This course is archived!
This tutorial uses a deprecated micro-framework called Silex. The fundamentals of REST are still valid, but the code we use can't be used in a real application.
RESTful APIs in the Real World Course 1
Unearth the principles of RESTful APIs, from HTTP basics to proper status codes, and effectively test your API.
About this course
Making RESTful APIs is hard, really hard. There are a lot of concepts to know - resources, representations, HTTP methods, status codes, etc.
And putting this all to life in a sane way in PHP is no small task. In this course, we'll learn all the most fundamental concepts around REST and learn about the "rules" and the advantages and disadvantages of each. And we'll of course build a real API to show it all off. Topics include:
- HTTP basics
- Resources and Representations
- Resource state, client state
- GET, POST, PUT, PATCH, DELETE
- Idemptotency and safe methods
- Proper Status Codes, Location header and Content-Type
- Testing your API
- RFCs and where the "rules" come from
- Advanced error and validation handling
If you want to know about Hypermedia, HATEOAS, Content-Type negotiation, pagination and more, these are not covered here, but are covered in Episode 2.
This tutorial uses Silex and we avoid talking about it as much as possible and instead focus on the core concepts of REST. Once you understand these, you can watch our Symfony REST series to learn how to leverage Symfony for your API.
Next courses in the APIs: Beginner: Learn REST Fundamentals section of the APIs Track!
106 Comments
You're right! I've just updated the code download - it *does* contain a few more things than the original beta code download.
Thanks for pointing that out!
Most of the chapters are recorded and edited, so it should be very soon now! We're passed due on getting this out and we know it - so we're working our butts off this week on it to not delay further :).
Cheers!
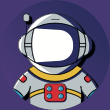
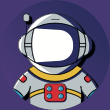
Excellent so far! I've purchased and already seen it all, so great job! Waiting to see the rest of it. It would be great if you cover how to handle different formats for same url endpoints, just like FOSRestController do, and not to have the code duplicate in API Controllers and so on.
Cheers!
Yes, that's not an easy problem to solve! We'll definitely cover that, using an approach that you'll recognize from FOSRestController. There are still a lot of tough details to cover in episode 2!
Really happy you've enjoyed it!


Hi I see a link to Episode 3 but redirects to home page.
Quote:
If you want to know about Hypermedia, HATEOAS, Documenting your API,
Content-Type negotiation, pagination and more, these are not covered
here, but will be covered in Episode 2 and Episode 3.
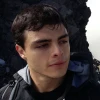
Hey Pierpaolo D.
Dang, that's our bad, that epidose doesn't exit yet and I can't tell if it will exist any time soon. Sorry about it
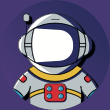
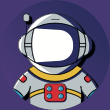
Hi, I'm excited for the second part of the RESTful API tutorial.
If possible I want to know which is the more efficient or preferred way of implementing the api and web interface.. separate controllers or only one but be able to handle different representations?
Hi Jasper!
Yea, that's quite controversial - even William and I disagree on this :). William (who I admit is more knowledgeable than I am) recommends using a single controller for both the HTML and JSON (or XML) representations. The potential problem with this approach might be if your web interface doesn't really look like your API. For example, you might have a /products API endpoint. But perhaps there's really no "products list" web interface page - perhaps instead there is /dashboard only, which shows products and users you're connected to (just imagining some example).
I would say, if you're comfortable with the idea of using one controller to return different representations, try that. But realize, that you may have some controllers that will only return JSON or will only return HTML, because there is only a JSON version of /products and an HTML version of /dashboard. And I think that's ok :). If you're overwhelmed by all of this, then don't feel bad using different controllers (but do your best to use services to avoid duplication).
Great question!
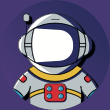
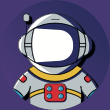
Any ETA? :) Too late for me to take advantage (I think i have a pretty good grasp now anyway) but I am looking forward to these anyway.
Hey! No hard ETA yet - but we're aiming to start releasing chapters in the next couple of weeks (I'm working on stuff right now).
Cheers!
hi,Ryan, Some times, i want get collection first,then sort by custom condition( not property), how to do that
Hey @Yangzhi!
