Chapters
-
Course Code
Compatible PHP versions: ^7.1.3
Compatible PHP versions: ^7.1.3
- This Video
- Subtitles
- Course Script
Webpacking our First Assets
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
So, Webpack only needs to know three things. The first - setOutputPath()
- tells it where to put the final, built files and the second - setPublicPath()
- tells it the public path to this directory:
Show Lines
|
// ... lines 1 - 2 |
Encore | |
// directory where compiled assets will be stored | |
.setOutputPath('public/build/') | |
// public path used by the web server to access the output path | |
.setPublicPath('/build') | |
Show Lines
|
// ... lines 8 - 65 |
; | |
Show Lines
|
// ... lines 67 - 68 |
The Entry File
The third important piece, and where everything truly starts, is addEntry()
:
Tip
The Encore recipe now puts app.js
in assets/app.js
. But
the purpose of the file is exactly the same!
Show Lines
|
// ... lines 1 - 2 |
Encore | |
Show Lines
|
// ... lines 4 - 10 |
/* | |
* ENTRY CONFIG | |
* | |
* Add 1 entry for each "page" of your app | |
* (including one that's included on every page - e.g. "app") | |
* | |
* Each entry will result in one JavaScript file (e.g. app.js) | |
* and one CSS file (e.g. app.css) if you JavaScript imports CSS. | |
*/ | |
.addEntry('app', './assets/js/app.js') | |
//.addEntry('page1', './assets/js/page1.js') | |
//.addEntry('page2', './assets/js/page2.js') | |
Show Lines
|
// ... lines 23 - 65 |
; | |
Show Lines
|
// ... lines 67 - 68 |
Here's the idea: we point Webpack at just one JavaScript file - assets/js/app.js
. Then, it parses through all the import statements it finds, puts all the code together, and outputs one file in public/build
called app.js
. The first argument - app
- is the entry's name, which can be anything, but it controls the final filename: app
becomes public/build/app.js
.
And the recipe gave us a few files to start. Open up assets/js/app.js
:
Tip
The recipe now puts CSS files into an assets/styles/
directory.
So, assets/styles/app.css
- but the purpose of all these
files is the same.
/* | |
* Welcome to your app's main JavaScript file! | |
* | |
* We recommend including the built version of this JavaScript file | |
* (and its CSS file) in your base layout (base.html.twig). | |
*/ | |
// any CSS you require will output into a single css file (app.css in this case) | |
require('../css/app.css'); | |
// Need jQuery? Install it with "yarn add jquery", then uncomment to require it. | |
// const $ = require('jquery'); | |
console.log('Hello Webpack Encore! Edit me in assets/js/app.js'); |
This is the file that Webpack will start reading. There's not much here yet - a console.log()
and... woh! There is one cool thing: a require()
call to a CSS file! We'll talk more about this later, but in the same way that you can import other JavaScript files, you can import CSS too! And, by the way, this require()
function and the import
statement we saw earlier on Webpack's docs, do basically the same thing. More on that soon.
To make the CSS a bit more obvious, open app.css
and change the background to lightblue
and add an !important
so it will override my normal background:
body { | |
background-color: lightblue !important; | |
} |
disableSingleRuntimeChunk()
Before we execute Encore, back in webpack.config.js
, we need to make one other small tweak. Find the enableSingleRuntimeChunk()
line, comment it out, and put disableSingleRuntimeChunk()
instead:
Show Lines
|
// ... lines 1 - 2 |
Encore | |
Show Lines
|
// ... lines 4 - 26 |
// will require an extra script tag for runtime.js | |
// but, you probably want this, unless you're building a single-page app | |
//.enableSingleRuntimeChunk() | |
.disableSingleRuntimeChunk() | |
Show Lines
|
// ... lines 31 - 66 |
; | |
Show Lines
|
// ... lines 68 - 69 |
Don't worry about this yet - we'll see exactly what it does later.
Running Encore
Ok! We've told Webpack where to put the built files and which one file to start parsing. Let's do this! Find your terminal and run the Encore executable with:
./node_modules/.bin/encore dev
Tip
For Windows, your command may need to be node_modules\bin\encore.cmd dev
Because we want a development build. And... hey! A nice little notification that it worked!
And... interesting - it built two files: app.js
and app.css
. You can see them inside the public/build
directory. The app.js
file... well... basically just contains the code from the assets/js/app.js
file because... that file didn't import any other JavaScript files. We'll change that soon. But our app
entry file did require a CSS file:
Show Lines
|
// ... lines 1 - 7 |
// any CSS you require will output into a single css file (app.css in this case) | |
require('../css/app.css'); | |
Show Lines
|
// ... lines 10 - 15 |
And yea, Webpack understands this!
