Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: ^7.1.3
Subscribe to download the code!Compatible PHP versions: ^7.1.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Question: have you ever gone to the store and accidentally bought too much cheese? It's the story of my life. Or maybe you have the opposite problem: you're hosting a big party and you don't have enough cheese! This is our new billion dollar idea: a platform where you can sell that extra chunk of Brie you never finished or buy a truck-load of camembert from someone who go a little too excited at the cheese market. Yep, it's what the world is asking for: a peer-to-peer cheese marketplace. We're calling it: Cheese Whiz.
For the site, maybe we're going to make it an single-page application built in React or Vue... or maybe it'll be a little more traditional: a mixture of HTML pages and JavaScript that makes AJAX requests. And maybe we'll even have a mobile app. It doesn't really matter because all of these options mean that we need to be able to expose our core functionality via an API.
Generating the Entity
But to start: forget about the API and pretend like this is a normal, boring Symfony project. Step 1 is... hmm, probably do create some database entities.
Let's open up our .env
file and tweak the DATABASE_URL
. My computer uses root
with no password... and how about cheese_whiz
for the database name.
Show Lines
|
// ... lines 1 - 30 |
DATABASE_URL=mysql://root:@127.0.0.1:3306/cheese_whiz | |
Show Lines
|
// ... lines 32 - 33 |
You can also create a .env.local
file and override DATABASE_URL
there. Using root
and no password is pretty standard, so I like to add this to .env
and commit it as the default.
Cool! Next, at your terminal, run
composer require maker:1.11 --dev
to get Symfony's MakerBundle... so we can be lazy and generate our entity. When that finishes, run:
php bin/console make:entity
Call the first entity: CheeseListing
, which will represent each "cheese" that's for sale on the site. Hit enter and... oh! It's asking:
Mark this class as an API Platform resource?
MakerBundle asks this because it noticed that API Platform is installed. Say "yes". And before we add any fields, let's go see what that did! In my editor, yep! This created the usual CheeseListing
and CheeseListingRepository
. Nothing special there. Right now, the only property the entity has is id
. So, what did answering "yes" to the API Platform resource question give us? This tiny annotation right here: @ApiResource
.
Show Lines
|
// ... lines 1 - 7 |
/** | |
* @ApiResource() | |
Show Lines
|
// ... line 10 |
*/ | |
class CheeseListing | |
Show Lines
|
// ... lines 13 - 111 |
The real question is: what does that activate? We'll see that soon.
But first, let's add some fields. Let's see, each cheese listing probably needs a title
, string
, 255
, not nullable, a description
, which will be a big text field, price
, which I'll make an integer
- this will be the price in cents - so $10 would be 1000, createdAt
as a datetime
and an isPublished
boolean. Ok: good start! Hit enter to finish.
Congratulations! We have a perfectly boring CheeseEntity
class: 7 properties with getters and setters.
Show Lines
|
// ... lines 1 - 11 |
class CheeseListing | |
{ | |
/** | |
* @ORM\Id() | |
* @ORM\GeneratedValue() | |
* @ORM\Column(type="integer") | |
*/ | |
private $id; | |
/** | |
* @ORM\Column(type="string", length=255) | |
*/ | |
private $title; | |
/** | |
* @ORM\Column(type="text") | |
*/ | |
private $description; | |
/** | |
* @ORM\Column(type="integer") | |
*/ | |
private $price; | |
/** | |
* @ORM\Column(type="datetime") | |
*/ | |
private $createdAt; | |
/** | |
* @ORM\Column(type="boolean") | |
*/ | |
private $isPublished; | |
Show Lines
|
// ... lines 45 - 109 |
} |
Next, generate the migration with:
php bin/console make:migration
Oh! Migrations isn't installed yet! No problem, follow the recommendation:
composer require migrations:2.0.0
But before we try generating it again, I need to make sure my database exists:
php bin/console doctrine:database:create
And now run make:migration
:
php bin/console make:migration
Let's go check that out to make sure there aren't any surprises:
CREATE TABLE cheese_listing...
Show Lines
|
// ... lines 1 - 12 |
final class Version20190508193750 extends AbstractMigration | |
{ | |
Show Lines
|
// ... lines 15 - 19 |
public function up(Schema $schema) : void | |
{ | |
// this up() migration is auto-generated, please modify it to your needs | |
$this->abortIf($this->connection->getDatabasePlatform()->getName() !== 'mysql', 'Migration can only be executed safely on \'mysql\'.'); | |
$this->addSql('CREATE TABLE cheese_listing (id INT AUTO_INCREMENT NOT NULL, title VARCHAR(255) NOT NULL, description LONGTEXT NOT NULL, price INT NOT NULL, created_at DATETIME NOT NULL, is_published TINYINT(1) NOT NULL, PRIMARY KEY(id)) DEFAULT CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci ENGINE = InnoDB'); | |
} | |
Show Lines
|
// ... lines 27 - 34 |
} |
Yea! Looks good! Close that and run:
php bin/console doctrine:migrations:migrate
Say Hello to your API!
Brilliant! At this point, we have a completely traditional Doctrine entity except for this one, @ApiResource()
annotation. But that changes everything. This tells API Platform that you want to expose this class as an API.
Check it out: refresh the /api
page. Woh! Suddenly this is saying that we have five new endpoints, or "operations"! A GET
operation to retrieve a collection of CheeseListings, a POST
operation to create a new one, GET
to retrieve a single CheeseListing
, DELETE
to... ya know... delete and PUT
to update an existing CheeseListing
. That's a full, API-based CRUD!
And this isn't just documentation: these new endpoints already work. Let's check them out next, say hello to something called JSON-LD and learn a bit about how this magic works behind the scenes.
73 Comments
Hey Dominik P.!
Sorry for the slow reply (I had some vacation) and especially for the trouble! This tutorial IS starting to show its age... but I'm not aware of any issues (other than the biggest that the downloadable code only works with PHP 7.*). If you're hitting specific problems, let us know and we'll do our best to help debug :). We will probably update these course soon anyways, as API Platform v3 will be released soon.
Cheers!
Hi
A little update for composer migrations dependencies.
Currently composer try to install ^3.1.
To make it work (and be consistent with finish project) I use composer require doctrine/doctrine-migrations-bundle "^2.0"