Are you using API Platform? Or just creating a RESTful endpoint on your own? Let me know, and I'll see if I can help :). Doing what you want can be less efficient on large collections but, in general, yea, it should be possible and not a huge deal.
Cheers!
yes, i use api platform,i konw how sort by property,but if i want sort by custom condition,how to do that,thanks
Hey @Yangzhi!
Sorry for the slow reply :). Hmm. Ideally, you could translate this "custom condition" into a query. Then, you would create a custom API Platform filter - https://symfonycasts.com/screencast/api-platform-extending/entity-filter-logic - and modify the query. This is really the best way, because it works with pagination.
If you need to make the query first and then filter in PHP, probably the best option is to "decorate" the CollectionProvider
that your collection endpoint uses in Api Platform 3. We do something a bit similar with a "state processor" - https://symfonycasts.com/screencast/api-platform-security/state-processor (API platform also has docs on this - they do it a slightly different way - but both are great https://api-platform.com/docs/core/state-providers/#hooking-into-the-built-in-state-provider)
The idea is that you would call the inner, decorated CollectionProvider
and allow it to make the query like normal. You would then filter that in PHP and return the results. You could create an actual custom filter class if you want - kind of like https://symfonycasts.com/screencast/api-platform-extending/custom-resource-filter - so that the filter shows up in your documentation.
This will likely not play well with your pagination or "count", however. What I mean is: your API may say that it has 50 items, but really you can only find 35. That's because it gets the 50 count from the original query results (I believe)... but then you're removing some afterwards.
Good luck!
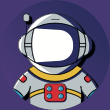
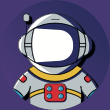
Hello Ryan,
Is this course updated in order to meet symfony 4? Is it still relevant?
Ty
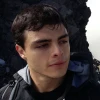
Hey dianikol
This course was made on Symfony2 but its content is still relevant speaking about REST API's, but of course, you will have to adjust some blocks of code in order to work with Symfony4
Cheers!
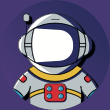
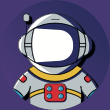
Can you tell us when approximately this screencast will be available? I'm looking forward to it!
Hey Michal!
We're aiming at Feb to start releasing this.
Cheers!
We didn't hit Feb, but I just updated the release date information (above in the summary) - I'm working hard on this - we'll post things as soon as we can :) Cheers!
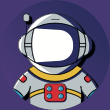
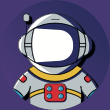
Hey Ryan - any chance of an update on the timings on this one? keen to see it.
Hey Chris!
We just released the first chapters this morning and a full chapter list. Hope you enjoy it!
Cheers!
Hey Tor!
A few chapters should come out next week (good REST background, building up the basic endpoints, testing them, etc) with more of the meat coming a few weeks later. We'll email you with updates when the first chapters come out.
Cheers!
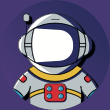
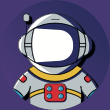
Ok, thanks for letting me know, and for working on the tutorials. I'm looking very much forward to seeing them released!
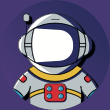
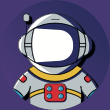
Hey Ryan!
Just finished this episode, learned a lot about the idea behind REST, HTTP methods and status codes! :-)
However I still have some open questions. I am now working on a web service which should accept PUT requests from other domains (in this case sent from an iOS app). Up until now we are building the request using an AJAX call and JSONP, which turns out not to be true REST and has well-known security issues (so I am not happy at all with it).
I was just wondering if there is something related to this topic coming in episode 2 of these series, maybe related with Cross Domain Headers and so on... That would be very helpful!
I look forward to it and keep up the nice work!
Hi Miriam!
Hmm, I haven't gotten this far in the next tutorial, but we *do* have CORS on the menu for it, which indeed could be very helpful in your case. Instead of waiting for us, my advice would be to go ahead and check into this. If you happen to use Symfony, there is a bundle that helps with this: https://github.com/nelmio/N.... And if not, the bundle may still be instructive.
Cheers!
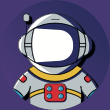
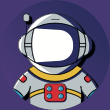
Hi Ryan! thanks for the quick reply :-) I will look definitely into it. And yeap, I do use Symfony for it! Cheers!