Here's the full flow. First, Webpack looks at assets/js/app.js
. It then looks for all the import
and require()
statements. Each time we import a JavaScript file, it puts those contents into the final, built app.js
file. And each time we import a CSS file, it puts those contents into the final, built app.css
file.
Oh, and the final filename - app.css
? It's app.css
because our entry is called app. If we changed this to appfoo.css
, renamed the file, then ran Encore again, it would still build app.js
and app.css
files thanks to the first argument to addEntry()
:
Show Lines
|
// ... lines 1 - 2 |
Encore | |
Show Lines
|
// ... lines 4 - 19 |
.addEntry('app', './assets/js/app.js') | |
Show Lines
|
// ... lines 21 - 66 |
; | |
Show Lines
|
// ... lines 68 - 69 |
Adding the Script & Links Tags
What this means is... we now have one JavaScript file that contains all the code we need and one CSS file that contains all the CSS! All we need to do is add them to our page!
Open up templates/base.html.twig
. Let's keep the existing stylesheets for now and add a new one: <link rel="stylesheet" href="">
the asset()
function and the public path: build/app.css
:
<!doctype html> | |
<html lang="en"> | |
<head> | |
Show Lines
|
// ... lines 5 - 8 |
{% block stylesheets %} | |
<link rel="stylesheet" href="{{ asset('build/app.css') }}"> | |
Show Lines
|
// ... lines 11 - 14 |
{% endblock %} | |
</head> | |
Show Lines
|
// ... lines 17 - 107 |
</html> |
At the bottom, add the script
tag with src="{{ asset('build/app.js') }}"
.
Tip
In new Symfony projects, the javascripts
block is at the top of this file - inside the <head>
tag.
We'll learn more about why in a few minutes.
<!doctype html> | |
<html lang="en"> | |
Show Lines
|
// ... lines 3 - 17 |
<body> | |
Show Lines
|
// ... lines 19 - 90 |
{% block javascripts %} | |
<script src="{{ asset('build/app.js') }}"></script> | |
Show Lines
|
// ... lines 93 - 105 |
{% endblock %} | |
</body> | |
</html> |
If you're not familiar with the asset()
function, it's not doing anything important for us. Because the build/
directory is our document root, we're literally pointing to the public path.
Let's try it! Move over, refresh and... hello, weird blue background. And in the console... yes! There's the log!
Tip
If you're coding along with a fresh Symfony project, you likely will not see
the console.log()
being printed. That's ok! In the next chapter, you'll learn
about a Twig function that will render some <script>
tags that you're
currently missing.
We've only started to scratch the surface of the possibilities of Webpack. So if you're still wondering: "why is going through this build process so useful?". Stay tuned. Because next, we're going to talk about the require()
and import
statements and start organizing our code.
47 Comments
Hey Marcel D.!
Hmm, nice questions! Let's look at each of them:
Why you decided to choose
build
directory as the output for the built files? It seems a bit weird and not common aspublic/css
andpublic/js
or something like that.
The main reason is that Webpack likes to have a single "build" directory that everything goes inside of. In order to have directories like public/css
and public/js
, we would have needed to set public
as the build directory. That's not a great choice because you want to have your build directory a bit isolated, for a few reasons. First, it's nice to be able to just "clean out" the entire build directory (without accidentally deleting other files) - there's an option in Encore to do that. Second, for deploying, you only need to upload the "built" files from Encore. So having them in one, isolated directory (with no other files), makes that easier. So, it's mostly because of Webpack's requirement that there be one build directory... and we want it to only contain Webpack stuff.
Other thing that seems odd to me is require CSS dependencies inside my JavaScript code.
Yea, this one can feel weird. The important thing to realize is that Webpack was created by people who primarily build single-page applications. And if your JavaScript is what is building your HTML... then it really makes perfect sense for each JavaScript file to require the CSS that it needs to style itself. If you're not building an SPA, it can sometimes feel weird.
'm used to have two different entries: one for my CSS file (compiled from a Sass source code) and another for JS file (compiled from my JavaScript source code). In fact, I'm used to split my JavaScript output in two different files: one for vendor (with jQuery, Parsley and other libraries) that almost don't change and can be cached for a long time and another for app (with my own code) that changes all the time.
Ok, so let's talk about this... because it sounds like you're already doing what Webpack wants you to do ;). Creating 1 CSS entry and 1 JS entry is really identical to creating 1 entry and then requiring your main SASS file from the top of that 1 .js file. The result in both cases is exactly the same: 1 final built CSS file and 1 final JS file. So, that's just my way of saying that if you do it your way... you're really already doing it the Webpack way.