Thanks it helped!!
Hey atournayre!
Thanks for the note about this! We'll take a look at it and add a note if necessary to the video :).
Question: did installing version 3.1 cause some problems (e.g. it gave a composer dependency error)?
Thanks!
hi, i have a problem..bin/console make:entity<br />".bin" no se reconoce como un comando interno o externo,<br />programa o archivo por lotes ejecutable.<br />
when i execute .bin/console make:entity
Hey Sebastian R.
Yep the error is in command, there shouldn't be a dot before bin
use justbin/console make:entity
or php bin/console make:entity
Cheers!
Thanks.


Hi, I've a problem when running:
php bin/console make:entity
Class name of the entity to create or update (e.g. AgreeableElephant):
> CheeseListing
Your entity already exists! So let's add some new fields!
New property name (press <return> to stop adding fields):
> title
[ERROR] An exception occurred in driver: SQLSTATE[HY000] [2002] php_network_getaddresses: getaddrinfo failed: nodename
nor servname provided, or not known
In the .env file I've this:
# In all environments, the following files are loaded if they exist,
# the later taking precedence over the former:
#
# * .env contains default values for the environment variables needed by the app
# * .env.local uncommitted file with local overrides
# * .env.$APP_ENV committed environment-specific defaults
# * .env.$APP_ENV.local uncommitted environment-specific overrides
#
# Real environment variables win over .env files.
#
# DO NOT DEFINE PRODUCTION SECRETS IN THIS FILE NOR IN ANY OTHER COMMITTED FILES.
#
# Run "composer dump-env prod" to compile .env files for production use (requires symfony/flex >=1.2).
# https://symfony.com/doc/current/best_practices/configuration.html#infrastructure-related-configuration
###> symfony/framework-bundle ###
APP_ENV=dev
APP_SECRET=1c78f3e5e06eb3c88a52819fbccdba6e
#TRUSTED_PROXIES=127.0.0.1,127.0.0.2
#TRUSTED_HOSTS='^localhost|example\.com$'
###< symfony/framework-bundle ###
###> doctrine/doctrine-bundle ###
# Format described at https://www.doctrine-project.org/projects/doctrine-dbal/en/latest/reference/configuration.html#connecting-using-a-url
# IMPORTANT: You MUST configure your server version, either here or in config/packages/doctrine.yaml
#
# DATABASE_URL="sqlite:///%kernel.project_dir%/var/data.db"
# DATABASE_URL="mysql://app:!ChangeMe!@127.0.0.1:3306/app?serverVersion=8"
# DATABASE_URL=mysql://root:@127.0.0.1:3306/cheese_whiz
DATABASE_URL=mysql://root:root@mysql:3306/cheese_whiz
###< doctrine/doctrine-bundle ###
###> nelmio/cors-bundle ###
CORS_ALLOW_ORIGIN='^https?://(localhost|127\.0\.0\.1)(:[0-9]+)?$'
###< nelmio/cors-bundle ###
Cheers!
Hey Rango-L,
First of all, please make sure that Doctrine has access to your DB. The simplest command check is:
$ bin/console doctrine:schema:validate
I suppose you will get the same error, but just in case let's double-check. And if so - you really have problems with your DB credentials. You can change them in .env
, but also make sure that you don't have that DATABASE_URL
in your .env.local
file because that will overwrite the credentials from your .env
. You should either change credentials in both places, or at least in .env.local
if you have it.
Also, make sure that credentials are correct the DATABASE_URL
- I see you have username root
and password root
- most probably you have no password for MySQL? Usually, that line is written without a password if you have the default MySQL config. And probably the host should be localhost
instead of mysql
, i.e.:
DATABASE_URL=mysql://root@localhost:3306/cheese_whiz
Also, make sure you're started the MySQL server locally. Or are you using Docker?
Cheers!