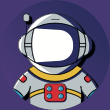
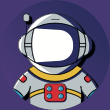
I had a little trouble initially setting up, but a few things that may help others, in case you are as n00b as me:
sql lite requires ownership for me so i did: $ sudo chgrp _www -R data
also, I'm on php 5.3 to match my work servers, so I had to make adjustment to line 70 from [] to array()
src/KnpU/CodeBattle/Controller/UserController.php
return $this->render('user\register.twig', array('errors' => $errors, 'user' => $user));
Thanks buddy - I've made an issue (https://github.com/knpunive... to get rid of the share array syntax They're cute, but not necessary.
Cheers!
Hey Davor!
It means that part of the tutorial is available, but part of it still needs to be released. Right now, if you look at the table of contents on the left, you'll see the last few chapters are grey - we're still working on those and will release them soon. So, you can start going through the tutorial now, and if you own it, we'll email you when the rest of the chapters come out.
Cheers!
Hey Wojtek!
I'm not sure yet. We have an OAuth tutorial coming out very shortly (http://knpuniversity.com/sc..., which might address some of this. Which parts of authorization with a REST API would be helpful to you?
Thanks!
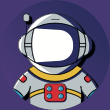
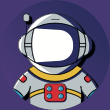
I'd like to see HTTP basic, HTTP digest and OAuth2.
Also are you planning to show how to implement HATEOAS (Hypermedia)?
Definitely will be covering Hypermedia, that's where things get interesting :). I'll have at least some details in there on authentication as well - I understand very well why you're asking!
Thanks for the extra details!
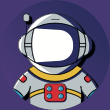
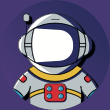
I am also extremely curious when this is going to be done. I could use this information ASAP :)
This will be a Feb release! Sorry it can't be sooner - but if you click "Notify Me" - we'll ping you as soon as the first chapters are published.
Cheers!
FYI - I've just updated the release date information, so not by Feb obviously, but soon! Thanks!
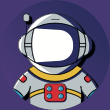
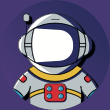
Where part with authorization via REST? I already did REST before this tutorial has been published, however authorization part still didn't chosen. WSSE, Token or your ideas?
Hey Aliaksandr Harbunou!
Obviously, it depends on what you need, but I'm a fan of using a token system: it's simple to implement and very understandable by your clients. That's what we're using in episode 2, modeled roughly off of GitHub. If you're curious, you can see the low-level setup here: https://github.com/knpunive....
Hope that helps!
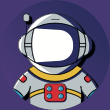
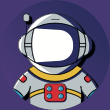
Hi, sounds very intresting! Can't wait! Will be this REST API course connected with SF2 ?
Almost definitely :). Though I haven't decided if we should do something more generic first to introduce the concepts directly (like in Silex) and *then* something in Symfony2 using our extra tools. I'd love to have something useful for Sf2 and non-Sf2 devs.
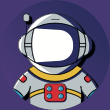
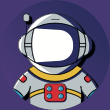
Hmmm i think it should be everything with Sf2 for Sf2 devs! :D Almost exactly one year before i started with Sf2 and i was like :O and it was the KNP course that had introduced me for the first time the world of frameworks and the beauty of this framework. Now i desperately crave for some great Sf2 REST course that will learn me a lot and will show practical tricks :) So once again, can't wait! Very excited :)
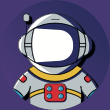
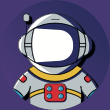
Just finished it up - really can't wait for the next course. Great job everyone! :)
If anyone wants more reading on this, Phil Sturgeon's "Build APIs You Won't Hate" (available via Leanpub) is a great resource to learn more. It covers topics that will be in the second course, but it's available now.
I'll be back to watch the second course when it comes out :D
Thanks so much! I've also read about half of Phil's book (just been short on time) and very much enjoyed it as well.
We'll keep pushing forward with the 2nd episode - I'm excited too!
Cheers!
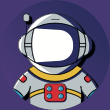
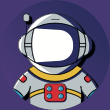
Should there also be a topic related to a client that can consume the api in the right way?
Hi Ryan,
the source code is missing the ApiFeatureContext::thePropertyShouldContain method (that was not defined in the previous beta code).