Second, you're correct to split your vendor libraries from your app libraries. However... with the new splitEntryChunks()
feature, Webpack does that automatically. Check out that chapter if you haven't already - it's craziness.
Let me know if you still have some doubts :).
Cheers!
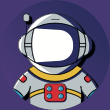
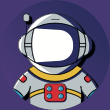
Hello ./node_modules/.bin/encore dev command is not working.
The encore is not found
But encore is there in package.json file
Hey Kiran Kaple!
Sorry for the troubles! Let's try a few things :)
1) Try running yarn install
if you haven't already. That populates the node_modules directory.
2) If running this doesn't help, try manually deleting the node_modules directory and running it again.
3) If THAT doesn't help, try running yarn encore dev
. If this last one works, then it's possible (though I haven't heard anything about it) that the way the node_modules/.bin directory is organized with yarn has changed. The above command tells yarn to "run encore" - and we actually switch to using a shorter command like this later :).
Let me know if this helps!
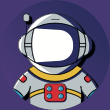
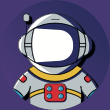
hello
May i know when the manifest.json file is generated
Hi Kiran Kaple!
Yes! This is generated when you execute encore/Webpack (Encore is a wrapper around Webpack). So, when you run, for example, "yarn encore dev". It's created at the same time as all of the other files in the public/build directory/.
Let me know if that helps :)
Cheers!
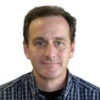
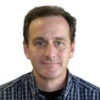
Hey there : While going through this tutorial a second time, I ran into something strange. I downloaded source, ran composer install
, yarn install
and then composer require "encore:^1.8"
. I now have a Symfony 4.2.5 app which runs great, although it differs slightly from the tutorial because it has the updated assets
folder structure.
After running ./node_modules/.bin/encore dev
and reloading the page, I do see the blue background set in app.css and I can see both the app.css <link> tag and the app.js <script> tag in the html source. But.. when I add my own console.log()
step (like the tutorial has) to the bottom of app.js, and rebuild assets, that statement never runs. I get nothing in the console. In fact, I can set a breakpoint in Chrome at that line, and it never gets hit. However, if i comment out the import './bootstrap';
line in app.js and rebuild assets, then everything works. The console.log() line executes and I can now set a breakpoint.
What is going on here? Why does the stimulus bootstrap.js file seem to prevent any other code from executing in app.js?
Here is my app.js file:
/*
* Welcome to your app's main JavaScript file!
*
* We recommend including the built version of this JavaScript file
* (and its CSS file) in your base layout (base.html.twig).
*/
// any CSS you import will output into a single css file (app.css in this case)
import './styles/app.css';
// start the Stimulus application
import './bootstrap';
// this line never executes; why not??
console.log('Hello Webpack Encore! Edit me in assets/app.js');```
Hey John christensen!
Hmm, this IS weird. In short, you shouldn't be seeing this behavior :). The bootstrap.js file (as you probably would have guessed, before seeing the weird behavior) initializes some stuff... but that's all: it doesn't block or prevent anything else from executing. So... I don't have an immediate answer. So let's do some debugging! Here's what I would try:
A) Open (like, in a new browser tab) the built app.js file and search for "Hello Webpack Encore". Do you see it in the built file? Do a force refresh to be sure - we don't want our browser cache to be causing problems :).
B) Remove the import './styles/app.css';
line entirely from app.js. When you refresh, you should now see NO styling. Does that happen? Or is the blue background still there?
My guess is that there is some simple problem: nothing fundamental has changed that should cause this.
Cheers!
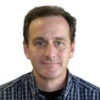
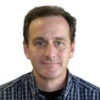
A) Yes; when I search in /build/app.js
it does find the console.log() line with 'Hello Webpack..' message. And B) if I remove app.css and hard-refresh, the blue does NOT go away immediately. But that appears to be because this version of Webpack-encore is not fully cleaning the build
folder (even though I have .cleanupOutputBeforeBuild()
in my webpack config). If I stop/start encore, then the cached .css file is removed and the blue background goes away. I still get no console.log message, but I do get a 404 error because I am still calling asset('build/app.css')
in base.html.twig
. Of course, if I comment that line out, the error goes away; no blue background; but also no log message.
But here is a big clue: if I comment out the .splitEntryChunks()
and restart encore (with yarn watch
), everything works! I can re-enable everything and it all runs correctly with expected console.log() message. So I think there may be a conflict with webpack-encore 1.8 (or more specifically, stimulus bridge) and splitEntryChunks.