Hey victor,
Thank you for your reply, the first thing I have done is to change the DATABASE_URL
to the one you have indicated.
DATABASE_URL=mysql://root:@localhost:3306/cheese_whiz
I have seen that I don't have Doctrine installed, but I can't install any version because it gives me incompatibilities with Symfony or Composer.
Jonatan@MacBook-Pro-de-Jon start % composer require doctrine/doctrine-migrations-bundle
Info from https://repo.packagist.org: #StandWithUkraine
Using version ^3.2 for doctrine/doctrine-migrations-bundle
./composer.json has been updated
Running composer update doctrine/doctrine-migrations-bundle
Loading composer repositories with package information
Updating dependencies
Your requirements could not be resolved to an installable set of packages.
Problem 1
- doctrine/doctrine-migrations-bundle[3.2.0, ..., 3.2.2] require doctrine/migrations ^3.2 -> found doctrine/migrations[3.2.0, ..., 3.5.2] but the package is fixed to 3.0.1 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
- Root composer.json requires doctrine/doctrine-migrations-bundle ^3.2 -> satisfiable by doctrine/doctrine-migrations-bundle[3.2.0, 3.2.1, 3.2.2].
Use the option --with-all-dependencies (-W) to allow upgrades, downgrades and removals for packages currently locked to specific versions.
You can also try re-running composer require with an explicit version constraint, e.g. "composer require doctrine/doctrine-migrations-bundle:*" to figure out if any version is installable, or "composer require doctrine/doctrine-migrations-bundle:^2.1" if you know which you need.
Installation failed, reverting ./composer.json and ./composer.lock to their original content.
I have followed all the steps in video 1 and this one and at no point does it say to install MySql or Docker, so I haven't even started it.
Also note that I only have one .env
file (I have not created any .env.local
file).
I would like you to tell me what steps I should take and what versions of all the packages we need to do the course, as it is outdated and it is impossible to go on.
Thank you.
Hey Rango,
Hm, why do you install that doctrine/doctrine-migrations-bundle
package? It's not about Doctrine, it's about migrations actually.
Well, OK! Let's go in a different direction. First of all, you need to download the course code and start from the start/ directory. Also, in the start/ directory you will find a README file that contains instructions on how to bootstrap the course project website. Let me know if you could finish all those steps from the README, or in case you get stuck somewhere following them. It just should work if you follow all the steps without any errors. So far it sounds like you missed some of those required steps from the README.
Cheers!
Running ./bin/console make:entity
, I was getting an error from DoctrineHelper.php:line 314 when it tried to create the empty entity files. composer require maker:1.11 --dev
was giving me maker-bundle 1.35. Being harder about the version and using composer required maker:~1.11.0
seems to have fixed it for me - possibly something in 1.35 that breaks the project? That might or might not have been the correct way to fix it...
Hey Paul-L!
Sorry for the slow reply! That's super weird that composer require maker:1.11 --dev
would give you 1.35! Anyways, I think the only reason we show using 1.11 in the tutorial is in case you're using OUR code to code along. In that case, iirc, you need to specify 1.11 so you get an old enough version of MakerBundle that is compatible with this version of Symfony. But, in general, you should use the latest and greatest.
Anyways, it looks like you hit this issue - https://github.com/symfony/maker-bundle/issues/1001 - which was fixed in 1.36!
Cheers!
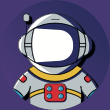
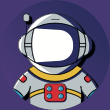
Hey
When I generate an Entity with this command "php bin/console make:entity" in the Entity Class aren't the Annotations like in this Video.
Is there maybe a configuration I missed because the generated code looks like following:
`
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
use App\Repository\CheeseListingRepository;
use Doctrine\ORM\Mapping as ORM;
#[ORM\Entity(repositoryClass: CheeseListingRepository::class)]
#[ApiResource]
class CheeseListing
{
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column(type: 'integer')]
private $id;
public function getId(): ?int
{
return $this->id;
}
}
`
I highly prefer annotations, so it would be nice if I dont need to rewrite the auto generated by hand.
Hey Yannick Fuchs!
Ah yes! MakerBundle is detecting that you support PHP 8 attributes and is using those instead. There's no way to configure/disable this... because attributes are "taking over". Eventually, annotations will be deprecated in favor of them.
So... my best recommendation would be to leave them and get used to looking at them :). They looked weird to me after first, but I've grown to really like them. You can also use attributes for the API Platform stuff too (which you probably guess by the #[ApiResource]
.
Cheers!
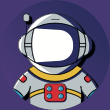
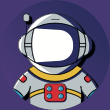
thank you for the quick help! Then I will have a look into the PHP 8 attributes :)


