sorry you hit a lot of "real world" issues - that's not at all what we want! If you have some specific problems that you overcame that we didn't cover (or didn't cover well), I would love to know what those are so we can do a better job in the future :).
Cheers!


Am I the only one who said "what the heck is yarn"? 😂 so I went to their home page, and they say to install yarn you need Corepack which needs Node, so ok I went to download nodejs... which at the setup wants to install also "Python and the Visual Studio Build Tools, necessary to compile Node.js native modules"... whaat I just needed to add some javascript to a php application 😅


Hi, I just started with this course. I am using Symfony 6 and php 8.1.3 and I have to run composer install --ignore-platform-reqs to ignore certain packages but still getting error in Markdown.php Compile Error: Array and string offset access syntax with curly braces is n
!! o longer supported I solved the error by going to the file and converting {} to []. My latest error is # unable to fetch the response from the backend: unexpected EOF with PhpMatcherDumper.php. Is there a simple way where you can update this course. Later on planning to take Vue.js as well any advice as I am running latest versions.
Hey Chintan,
Hm, most probably you just hide/postpone the root problem when you install dependencies with --ignore-platform-reqs. Could you try to install dependencies without that flag? Do you have any errors? Otherwise, it seems like knplabs/knp-markdown-bundle bundle may have some problems working on your PHP version, probably try to upgrade it to the latest first and try again. Or do you mean Markdown.php in src/ folder of this project?
Cheers!


Hey Victor, I have tried to install dependencies without --flag and error is showing many versions are above than the required one. Markdown and many other packages are backward compatible.
Hey Chintan,
Ah, I just noticed that you're on Symfony 6... did you decide to follow the course on your own project? Or did you donwload the course code and upgraded it to Symfony 6 yourself? We do recommend you to donwload the course code to match the exact code we have in the video, but upgrading dependencies may cause imcompatibility issues like one you mentioned. Also, I see tht this course isn't compatible with PHP 8 yet.
Cheers!
Yea, unfortunately, while we try to update our code a *bit* to stay compatible with newer versions of things, at some point, unless we change a LOT of things (which would make the code no longer match the video), we can't upgrade anymore. We're kinda stuck at this exact point with this tutorial at the moment :/.
But, we're happy to help debug at least. For this error:
> My latest error is # unable to fetch the response from the backend: unexpected EOF with PhpMatcherDumper.php
That's odd. That's coming from Symfony's routing component... and if you installed a fresh project, you should have the latest version of that. Did you start a fresh Symfony 6 project? Are you getting things working some other way?
Sorry for all the trouble - but, as I said, it just gets tricky as some tutorials get older :/.
Cheers!


Hi.
I downloaded the source code, but it's missing the 'var' and 'vendor' folders, which is apparently causing errors that don't let me start the symfony server:
`Warning: require([my route]/vendor/autoload.php): Failed to open stream: No such file or directory in [my route]\config\bootstrap.php on line 5
Fatal error: Uncaught Error: Failed opening required '[my route]/vendor/autoload.php' (include_path='.;C:\php\pear') in [my route]\config\bootstrap.php:5 Stack trace: #0 [my route]\public\index.php(7): require() #1 {main} thrown in [my route]\config\bootstrap.php on line 5`
Hey Jose,
That's totally fine that project code does not have vendor/ folder, you just need to follow the steps from the README file that you can find in the downloaded archive - basically, you just need to change dir to start/ and open README.md file there following the instructions in it. There's a step that explains how to install vendors with Composer package manager - you just need to run "composer install" command when you're in start/ dir. Also, there're a few more required commands you have to execute to start the project app successfully, just follow the steps in README.
Cheers!


Hi,
I wanted to do this course, so I went ahead and downloaded the source code as a first step. But it seems the .env file is missing:
PHP Fatal error: Uncaught Symfony\Component\Dotenv\Exception\PathException: Unable to read the "[...]/WebpackEncore/.env" environment file. in [...]/WebpackEncore/vendor/symfony/dotenv/Dotenv.php:465
So I went ahead and created it (...and I also set up a local database), but then I get the next error:
!! In EnvVarProcessor.php line 96:<br />!! <br />!! Environment variable not found: "SITE_BASE_URL". <br />!!
Any help would be greatly appreciated.
Kind regards & thanks
Hey Simon,
Did you download the course code and started from the start/ directory? I just downloaded the course code on this page and I see that .env file in the start/ directory. Please, re-download the course code and copy/paste that file to your project, or probably better start from that new curse code in case you didn't work on the project yet. Let us know if this does not help you!
Cheers!