Btw, I tested this with SingleRuntimeChunk
both enabled and disabled, but that made no difference. It's the splitEntryChunks feature that's seems to be causing the issue. I also tested this on two machines (one PC, one Mac) and with multiple browsers, but same problem. And all of that was with a fresh download of source.
I guess another way around this would be to disable the stimulus bridge altogether by commenting out .enableStimulusBridge('./assets/controllers.json')
in webpack config and import
./bootstrap';` in app.js. Users could then go back to the original folder structure which would make it easier to follow along with the tutorial.
Hey John christensen!
Ah, yes@! You figured it out :).
When you use splitEntryChunks()
, that built app.js
file is split into multiple files, and you need to include all of them. For this reason, instead of using assets('build/app.css')
, you should use the encore_entry_script_tags('app')
and encore_entry_link_tags('app')
Twig functions. These retrieve a list of ALL of the .js and .css files needed for the "app" entry.
In this tutorial - specifically in this chapter - I show asset('build/app.js') for simplicity at first. Why did this work in my tutorial but not in your version? When I recorded the tutorial, it's likely that, even though splitEntryChunks()
was enabled, there was not enough code yet for Webpack to actually split it. So build/app.js was the only file you needed. Now, specifically with the inclusion of the Stimulus stuff, out-of-the-box there IS enough code to cause the built app.js file to be "split". This means that including only that file isn't enough. In the NEXT chapter I talked about the Twig functions. But it does appear to be true that if you start this tutorial with the new fresh directory structure + Stimulus, you'll have problems with this chapter. I'll add a note :).
Cheers!
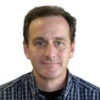
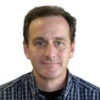
weaverryan Ah! That makes total sense now. And I now think I understand much better what all Webpack is doing behind scenes. Thx much for looking into this and for the detailed response :)


Hello.
I have created simple personal project where I have only one header(<h1>). No CSS and JS files.
When I download encore and use it I get LIGHTBLUE background color on my page. When I look in assets/styles/app.css the background color is set to LIGHTGRAY. Also in my build/app.css background color is set to LIGHTGRAY so I don't know where this LIGHTBLUE color is comming from.
Also I can't change the color of this lightblue background (i tried to set it to red but it is not working). My "yarn watch" is running all the time, and it also updates my build/app.css file to "background-color:red", but the red color never shows on my page. Also i tried to use "!important" but without success...
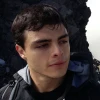
Hey there,
I believe there is something setting a style
property directly on your body HTML element (it could be a different HTML element). Double-check your HTML's source code to see if you can spot something odd
Cheers!


It says '.' is not recognized as internal or external command
when I try to run ./node_modules/.bin encore dev
also when I do encore$ ./node_modules/.bin encore dev
it says encore$ is not recognized
Hey @Farry7!
What operating system are you using - is it Windows? Try running:
yarn run encore dev
yarn run is basically a shortcut to execute the
encore executable in the
node_modules/.bin` directory. My guess (but I could be wrong) is that you're on Windows, and windows isn't liking the ./ syntax to execute that binary.
Let me know! Cheers!


./node_modules/.bin/encore dev
'.'is not recognized
I had a similar problem on php basics course but there I could use php bin/console instead of ./bin/console
I dont know what to use here.
Hey Farry7,
"php bin/console" and "./bin/console" are the same, it's the same "bin/console" file, but in the first you're process that file via PHP interpreter explicitly so it requires less permissions to be called. In the second - that file should have executable permissions to be able to run. So, you can use whatever option works for you, it's literally the same thing but in different ways.
Cheers!
Hi I have an issue, when I follow your instructions and I run ./node_modules/.bin/encore dev, iIget no error and the message ''Hello Webpack Encore! Edit me in assets/js/app.js appears in my console as expected, but I don't see my css style changes applied in my browser :(
Hey Jelle S.!
Well that's no fun! Let's check a few things :).
1) If you look at the HTML source of the page, do you see any <link rel="stylesheet">
tags? You should see one that points to /build/app.css
or, at least, something else in /build
(the exact filename(s) can vary for performance reasons).
2) If you DO see this link tag, what happens if you try to go to that URL directly in your browser? Can you see the CSS? Or do you see an error?
Let me know - I'm sure it's something little that we can work out.
Cheers!
Hi Ryan, sorry it was a typo in my rel="" tag... Whoooops
It works as intended now :)
Thank you for your reply!
Hey Jelle S.!
Nice find! I have *totally* done that before in a training... and it was SUPER hard to debug :p.
Cheers!