Seriously, just go and try to built it every 1-2 months. I got 4 errors trying to follow your steps.
What I did was just upgrade everything and change composer.json manually to get this to work. I got errors in maker, migrations.
You have to spend some time and fix this, else people are just wasting time.
Yea, sorry about that @Petros_Z :/. As Victor mentioned, we've recently done a bunch of work internally to track whether or not our course code continues to build correctly as new versions of PHP come out. That system is now in place, but we're still working through tweaks to some courses - including this one. Thanks for pushing us on this, and I'm sorry you had so many problems - that's not the experience we want!
Hey Petros_Z,
We're sorry to hear you have some issues following this tutorial and thank you for letting us know this tutorial does not work properly on a newer version of the library.
We do recommend you to download the code and code long with us using the same version as we have in the video, for this you need to go to the start/ directory of the course and execute "composer install" instead of "composer update". The course should work on locked versions that we were using in the video.
But you're right, we also need to update this tutorial wit the latest changes in the library. For this, we recently wrote an internal system that will automatically help with testing the tutorial to find has any problems on newer version of the library in the future. We do see this course fails right now, and we will add some notes and fixes to it so people could follow it on a newer version.
So, while we're working on fixing this tutorial, I'd recommend you to follow the tutorial on locked versions instead, or you can always leave a comment with a problem where you stuck describing the issue and our team will help in case you're following this tutorial on a newer version.
Cheers!


Thanks for replying but I wouldn't make the comment if things were working with the locked versions.
In your script you require maker (without a version). That didn't work because it probably requires a different version than yours.
I know these stuff are difficult to follow up, don't get me wrong. But what I am suggesting is that you simply try to run your commands once every 1-2 months. I am sure that would solve half the problems people are having.
I was ready to just drop the course all together. If I have to hunt down the comments and try 10 different things just to be able to code along, it's not worth the trouble.
Also some of my colleagues are not going to solve these kind of problems so I can't even imagine the wasted time.
Hey Petros_Z,
Ah, you're right! Yes, during the course, you will get latest version of the installed library. Just a quick workaround would be to peep on the versions installed in our videos, you can do this on the course intro page pressing on the "Symfony 4" button, or on any chapter page like the current one in the tab called "Versions" near the "Conversation" tab. For example, the version of "symfony/maker-bundle" installed in our course code is "v1.11.6". You can try to require this exact version with:
composer require "symfony/maker-bundle:v1.11.6"
I understand that's not perfect solution, more like a workaround, but this should do the trick and unblock you from following the tutorial while we're working on adding notes and fixing the course code.
> I know these stuff are difficult to follow up, don't get me wrong. But what I am suggesting is that you simply try to run your commands once every 1-2 months. I am sure that would solve half the problems people are having.
Yeah, they are... And maintaining tutorial's course code is tough fairly speaking. But that's why we wrote an internal testing system for our tutorials. And yes, we're going to run the tests constantly on our tutorials like about once per month or even more often to see that everything is still green. We just haven't gotten to this course yet to fix things properly.
We're thank you for taking your time to report it and thank you for your patience!
And please, feel free to ask in comments if you have any troubles with following the course - our team will be happy to help you along the whole course, even if you're working on newer versions of libraries!
Cheers!