Hi Victor,
Thanks, I just re-downloaded the source code, and now the .env file is there. I don't know what was wrong before, but it's all working fine now!
Best, Simon
Hey Simon,
Awesome, glad to hear it works for you now. I may have some ideas, if you copy/paste the content from that start/ folder manually to a different folder - you might miss dot files because they are usually hidden in the Finder, at least it's true for my Mac OS :) Better to move the whole dir and rename it or do copy via your terminal.
Cheers!


HI Victor,
You are absolutely right, I copied the contents of the start/ dir using the finder (from my downloads dir to a more logical place) and the .env indeed was hidden and did not get copied over!
Thanks again :)
Hey Simon,
Mystery solved :) Yeah, something that could be easily missed, I got this trap once too ;) Glad we figured it out, and I'm happy it's not related to our system and course downloads are correct.
Cheers!
Hello I am running composer require encore
and get errors due to dependencies' lock. I run composer require encore --with-all-dependencies
and still I get an error:
`Problem 1
- symfony/webpack-encore-bundle[v1.11.0, ..., v1.11.1] require symfony/asset ^4.4 || ^5.0 -> found symfony/asset[v4.4.0, ..., v4.4.19, v5.0.0, ..., v5.2.3] but the package is fixed to v4.2.12 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
- Root composer.json requires symfony/webpack-encore-bundle ^1.11 -> satisfiable by symfony/webpack-encore-bundle[v1.11.0, v1.11.1].
`
How can I resolve this problem?
Thanks
Hey Juan E.!
Ah, that's our fault! The version of Symfony that ships with this tutorial isn't compatible with the latest version of WebpackEncoreBundle. We need to add a note to the tutorial. The fix is just to install a slightly older version - which is fine, because the newer versions just contain a few new features that aren't important here. So, run this:
composer require "encore:^1.8"
Let me know if that helps!
Cheers!
Yes, it worked. Yet I got this error "An exception has been thrown during the rendering of a template Asset manifest file missing" which I fixed the following way:
yarn add --dev @symfony/webpack-encore
yarn add webpack-notifier --dev
yarn encore dev
Then I also run
composer require symfony/apache-pack
so I could get the debug bar running correctly.
thank you very much


how do i define a webpack_encore.yaml if I'm not using symfony flex?
Hey Dimitrios,
Technically, you can just paste the configuration from webpack_encore.yaml to your app/config/services.yaml or app/config.yml file, depending on the version of Symfony that is used in your project. In other words, just do the same you do for any other bundle configuration in your project.
Cheers!


How to preload a font or css file with Webpack Encore ?
Google PageSpeed Insights recommend me that...
Hey Helmis D.
Interesting question, which issue google found on you project? Honestly I can't remember this recommendation, that's why asking =)
Cheers!


Thanks for reply
https://developers.google.c...
in the print screen it’s in French
http://prntscr.com/t6ljz9
in short it says
Consider using `<link rel="preload">` to prioritize the recovery of resources currently required for subsequent page loading. Learn more at
https://web.dev/uses-rel-pr...
Hey Helmis D.
That's really interesting, I think, I understand why google recommends to use preload. The reason is slow response on this files from server. From my opinion it's better to improve files handling and speedup response from server. But you asked about how to add preload to links, so it's no possible with standard encore_entry_link_tags
, But you can do it with encore_entry_css_files
. it will return the array of css files. you will need to iterate through them and build your own <link>
tags with attributes you need!
Cheers!


