Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: ^7.1.3
Subscribe to download the code!Compatible PHP versions: ^7.1.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Installing Doctrine
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Friends! Welcome back to the third episode in our starting in Symfony 4 series! We've done some really cool stuff already, but it's time to explore deeper in an epic search for intelligent life... and also.. and database! Yea - what good is our cool interstellar space news site... without being able to insert and query for data?
But... actually... Symfony does not have a database layer. Nope, for this challenge, we're going to rely one of Symfony's BFF's: an external library called Doctrine. Doctrine has great integration with Symfony and is crazy powerful. It also has a reputation for being a little bit hard to learn. But, a lot has improved over the last few years.
Code with Me!
If you also want to be best-friends-forever with Doctrine, you should totally code along with me. Download the course code from this page. When you unzip it, you'll find a start/
directory that has the same code that you see here. Open the README.md
file for details on how to get the project setup... and of course, a space poem.
The last step will be to open a terminal, move into the project and run:
php bin/console server:run
to start the built in web server. Then, float over to your browser, and open http://localhost:8000
to discover... The Space Bar! Our inter-planetary, and extraterrestrial news site that spreads light on dark matters everywhere.
In the first two episodes, we already added some pretty cool stuff! But, these articles are still just hard-coded. Time to change that.
Installing Doctrine
Because Doctrine is an external library, before we do anything else, we need to install it! Thanks to Symfony flex, this is super easy. Open a new terminal tab and just run:
composer require doctrine
This will download a "pack" that contains a few libraries, including doctrine itself and also a migrations library to help manage database changes on production. More on that soon.
And... done! Hey! That's a nice message. Because we're going to be talking to a database, obviously, we will need to configure our database details somewhere. The message tells us that - no surprise - this is done in the .env
file.
Configuring the Database Connection
Move over to your code and open the .env
file. Nice! The DoctrineBundle recipe added a new DATABASE_URL
environment variable:
Show Lines
|
// ... lines 1 - 15 |
###> doctrine/doctrine-bundle ### | |
# Format described at http://docs.doctrine-project.org/projects/doctrine-dbal/en/latest/reference/configuration.html#connecting-using-a-url | |
# For an SQLite database, use: "sqlite:///%kernel.project_dir%/var/data.db" | |
# Configure your db driver and server_version in config/packages/doctrine.yaml | |
DATABASE_URL=mysql://db_user:db_password@127.0.0.1:3306/db_name | |
###< doctrine/doctrine-bundle ### |
Let's set this up: I use a root
user with no password locally. Call the database the_spacebar
:
Show Lines
|
// ... lines 1 - 15 |
###> doctrine/doctrine-bundle ### | |
# Format described at http://docs.doctrine-project.org/projects/doctrine-dbal/en/latest/reference/configuration.html#connecting-using-a-url | |
# For an SQLite database, use: "sqlite:///%kernel.project_dir%/var/data.db" | |
# Configure your db driver and server_version in config/packages/doctrine.yaml | |
DATABASE_URL=mysql://root:@127.0.0.1:3306/symfony4_space_bar | |
###< doctrine/doctrine-bundle ### |
Of course, this sets a DATABASE_URL
environment variable. And it is used in a new config/packages/doctrine.yaml
file that was installed by the recipe. If you scroll down a bit... you can see the environment variable being used:
parameters: | |
# Adds a fallback DATABASE_URL if the env var is not set. | |
# This allows you to run cache:warmup even if your | |
# environment variables are not available yet. | |
# You should not need to change this value. | |
env(DATABASE_URL): '' | |
doctrine: | |
dbal: | |
Show Lines
|
// ... lines 10 - 17 |
# With Symfony 3.3, remove the `resolve:` prefix | |
url: '%env(resolve:DATABASE_URL)%' | |
Show Lines
|
// ... lines 20 - 31 |
There are actually a lot of options in here, but you probably won't need to change any of them. These give you nice defaults, like using UTF8 tables:
Show Lines
|
// ... lines 1 - 7 |
doctrine: | |
dbal: | |
Show Lines
|
// ... lines 10 - 12 |
charset: utf8mb4 | |
Show Lines
|
// ... lines 14 - 31 |
Or, consistently using underscores for table and column names:
Show Lines
|
// ... lines 1 - 7 |
doctrine: | |
Show Lines
|
// ... lines 9 - 19 |
orm: | |
Show Lines
|
// ... line 21 |
naming_strategy: doctrine.orm.naming_strategy.underscore | |
Show Lines
|
// ... lines 23 - 31 |
If you want to use something other than MySQL, you can easily change that. Oh, and you should set your server_version
to the server version of MySQL that you're using on production:
Show Lines
|
// ... lines 1 - 7 |
doctrine: | |
dbal: | |
# configure these for your database server | |
driver: 'pdo_mysql' | |
server_version: '5.7' | |
Show Lines
|
// ... lines 13 - 31 |
This helps Doctrine with a few subtle, version-specific changes.
Creating the Database
And... yea! With one composer require
command and one line of config, we're setup! Doctrine can even create the database for you. Go back to your terminal and run
php bin/console doctrine:database:create
Say hello to your new database! Well, it's not that interesting: it's completely empty.
So let's add a table, by creating an entity class.
77 Comments
Hey Mike,
Thank you for sharing it for our MariaDB users!
Cheers!