- symfony/maker-bundle v1.11.6 requires doctrine/inflector ^1.2 -> found doctrine/inflector[v1.2.0, ..., 1.4.4] but the package is fixed to 2.0.4 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
Hey Robot_9000,
Could you give a bit more context please? What are you doing when seeing this error? :) Also, did you download the course code of this course, moved to a start/ directory and run "composer install" in it?
Also, did you execute "composer update" on this project at some point? It may cause problems with dependencies following this course.
Cheers!


Hi,
When creating a new entity using "bin/console make:entity" command, Symfony doesn't offer to mark the entity as an API Platform resource. Steps I took:
1) composer create-project symfony/skeleton
2) composer require api
3) composer require symfony/apache-pack (to enable /api route to work)
4) composer require maker
5) bin/console make:entity CheeseListing
Expected result: Symfony asks whether to mark entity as an API Platform resource.
Actual result: Symfony asks to add a new property.
Hey Intexsys I.!
Ah, you're totally right! There's a bug in make:entity. The behavior changes if you simply run bin/console make:entity
and then interactively answer CheeseListing
. The problem is that, if you pass the argument to the command, make:entity
incorrectly skips the interactive "Mark this class as an API Platform resource?` question. I'll open an issue about that :).
Cheers!


Hi,
I faced the same issues mentioned below and in the previous video whereby I had to specify api-platform/api-pack "1.2.0" and doctrine/doctrine-migrations-bundle "^2.0" and now I'm getting a connection refused error when running ./bin/console doctrine:database:create.
My DB URL is DATABASE_URL="postgresql://root:@127.0.0.1:5432/cheese_whiz?serverVersion=13&charset=utf8"
I'm using root with no password and mysql 5.7 so this shouldn't be an issue
`
In AbstractPostgreSQLDriver.php line 88:
An exception occurred in driver: SQLSTATE[08006] [7] could not connect to server: Connection refused
Is the server running on host "127.0.0.1" and accepting
TCP/IP connections on port 5432?
In Exception.php line 18:
SQLSTATE[08006] [7] could not connect to server: Connection refused
Is the server running on host "127.0.0.1" and accepting
TCP/IP connections on port 5432?
In PDOConnection.php line 39:
SQLSTATE[08006] [7] could not connect to server: Connection refused
Is the server running on host "127.0.0.1" and accepting
TCP/IP connections on port 5432?
`
Hey BL,
From your DATABASE_URL credentials I see you configured your project to use PostgreSql, but later you're talking you use MySQL. So, what DB do you use exactly? If it's MySQL - you should change the DATABASE_URL to use the "mysql" instead of "postgresql". Also, if you're on MySQL - you probably would beed to tweak the port as well in your config, the default MySQL port is 3306 instead of 5432. Please, make sure your DB credentials in DATABASE_URL are correct and try again.
Cheers!


Ah ha, good spot. Sorry, the postgresql line one was uncommented by default, I didn't see the mysql and sqlite options above it ;)
Thanks!


(base) shubham@Shubhams-MacBook-Air API-Platform % ./bin/console doctrine:database:create
In AbstractMySQLDriver.php line 115:
An exception occurred in driver: SQLSTATE[HY000] [2006] MySQL server has gone away
In Exception.php line 18:
SQLSTATE[HY000] [2006] MySQL server has gone away
In PDOConnection.php line 39:
SQLSTATE[HY000] [2006] MySQL server has gone away
In PDOConnection.php line 39:
PDO::__construct(): MySQL server has gone away
doctrine:database:create [--shard SHARD] [--connection [CONNECTION]] [--if-not-exists] [-h|--help] [-q|--quiet] [-v|vv|vvv|--verbose] [-V|--version] [--ansi] [--no-ansi] [-n|--no-interaction] [-e|--env ENV] [--no-debug] [--] <command>
Currently composer try to install ^3.1.
To make it work (and be consistent with finish project) I use composer require doctrine/doctrine-migrations-bundle "^2.0"
I installed migrations and then tried to create the doctrine:database create it throws this error. I restarted the mysql server and tried several times.