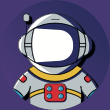
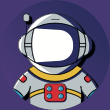
Hi,
I get the following syntax error while running webpack encore with following command:
$ ./node_module/.bin/encore dev
Error description:
/home/radhika/Docker/symfony5/node_modules/pkg-up/index.js:4
module.exports = async ({cwd} = {}) => findUp('package.json', {cwd});
^
SyntaxError: Unexpected token (
at createScript (vm.js:56:10)
at Object.runInThisContext (vm.js:97:10)
at Module._compile (module.js:549:28)
at Object.Module._extensions..js (module.js:586:10)
at Module.load (module.js:494:32)
at tryModuleLoad (module.js:453:12)
at Function.Module._load (module.js:445:3)
at Module.require (module.js:504:17)
at require (internal/module.js:20:19)
at Object.<anonymous> (/home/radhika/Docker/symfony5/node_modules/@symfony/webpack-encore/lib/config/parse-runtime.js:13:15)
Please Help.
Thanks in advance
Hey Radhika,
I suppose you just have a typo and run command:
$ ./node_modules/.bin/encore dev
where node_modules/ instead of node_module/
Regarding your errors, what version of NodeJS do you have installed? Run "node --version" to know it. I suppose your Node version is too old, or maybe too new for the current dependencies. In this course we're using Node v8, it's minimum required Node version to get it working.
Cheers!
Hi fellow coders!
I had an issue that I just resolved, so I share hear if anyone else has the same problem:
Short version: I got a BrowserlistError with the message: <blockquote>Unknown browser query basedir=$(dirname "$(echo "$0" | sed -e 's
. Maybe you are using old Browserslist or made typo in query.</blockquote>
I got the error when I ran encore dev
from within /node_modules/.bin/
. So that is appearantly not something you should do, but why did I try to do that? Well, I was following along with Ryan's coding and tried this at first: ./node_modules/.bin/encore dev
, but on Windows 10 I got:
<blockquote>'.' is not recognized as an internal or external command, operable program or batch file.</blockquote>
This led me to think that I had to go into the folder and run encore
from there. I thought wrong!
The solution (on Windows 10):.\node_modules\.bin\encore dev
See? I had to use backslashes instead of forward slashes. Silly me 😬
Hey anders
Great catch =) BTW there is a TIP on the page near the original command with advice for Windows users ;)node_modules\bin\encore.cmd dev
Use the force of the tips!
Cheers!
Thanks Vladimir!
Yeah, I actually did see that one but I didn't really copy what it said. My confused mind thought that the point was to use encore.cmd and I completely missed the backslashes. It took me removing and installing the code on different machines before I realized my mistake. Ick!

Hi there!
I get the following error in my console after running the encore command and trying it out:Failed to find a valid digest in the 'integrity' attribute for resource 'https://mydomain.com/build/app.js' with computed SHA-256 integrity '/fNLzADFdE1OKuBznqu1mB6ZbO+w9cnGd99loR18+tk='. The resource has been blocked.
I also do not see the console output, that should be there. What's the reason for this?
I IMMEDIATELY take everything back- I thought I need an integrity value, but it turns out, that I just forgot to close my script-tag at the end.... fixed!
Hey denizgelion
Yo! Great work! Keep going and ask questions if you got difficulties!
Cheers!
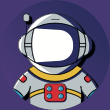
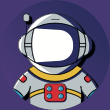
Hello again, if I run ./node_modules/.bin/encore dev
, I cannot create the Webpack bundle and get the following error:
$ ./node_modules/.bin/encore dev
Running webpack ...
Invalid configuration object. Webpack has been initialised using a configuration object that does not match the API schema.
- configuration.context: The provided value "/Users/antony/Self-development/Web development/Module bundlers/Webpack/Webpack Encore - Frontend like a Pro!/code-webpack-encore/start" contains exclamation mark (!
) which is not allowed because it's reserved for loader syntax.
-> The base directory (absolute path!) for resolving the `entry` option. If `output.pathinfo` is set, the included pathinfo is shortened to this directory.
- configuration.output.path: The provided value "/Users/antony/Self-development/Web development/Module bundlers/Webpack/Webpack Encore - Frontend like a Pro!/code-webpack-encore/start/public/build" contains ex
clamation mark (!) which is not allowed because it's reserved for loader syntax.
-> The output directory as **absolute path** (required).
I use Webpack 4:
$ npm list webpack
/Users/my-user/code-webpack-encore/start
└─┬ @symfony/webpack-encore@0.28.3
└── webpack@4.42.0
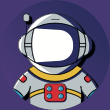
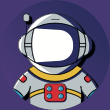
Nevermind! I was able to solve, there was a problem in my folder name, everything works now!!! Thanks!