I use mysql 8.0 and I keep getting this error:
"php bin/console doctrine:database:create
2018-09-28T01:27:27+00:00 [error] Error thrown while running command "doctrine:database:create". Message: "An exception occurred in driver: SQLSTATE[HY000] [2054] The server requested authentication method unknown to the client""
For me is work only if I do this 3 steps:
1. I add this two lines to my.cnf
default_authentication_plugin=mysql_native_password
validate_password.policy=LOW
2. restart mysql server
3. Create a new user/password and change it in .env file
Without the step 3, the root user is keeping the Authentication Type: "caching_sha2_password"
After create a new user, then this user will use Authentication Type: "mysql_native_password"
PS
This all is because of new Authentication Type in MySql 8.0.13 Server
but will be supported in a future PHP release by default.
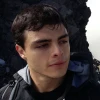
Thanks for sharing your solution!


Figured it out. I had to run this query:
ALTER USER 'root'@'localhost' IDENTIFIED WITH mysql_native_password BY '';


Hello there,
I try to install doctrine and I got the following error:
<blockquote>Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 1
!!
!! In EnvVarProcessor.php line 76:
!!
!! Environment variable not found: "DATABASE_URL".
!!
!!
!!
Script @auto-scripts was called via post-update-cmd
Installation failed, reverting ./composer.json to its original content.
exit status 1</blockquote>
This was my steps:
- I downloaded the course code
- Unzip the file
- Go to terminal
cd start
symfony composer install
symfony composer require doctrine
Hey Ngan-Cat,
Yes, you need to add that DATABASE_URL to your .env.dist file. The Symfony Flex should do this itself, not sure why it won't added for you. I just tried to repeat your steps and I see "DATABASE_URL=mysql://db_user:db_password@127.0.0.1:3306/db_name?serverVersion=5.7" line in my .env.dist file. Probably you denied to execute Symfony Flex recipes? Anyway, just add this line default line to your .env.dist and the error will be gone :)
Cheers!


C'mon Ryan!?$%@# This webcast is suppose to be for noobs like me. A very important detail was left out of the first chapter which is that MYSQL has to already be installed and running. I spent 2 days trying to figure this out. If it's not installed you will get 2002 - connection refused error. And since this was such a basic and fundamental misstep there is no advice on the web to help fix it.
Hey Andre V.!
Sorry for the SUPER slow reply - your comment got stuck in a "pending" state for some reason and we missed it!
Also, sorry about the problem - you're 100% correct! While this isn't a tutorial about getting MySql installed, I don't even mention it - which I *absolutely* should. My apologies for your making you lose time on this - but it's really good feedback. We'll be recording the Symfony 5 Doctrine tutorial soon and I'll make sure to do a better job there.
Cheers!