It worked, the port number was not 3306 idk why. so i changed it to 3306 and it worked.
Hey Shubham,
Awesome! Glad it works for you now :) And thanks for sharing your solution with others!
Cheers!
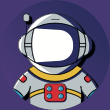
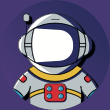
I'm working through the API Platform tutorials and have a solid start to an API. Your API tutorials are great!
Now I want to call the API I built from my Symfony web app directly. So say I have a resource with a route for /api/books (that is connected to MySQL DB) - I want to create a Symfony Controller to list the collection of books as well as perform all the CRUD for a given item. The results of the calls are displayed in my Symfony web app. So say I want to list all books, I want a Symfony Controller to call a GET for a collection of /api/books. Same with the all Items (e.g. add a new book, edit a book, update a book, delete a book, etc).
Do you have a tutorial/example for doing this, please? Only thing I seem to come by is this page on the API Platform website but it seems a bit incomplete: https://api-platform.com/do....
Thanks!
Hi @max!
Sorry for the slow reply - I had a family matter come up. But I'm really happy you're enjoying the tutorials! ❤️
Ok, let me make sure I understand correctly :). You already have an API built - with, for example, a /api/books resource. Now, you have a *separate* Symfony app where you would like to (effectively) build a Book web "CRUD" section, where a normal browser user can list, create, update, delete books... like any normal web app (with the one big difference being that instead of reading and saving from a local "book" database table, your Symfony app will be talking to the other API app). Is this accurate?
Of so, this is... for the most part, functionality that you will need to build "by hand". But I have a few pieces of advice:
A) Since creating a CRUD is a well-known thing to do with Doctrine, I would model my code off of a normal Doctrine CRUD... but I might create services (that look similar to the entity manager, for example) to do the "querying" and "saving" across the API.
B) API Platform generates an OpenAPI spec for your API. And... one of the "perks" of this, is that you can automatically generate "PHP model & client code" for your API. The docs are here: https://api-platform.com/do... - you may love this... or. maybe you don't love how the generated code looks (and it can be, in my experience, a bit of a trial-and-error to learn how to get things set up), but it's worth a try. This process would literally give you, for example, a "Book" php model class that allows you to read & set all the data from/to it and "save" it back to the API. Basically, it's like a "SDK" for your API... generated automatically.
And if I completely answered the wrong question... let me know ;).
Cheers!
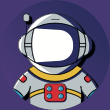
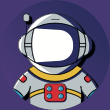
Why can't all of this be handled from api webpage instead of terminal?
Hey nocturnalProgrammer
Are you talking about the MakerBundle
commands?


Hi
I can not create the database. I get this error:
In AbstractMySQLDriver.php line 97:
An exception occurred in driver: SQLSTATE[HY000] [2002] Connection refused
In PDOConnection.php line 31:
SQLSTATE[HY000] [2002] Connection refused
In PDOConnection.php line 27:
SQLSTATE[HY000] [2002] Connection refused
I am using Windows 10 Ubuntu bash.
This is my DATABASE_URL
#DATABASE_URL=mysql://db_user:db_password@127.0.0.1:3306/db_name?serverVersion=5.7
DATABASE_URL=mysql://root:@127.0.0.1:3306/cheese_whiz?serverVersion=5.7
Hey Payman,
This sounds like you're using invalid credentials to the DB. Are you sure you can access DB locally via "root" user and without password? Btw, what OS do you use? Ubuntu? If so, I may know what's the problem... in newer versions of MySQL you can't access the DB via "root" user anymore for security reasons, I'd recommend you to create a new MySQL user with necessary privileges and use it instead for your DATABASE_URL.
Cheers!


First, how can I run a migration file for a specific schema?
When I run make:migration
, I see $schema
argument is passed but never used in the functions in migration file.public function up(Schema $schema) : void
What is the purpose of $schema
and when/how can I use it?
Second, how can I use a specific database schema in entities?
Let's say CheeseListing
table is in products
schema and User
table is in public
schema.
Thanks for your help!
Hey Sung,
First of all, that "Schema $schema" comes from the parent class, your migrations should match method signature of the parent up() method. Doctrine migrations give you that object so you could do custom things easily. To know what you can do - see "Doctrine\DBAL\Schema\Schema" class for more reference or just use IDE autocompletion on $schema arg. It has some shortcuts like renameTable(), dropTable(), and much more.
But since we usually "generate" migrations automatically thanks to the Doctrine migration - it generates raw SQL queries and you can see those $this->addSql() calls - it's just simpler for the library to generate those. And most of the time you just need to make sure those already generated queries looks good. But if you need to do some custom operations in you migration file - you can totally use that $schema object.
> Second, how can I use a specific database schema in entities?
> Let's say CheeseListing table is in products schema and User table is in public schema.
I'm not sure I understand this question fairly speaking. If we're talking about SQL - we have a database that holds tables which in turn contain columns, right? Are you talking about storing "CheeseListing" table in one database e.g. "products" and "User" table in another database called e.g. "public"? Am I understand it correct?
Cheers!