Hi, I am using Windows 10 x64 1903. I do not see any notifications when build is completed. Are there any solutions for Windows users?
Hey Alexey!
Hm, Webpack Encore uses this https://github.com/Turbo87/... behind the scene, it from their readme it should work on Windows. Please, make sure you've installed and enabled it, though this issue looks related to your problem: https://github.com/Turbo87/... . Or was it opened by you? :) Anyway, nothing much I could say here, probably someone from maintainers or users might give some ideas on that issue.
Cheers!


Hi !
I'm very sorry, but I wanted to try that webpack encore, so i created a brand new S4 project on local. I just installed what I really needed (annotations, twig, toolbar and debug) then I installed Encore. Nothing else I swear ! So I watched first the entire course, then I did exactly what was written in the first chapter, typed "node_modules\.bin\encore.cmd dev" (by the way it misses the dot for the windows line) - I was pretty excited at that time - aaaaaand...Error : Module Build Failed (from ;/node_modules/babel-loader/lib/index.js): BrowserslistError: [BABEL] C:\wamp64\www\la_marine\assets\js\app.js: Unknown browser query `basedir=$(dirname "$(echo "$0" | sed -e 's`. Maybe you are using old Browserslist or made typo in query...
I have no idea what I did wrong. What did I miss ?
Thank you ! (as usual, awesome course)
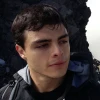
Hey Jean-tilapin looks like your babel config is wrong. Can you show me your webpack.config.js & package.json file? Thanks!


Of course, thank you Diego.
webpack.config.js is still the default one (I haven't touched it yet)
`
var Encore = require('@symfony/webpack-encore');
Encore
// directory where compiled assets will be stored
.setOutputPath('public/build/')
// public path used by the web server to access the output path
.setPublicPath('/build')
// only needed for CDN's or sub-directory deploy
//.setManifestKeyPrefix('build/')
/*
* ENTRY CONFIG
*
* Add 1 entry for each "page" of your app
* (including one that's included on every page - e.g. "app")
*
* Each entry will result in one JavaScript file (e.g. app.js)
* and one CSS file (e.g. app.css) if you JavaScript imports CSS.
*/
.addEntry('app', './assets/js/app.js')
//.addEntry('page1', './assets/js/page1.js')
//.addEntry('page2', './assets/js/page2.js')
// When enabled, Webpack "splits" your files into smaller pieces for greater optimization.
.splitEntryChunks()
// will require an extra script tag for runtime.js
// but, you probably want this, unless you're building a single-page app
.enableSingleRuntimeChunk()
/*
* FEATURE CONFIG
*
* Enable & configure other features below. For a full
* list of features, see:
* https://symfony.com/doc/current/frontend.html#adding-more-features
*/
.cleanupOutputBeforeBuild()
.enableBuildNotifications()
.enableSourceMaps(!Encore.isProduction())
// enables hashed filenames (e.g. app.abc123.css)
.enableVersioning(Encore.isProduction())
// enables @babel/preset-env polyfills
.configureBabel(() => {}, {
useBuiltIns: 'usage',
corejs: 3
})
// enables Sass/SCSS support
//.enableSassLoader()
// uncomment if you use TypeScript
//.enableTypeScriptLoader()
// uncomment to get integrity="..." attributes on your script & link tags
// requires WebpackEncoreBundle 1.4 or higher
//.enableIntegrityHashes()
// uncomment if you're having problems with a jQuery plugin
//.autoProvidejQuery()
// uncomment if you use API Platform Admin (composer req api-admin)
//.enableReactPreset()
//.addEntry('admin', './assets/js/admin.js')
;
module.exports = Encore.getWebpackConfig();
`
And my package.json :
`{
"devDependencies": {
"@symfony/webpack-encore": "^0.27.0",
"core-js": "^3.0.0",
"webpack-notifier": "^1.6.0"
},
"license": "UNLICENSED",
"private": true,
"scripts": {
"dev-server": "encore dev-server",
"dev": "encore dev",
"watch": "encore dev --watch",
"build": "encore production --progress"
}
}
`
Basically, it's still the default configuration. I just started a new S4 project to test webpack !
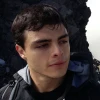
Hmm, that's weird, I don't get it why you are getting problems with Browserslist if you didn't configure it. What's your code in `app.js`?
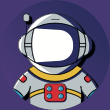
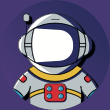
Hi there,
I'm able to follow along and install everything up to this point but when I load my app I get the following Symfony error:
"ClassNotFoundException
Attempted to load class "WebpackEncoreBundle" from namespace "Symfony\WebpackEncoreBundle".