Cool... Thanks sadikoff
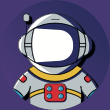
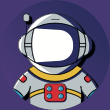
After installing with yarn, and reloading the symfony serve web server, I get the following exception: An exception has been thrown during the rendering of a template ("Asset manifest file "/Users/my-user/code-webpack-encore/start/public/build/manifest.json" does not exists").
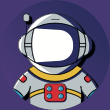
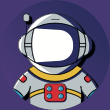
Nevermind! I was able to solve, running `./node_modules/.bin/encore dev` solved this. Thanks!
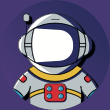
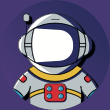
When I install composer require encore, I get the following warning:
Package zendframework/zend-code is abandoned, you should avoid using it. Use laminas/laminas-code instead.
Package zendframework/zend-eventmanager is abandoned, you should avoid using it. Use laminas/laminas-eventmanager instead.
Do I need to do something or can I ignore it?
Hey Anton Bagdatyev!
Good question. You can safely ignore those :). For the purposes of this tutorial, those packages are not important - zendframework was renamed to laminas, which is what is causing the warnings. So, ignore it and keep going! If this were a real project, I would eventually check into updating those - but for learning, the old ones won't cause any problems.
Cheers!
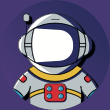
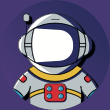
Sorry it not works at the moment
`$ yarn
yarn install v1.12.1
info No lockfile found.
[1/4] Resolving packages...
[2/4] Fetching packages...
error @symfony/webpack-encore@0.28.0: The engine "node" is incompatible with this module. Expected version "8.* || >= 10.*". Got "6.17.1"
error Found incompatible module
info Visit https://yarnpkg.com/en/docs/cli/install for documentation about this command.`
Hey isTom,
Hm, it looks like you have a really old Node JS, I'd recommend you to upgrade it, and then upgrade your Yarn as well, otherwise it seems JS dependencies we require in this project are too new your environment and cannot be installed. After you upgrade your Node and Yarn - you should be able to pull the dependencies.
I hope this helps!
Cheers!
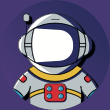
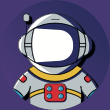
Hi !
How do we manage assets in a Bundle ? Like if i'm writting a Symfony bundle, should I use Webpack Encore to manage my bundle's assets or no ?
Hey @Paul!
GREAT question! The short answer is... you should probably not use Encore. Well, it depends... of course :p. You could choose to put some CSS and JS files into your bundle and then make it the responsibility of whatever app is using your bundle to import those from their own Encore files. In that case, Encore/Webpack isn't needed in your bundle as you're just creating some "source" files for someone to use and you expect them to process them through Encore.
But, speaking more broadly, in the "node" world, JS libraries typically write some "source" JavaScript & CSS files, where they use whatever new language features they want. They often then use Webpack to create "distribution" files from those source files. This means that their library ultimately ships with source files and distribution files (where maybe 5 source files have been combined into one and no "new" language features are used, like "import" or let/const, because their Webpack setup has compiled everything into a final version). If you want to do something like this, Encore is can be used, but probably isn't the best solution because it's opinionated to make Webpack work for end-user application files, not "library" files. For example, you might choose to use the output.library
setting in Webpack for a library (https://webpack.js.org/configuration/output/#outputlibrary), but this is not used in Encore (just because packaging files for use in an application versus packaging them for use as a "library" are a bit different cases).
Let me know if this helps!
Cheers!


Are these identical? composer require symfony/webpack-encore-bundle
and composer require encore
If not, should I require both of them through composer?
Hey Kensley L.
Yes, it's just a shortcut. You can check all composer aliases here: https://flex.symfony.com/
Cheers!