Hi
I'm having a problem when creating the first migration.
It returns this: An option named "connection" already exists.
My database has been created, and it's visible in Workbench. I have tried deleting everything and starting over, clearing the cache etc. but the problem prevails.
Google gets me nowhere, so it's hard to proceed from here.
Any tips would be greatly appreciated!
Hey C!
Hmm, this is odd. The error is coming from Symfony's console - it's a situation where a command tries to add an option (in this case "connection") two times... which just simply should not happen. It makes me wonder if - somehow (though this shouldn't be allowed) you have versions of doctrine/migrations and doctrine/doctrine-migrations-bundle that are conflicting with each other.
Try this: run composer show
. What versions do you see for all the doctrine/ libraries?
To try to fix it, you could try to composer remove doctrine/doctrine-migrations-bundle doctrine/migrations
and then re-install them... but that's just a guess. Btw, are you using the code download from this course or on a custom project?
Cheers!


Hi weaverryan
I'm using the code download from this course.
It looks like you sorta got it right with the conflicting versions of doctrine/doctrine-migrations-bundle and doctrine/migrations.
The versions were/are as follows:<br />doctrine/doctrine-migrations-bundle 3.0.1<br />doctrine/migrations 3.0.1<br />
But removing them and installing them again solved the problem even if the version numbers didn't change.
Thanks!!


Hi!
I'm running this code on Ubuntu and on the point of
<blockquote> composer require migrations</blockquote>
I get the following error:
`
Using version ^3.0 for doctrine/doctrine-migrations-bundle
./composer.json has been updated
Loading composer repositories with package information
Updating dependencies (including require-dev)
Restricting packages listed in "symfony/symfony" to "4.2.*"
Your requirements could not be resolved to an installable set of packages.
Problem 1
- doctrine/doctrine-migrations-bundle 3.0.0 requires doctrine/migrations dev-master -> satisfiable by doctrine/migrations[dev-master] but these conflict with your requirements or minimum-stability.
- Installation request for doctrine/doctrine-migrations-bundle ^3.0 -> satisfiable by doctrine/doctrine-migrations-bundle[3.0.0, 3.0.1].
- Conclusion: remove doctrine/migrations 2.2.1
- Conclusion: don't install doctrine/migrations 2.2.1
- doctrine/doctrine-migrations-bundle 3.0.1 requires doctrine/migrations ~3.0 -> satisfiable by doctrine/migrations[3.0.0, 3.0.1].
- Can only install one of: doctrine/migrations[3.0.0, 2.2.1].
- Can only install one of: doctrine/migrations[3.0.1, 2.2.1].
- Installation request for doctrine/migrations (locked at 2.2.1) -> satisfiable by doctrine/migrations[2.2.1].
Installation failed, reverting ./composer.json to its original content.
`
I've tried installing other versions as suggested in the output, but none of that helped.
I hope someone is able to help me out here?
Hey Michel!
I think I know this error :). The Doctrine organization has recently been releasing some major versions of libraries, and it's been causing various problems like this. The problem isn't really what Doctrine did, but that bumping new major versions makes it possible to have error like this.
Check out this line:
Installation request for doctrine/migrations (locked at 2.2.1)
This makes it sound like you actually already have doctrine/migrations (the library, not the bundle) installed in your app. Is that true? You can see by running composer up doctrine/migrations
.
Basically, the problem is that doctrine/doctrine-migrations-bundle 3 is now out which requires doctrine/migrations 3. But apparently, your app already has v2. Instead of upgrading doctrine/migrations from 2 to 3 (which you may not want), it gives you this error.
There are a few solutions:
1) You could install v2 of the bundle: composer require doctrine/doctrine-migrations-bundle:^2
. This only requires v2 of doctrine/migrations
2) Or, you could upgrade doctrine/migrations. This may or may not be easy :). What I mean is, if you see this in your composer.json file, then you will need to change the version constraint to ^3.0
. But if it is NOT in that file, then it's being required by another library... and that library may or may not allow v3. In either situation, see if the upgrade works by running composer up doctrine/migrations
. If that works, then you can re-install v3 of the bundle.
Let me know if that helps!
Cheers!