Did you forget a "use" statement for another namespace?"
I'm new to Symfony, and after searching multiple times for a solution to this error I can't seem to find one. Do you have any idea what could be causing this?
Thanks!
Hey Jennifer,
Please, make sure you have the exact line in your config/bundles.php file:
return [
// ...
Symfony\WebpackEncoreBundle\WebpackEncoreBundle::class => ['all' => true],
Also, make sure your cache is cleared by running in the terminal:
$ bin/console cache:clear
Btw, what Symfony version do you use? Is "composer require encore" command executed without any errors for you?
Cheers!
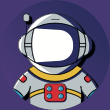
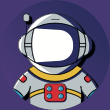
Hi victor ,
Thanks for your help with this! I checked and that line exists in config/bundles.php. I was able to clear the cache successfully but I'm still seeing the same error message. I'm using version 4.2 of Symfony. Here's what I see when I run "composer require encore":<br />Using version ^1.5 for symfony/webpack-encore-bundle<br />./composer.json has been updated<br />Loading composer repositories with package information<br />Updating dependencies (including require-dev)<br />Restricting packages listed in "symfony/symfony" to "4.2.*"<br />Nothing to install or update<br />Generating autoload files<br />ocramius/package-versions: Generating version class...<br />ocramius/package-versions: ...done generating version class<br />Executing script cache:clear [OK]<br />Executing script assets:install public [OK]<br />Executing script security-checker security:check [OK]<br />
Hey Jennifer,
Hm, looks good! Then, what page are you trying to access? Did you download course code or did you create an empty Symfony 4.2 project from scratch?
Cheers!
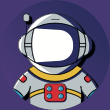
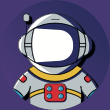
I fixed it. I'm running my application in Docker and I'm new to Docker so I didn't realize I needed to run a build and then restart. I really appreciate your help with this issue.
Ah, ok, that's great! Glad you fixed it :)
Cheers!
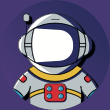
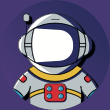
Hi Victor,
I'm trying to add it to an existing project at work. I'm getting that error on any template I try to load. Here's the full message I'm seeing:
`
ClassNotFoundException
Attempted to load class "WebpackEncoreBundle" from namespace "Symfony\WebpackEncoreBundle".
Did you forget a "use" statement for another namespace?
in Kernel.php line 33
at Kernel->registerBundles()
in Kernel.php line 424
at Kernel->initializeBundles()
in Kernel.php line 130
at Kernel->boot()
in Kernel.php line 193
at Kernel->handle(object(Request))
in index.php line 37
`
Hey Jennifer,
OK, what version of Symfony you're on? And what exactly version of WebpackEncoreBundle is installed? You can check it with Composer:
$ composer info symfony/webpack-encore-bundle
Actually, "Symfony\WebpackEncoreBundle\WebpackEncoreBundle" is the correct namespace. Did you install symfony/webpack-encore-bundle globally, i.e. is it in "require" or in "require-dev" section of your composer.json? Hm, let's try to remove and install it again:
$ composer remove symfony/webpack-encore-bundle
$ composer require symfony/webpack-encore-bundle
Do you still see the same error?
Cheers!


Hi @weaverryan,
In your video i see autocompletion on file webpack.config.js. I'm using Phpstorm as well, but there is no autocompletion, does i needs to configure this?
In PHPstorm i see the following message:
Can't analyse webpack.config.js: coding assistance will ignore module resolution rules in this file.
Possible reasons: this file is not a valid webpack configuration file or its format is not currently supported by the IDE. Error details: Cannot find module '@symfony/webpack-encore'
#UPDATE
Fixed it, i was trying to run node and yarn through vagrant but when i run it localy all files are generated correctly.