Hi, i can install encore in sf 3.4 without symfony flex?
Hey Fabio,
Yes, you can, just follow the installation guide: https://symfony.com/doc/3.4... . And in case you want to install WebpackEncoreBundle (you probably would like to install it) - here's the installation manual for it: https://github.com/symfony/... - don't forget to register this bundle manually.
Cheers!
ummm well, god morning...
I try to rum(Imean run, of course) your(our) course code on docker and the images are missing.
Not all the images, only the bd images.
Whats can I do?
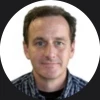
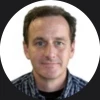
I had this same issue (images not loading) after a clean install of course code. The problem is that the image url's are using 'https' protocol.
To fix, I changed the SITE_BASE_URL var in .env to:
SITE_BASE_URL=http://localhost:8000
(from 'https' to 'http')
Hope that helps someone else! And maybe this change should probably be made in the course code as well?
Hey John christensen
You are right! It can be a problem if you are not running your local webserver with a SSL certificate (A thing that Symfony CLI enables by default). We will talk about this issue internally and come up with a solution.
Thanks!
Hey vespino_rojo
The images are not being displayed on your browser or the actual files are not there? I just double checked and the images are inside "start/public/images/" directory
Cheers!
thanks Diego!
Yes, the page show images, the ice cream, the meteor shower etc... but not the article image.
I suspect there are a problem with the liip bundle configuration.
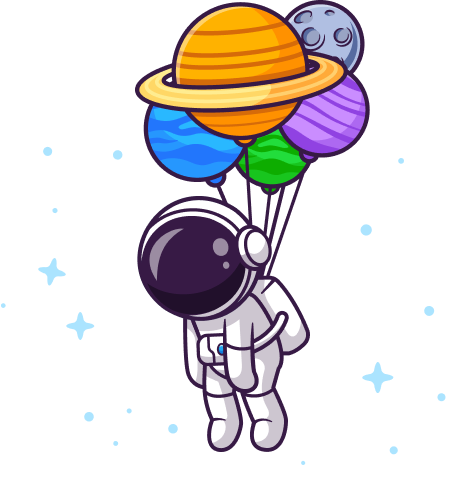
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.1.3",
"ext-iconv": "*",
"aws/aws-sdk-php": "^3.87", // 3.91.4
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/annotations": "^1.0", // 1.10.1
"doctrine/doctrine-bundle": "^1.6.10", // 1.10.2
"doctrine/doctrine-migrations-bundle": "^1.3|^2.0", // v2.0.0
"doctrine/orm": "^2.5.11", // v2.7.2
"knplabs/knp-markdown-bundle": "^1.7", // 1.7.1
"knplabs/knp-paginator-bundle": "^2.7", // v2.8.0
"knplabs/knp-time-bundle": "^1.8", // 1.9.0
"league/flysystem-aws-s3-v3": "^1.0", // 1.0.22
"league/flysystem-cached-adapter": "^1.0", // 1.0.9
"liip/imagine-bundle": "^2.1", // 2.1.0
"nexylan/slack-bundle": "^2.0,<2.2.0", // v2.1.0
"oneup/flysystem-bundle": "^3.0", // 3.0.3
"php-http/guzzle6-adapter": "^1.1", // v1.1.1
"phpdocumentor/reflection-docblock": "^3.0|^4.0", // 4.3.0
"sensio/framework-extra-bundle": "^5.1", // v5.3.1
"stof/doctrine-extensions-bundle": "^1.3", // v1.3.0
"symfony/asset": "^4.0", // v4.2.5
"symfony/console": "^4.0", // v4.2.5
"symfony/flex": "^1.9", // v1.21.6
"symfony/form": "^4.0", // v4.2.5
"symfony/framework-bundle": "^4.0", // v4.2.5
"symfony/property-access": "4.2.*", // v4.2.5
"symfony/property-info": "4.2.*", // v4.2.5
"symfony/security-bundle": "^4.0", // v4.2.5
"symfony/serializer": "4.2.*", // v4.2.5
"symfony/twig-bundle": "^4.0", // v4.2.5
"symfony/validator": "^4.0", // v4.2.5
"symfony/web-server-bundle": "^4.0", // v4.2.5
"symfony/webpack-encore-bundle": "^1.4", // v1.5.0
"symfony/yaml": "^4.0", // v4.2.5
"twig/extensions": "^1.5" // v1.5.4
},
"require-dev": {
"doctrine/doctrine-fixtures-bundle": "^3.0", // 3.1.0
"easycorp/easy-log-handler": "^1.0.2", // v1.0.7
"fzaninotto/faker": "^1.7", // v1.8.0
"symfony/debug-bundle": "^3.3|^4.0", // v4.2.5
"symfony/dotenv": "^4.0", // v4.2.5
"symfony/maker-bundle": "^1.0", // v1.11.5
"symfony/monolog-bundle": "^3.0", // v3.3.1
"symfony/phpunit-bridge": "^3.3|^4.0", // v4.2.5
"symfony/stopwatch": "4.2.*", // v4.2.5
"symfony/var-dumper": "^3.3|^4.0", // v4.2.5
"symfony/web-profiler-bundle": "4.2.*" // v4.2.5
}
}
What JavaScript libraries does this tutorial use?
// package.json
{
"devDependencies": {
"@symfony/webpack-encore": "^0.27.0", // 0.27.0
"autocomplete.js": "^0.36.0",
"autoprefixer": "^9.5.1", // 9.5.1
"bootstrap": "^4.3.1", // 4.3.1
"core-js": "^3.0.0", // 3.0.1
"dropzone": "^5.5.1", // 5.5.1
"font-awesome": "^4.7.0", // 4.7.0
"jquery": "^3.4.0", // 3.4.0
"popper.js": "^1.15.0",
"postcss-loader": "^3.0.0", // 3.0.0
"sass": "^1.29.0", // 1.29.0
"sass-loader": "^7.0.1", // 7.3.1
"sortablejs": "^1.8.4", // 1.8.4
"webpack-notifier": "^1.6.0" // 1.7.0
}
}
can you please update the tutorial files to the latest symphony version