Thanks for the reply weaverryan
Your suggestions did not solve it for me, so in the end I decided to start from scratch again.
And at that point I noticed what might have caused the issue for me in the first place! I had been working in the 'finish' folder instead of the 'start' folder.
Just leaving this here for any future course followers, in case they made that same mistake and hadn't noticed it either.
Woo! Happy that you got it worked out one way or another Michel - and thanks for sharing your solution :).
Cheers!


I get this error from composer require migrations:
`Your requirements could not be resolved to an installable set of packages.
Problem 1
- Installation request for doctrine/doctrine-migrations-bundle ^3.0 -> satisfiable by doctrine/doctrine-migrations-bundle[3.0.0, 3.0.1].
- Can only install one of: psr/log[1.1.3, 1.1.0].
- Can only install one of: psr/log[1.1.3, 1.1.0].
- Can only install one of: psr/log[1.1.3, 1.1.0].
- Conclusion: install psr/log 1.1.3
- Installation request for psr/log (locked at 1.1.0) -> satisfiable by psr/log[1.1.0].
Installation failed, reverting ./composer.json to its original content.`
Hey Eduard
Sorry for the delay but you can just upgrade the psr/log
library and it should work. Anyway, if you download the tutorial's code you won't get that error anymore.
Cheers!
Hey Ecludio!
Looks like the latest version of doctrine/doctrine-migrations-bundle that is 3.x does not work with your project. You can try to install the lower version, e.g. run:
$ composer require 'migrations:^2.0'
It should help, this will install the latest version of 2.x, and this is the version we're using in the course code. Otherwise, if you really want the 3rd version - I suppose you would need to update your dependencies first.
Cheers!
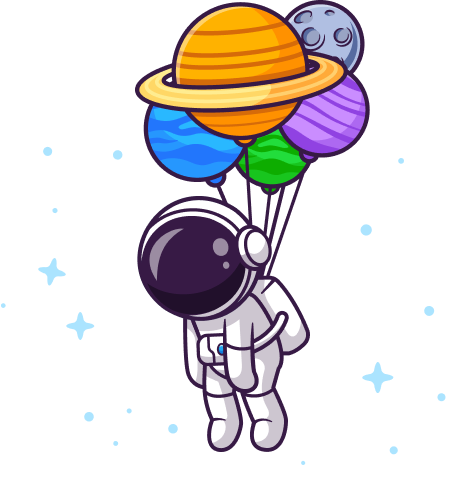
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.1.3",
"ext-ctype": "*",
"ext-iconv": "*",
"api-platform/core": "^2.1", // v2.4.3
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/annotations": "^1.0", // 1.10.2
"doctrine/doctrine-bundle": "^1.6", // 1.11.2
"doctrine/doctrine-migrations-bundle": "^2.0", // v2.0.0
"doctrine/orm": "^2.4.5", // v2.7.2
"nelmio/cors-bundle": "^1.5", // 1.5.5
"nesbot/carbon": "^2.17", // 2.19.2
"phpdocumentor/reflection-docblock": "^3.0 || ^4.0", // 4.3.1
"symfony/asset": "4.2.*|4.3.*|4.4.*", // v4.3.11
"symfony/console": "4.2.*", // v4.2.12
"symfony/dotenv": "4.2.*", // v4.2.12
"symfony/expression-language": "4.2.*|4.3.*|4.4.*", // v4.3.11
"symfony/flex": "^1.1", // v1.21.6
"symfony/framework-bundle": "4.2.*", // v4.2.12
"symfony/security-bundle": "4.2.*|4.3.*", // v4.3.3
"symfony/twig-bundle": "4.2.*|4.3.*", // v4.2.12
"symfony/validator": "4.2.*|4.3.*", // v4.3.11
"symfony/yaml": "4.2.*" // v4.2.12
},
"require-dev": {
"symfony/maker-bundle": "^1.11", // v1.11.6
"symfony/stopwatch": "4.2.*|4.3.*", // v4.2.9
"symfony/web-profiler-bundle": "4.2.*|4.3.*" // v4.2.9
}
}
This course should be marked as outdated for real. I simply cannot replicate steps you are doing. I am facing error literally everytime I am trying to do something.