Nice find Bertin! A very annoying issue :)
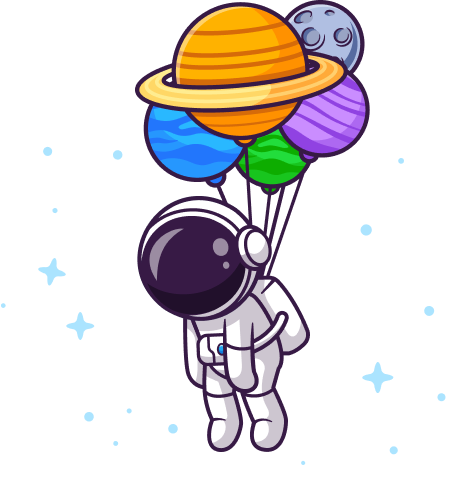
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.1.3",
"ext-iconv": "*",
"aws/aws-sdk-php": "^3.87", // 3.91.4
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/annotations": "^1.0", // 1.10.1
"doctrine/doctrine-bundle": "^1.6.10", // 1.10.2
"doctrine/doctrine-migrations-bundle": "^1.3|^2.0", // v2.0.0
"doctrine/orm": "^2.5.11", // v2.7.2
"knplabs/knp-markdown-bundle": "^1.7", // 1.7.1
"knplabs/knp-paginator-bundle": "^2.7", // v2.8.0
"knplabs/knp-time-bundle": "^1.8", // 1.9.0
"league/flysystem-aws-s3-v3": "^1.0", // 1.0.22
"league/flysystem-cached-adapter": "^1.0", // 1.0.9
"liip/imagine-bundle": "^2.1", // 2.1.0
"nexylan/slack-bundle": "^2.0,<2.2.0", // v2.1.0
"oneup/flysystem-bundle": "^3.0", // 3.0.3
"php-http/guzzle6-adapter": "^1.1", // v1.1.1
"phpdocumentor/reflection-docblock": "^3.0|^4.0", // 4.3.0
"sensio/framework-extra-bundle": "^5.1", // v5.3.1
"stof/doctrine-extensions-bundle": "^1.3", // v1.3.0
"symfony/asset": "^4.0", // v4.2.5
"symfony/console": "^4.0", // v4.2.5
"symfony/flex": "^1.9", // v1.21.6
"symfony/form": "^4.0", // v4.2.5
"symfony/framework-bundle": "^4.0", // v4.2.5
"symfony/property-access": "4.2.*", // v4.2.5
"symfony/property-info": "4.2.*", // v4.2.5
"symfony/security-bundle": "^4.0", // v4.2.5
"symfony/serializer": "4.2.*", // v4.2.5
"symfony/twig-bundle": "^4.0", // v4.2.5
"symfony/validator": "^4.0", // v4.2.5
"symfony/web-server-bundle": "^4.0", // v4.2.5
"symfony/webpack-encore-bundle": "^1.4", // v1.5.0
"symfony/yaml": "^4.0", // v4.2.5
"twig/extensions": "^1.5" // v1.5.4
},
"require-dev": {
"doctrine/doctrine-fixtures-bundle": "^3.0", // 3.1.0
"easycorp/easy-log-handler": "^1.0.2", // v1.0.7
"fzaninotto/faker": "^1.7", // v1.8.0
"symfony/debug-bundle": "^3.3|^4.0", // v4.2.5
"symfony/dotenv": "^4.0", // v4.2.5
"symfony/maker-bundle": "^1.0", // v1.11.5
"symfony/monolog-bundle": "^3.0", // v3.3.1
"symfony/phpunit-bridge": "^3.3|^4.0", // v4.2.5
"symfony/stopwatch": "4.2.*", // v4.2.5
"symfony/var-dumper": "^3.3|^4.0", // v4.2.5
"symfony/web-profiler-bundle": "4.2.*" // v4.2.5
}
}
What JavaScript libraries does this tutorial use?
// package.json
{
"devDependencies": {
"@symfony/webpack-encore": "^0.27.0", // 0.27.0
"autocomplete.js": "^0.36.0",
"autoprefixer": "^9.5.1", // 9.5.1
"bootstrap": "^4.3.1", // 4.3.1
"core-js": "^3.0.0", // 3.0.1
"dropzone": "^5.5.1", // 5.5.1
"font-awesome": "^4.7.0", // 4.7.0
"jquery": "^3.4.0", // 3.4.0
"popper.js": "^1.15.0",
"postcss-loader": "^3.0.0", // 3.0.0
"sass": "^1.29.0", // 1.29.0
"sass-loader": "^7.0.1", // 7.3.1
"sortablejs": "^1.8.4", // 1.8.4
"webpack-notifier": "^1.6.0" // 1.7.0
}
}
Hi @weaverryan!
Why you decided to choose `build` directory as the output for the built files? It seems a bit weird and not common as `public/css` and `public/js` or something like that.
Other thing that seems odd to me is require CSS dependencies inside my JavaScript code. I'm used to have two different entries: one for my CSS file (compiled from a Sass source code) and another for JS file (compiled from my JavaScript source code). In fact, I'm used to split my JavaScript output in two different files: one for vendor (with jQuery, Parsley and other libraries) that almost don't change and can be cached for a long time and another for app (with my own code) that changes all the time.
[]s