Hi. I try to install orm-bundle in symfony 5 but all the time I have this error:
!! In Configuration.php line 304:<br />!!<br />!! The doctrine/orm package is required when the doctrine.orm config is set.<br />
My composer pack:
`
{
"name": "symfony/skeleton",
"type": "project",
"license": "MIT",
"description": "A minimal Symfony project recommended to create bare bones applications",
"require": {
"php": "^7.2.5",
"ext-ctype": "*",
"ext-iconv": "*",
"sensio/framework-extra-bundle": "^5.1",
"symfony/asset": "5.0.*",
"symfony/console": "5.0.*",
"symfony/dotenv": "5.0.*",
"symfony/expression-language": "5.0.*",
"symfony/flex": "^1.3.1",
"symfony/form": "5.0.*",
"symfony/framework-bundle": "5.0.*",
"symfony/http-client": "5.0.*",
"symfony/intl": "5.0.*",
"symfony/mailer": "5.0.*",
"symfony/monolog-bundle": "^3.1",
"symfony/notifier": "5.0.*",
"symfony/orm-pack": "*",
"symfony/process": "5.0.*",
"symfony/security-bundle": "5.0.*",
"symfony/serializer-pack": "*",
"symfony/string": "5.0.*",
"symfony/translation": "5.0.*",
"symfony/twig-pack": "*",
"symfony/validator": "5.0.*",
"symfony/web-link": "5.0.*",
"symfony/webpack-encore-bundle": "^1.7",
"symfony/yaml": "5.0.*"
},
"require-dev": {
"symfony/debug-pack": "*",
"symfony/maker-bundle": "^1.0",
"symfony/profiler-pack": "*",
"symfony/test-pack": "*"
},
"config": {
"preferred-install": {
"*": "dist"
},
"sort-packages": true
},
"autoload": {
"psr-4": {
"App\\": "src/"
}
},
"autoload-dev": {
"psr-4": {
"App\\Tests\\": "tests/"
}
},
"replace": {
"paragonie/random_compat": "2.*",
"symfony/polyfill-ctype": "*",
"symfony/polyfill-iconv": "*",
"symfony/polyfill-php72": "*",
"symfony/polyfill-php71": "*",
"symfony/polyfill-php70": "*",
"symfony/polyfill-php56": "*"
},
"scripts": {
"auto-scripts": {
"cache:clear": "symfony-cmd",
"assets:install %PUBLIC_DIR%": "symfony-cmd"
},
"post-install-cmd": [
"@auto-scripts"
],
"post-update-cmd": [
"@auto-scripts"
]
},
"conflict": {
"symfony/symfony": "*"
},
"extra": {
"symfony": {
"allow-contrib": false,
"require": "5.0.*"
}
}
}
`
What is wrong what else shall I add?
Thank you.
Hi Dominik!
I wanted to apologize for never replying to this! Your comment somehow got stuck as "pending" and we missed it :/. If you're still having issues, let me know. It definitely looks weird as symfony/orm-pack
requires doctrine/orm
so that package absolutely should already be installed.
Cheers!


I'm using symfony 5 and docker and seem to keep getting this error:
SQLSTATE[HY000] [2002] Connection refused
What might be causing this?
Hey @Lars!
I think I know this error. This usually that your database connection information is wrong. It could be that you don't have a database running at all - or that the host name / IP address in the DATABASE_DSN is incorrect? Because you're using Docker, my guess is that either:
A) You don't have the php container networked correctly to the database container
OR
B) You DO have it linked correctly, but you need to update DATABASE_DSN to point to whatever host name the database container is exposed on.
Let me know if this helps!
Cheers!


Hello!
I got this error after php bin/console doctrine:database:create
In MariaDb1027Platform.php line 12:
Attempted to load class "MySqlPlatform" from namespace "Doctrine\DBAL\Platforms".
Did you forget a "use" statement for another namespace?
Can someone help me?
Hey Rafael,
Hm, interesting... Could you do "rm -rf vendor/" and then "composer install"? Does it helps? If not, probably try to upgrade the library with "composer update doctrine/dbal".
If you still have this error, could you tell us your doctrine/dbal version installed? You can do it with "composer info doctrine/dbal". And did you download the course code from SymfonyCasts? In what directory are you: start/ or finish/ ?
Cheers!


Hello there,
When building a project from scratch, what is a preferred way - to design and create database first then generate entity classes (https://symfony.com/doc/cur... or create entity first then migrate it into database?
Thank you!
Hey Dung,
Good question! Definitely you need to create an entity first, and then let Doctrine to do the low level set up for you. The reverse is useful for legacy projects only, when you have a DB already and want to migrate your project to Doctrine base on your table.
Cheers!


Thank you!
Can you Actually update the code so it matches Symfony 4, The Slack bundle is not useable with S4 and LTS is abondoned. Keep getting errors like
`Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 1
!!
!! In ContainerBuilder.php line 1055:
!! Circular reference detected for service "nexy_slack.client", path: "nexy_sl
!! ack.client -> Nexy\Slack\Client -> nexy_slack.client".
<br />and <br />
Circular reference detected for service "nexy_slack.client", path: "nexy_slack.client -> Nexy\Slack\Client -> nexy_slack.client".
`
Hey @jp!
Hmm. Are you downloading the course code and getting this error? Or installing the Slack library from the previous tutorial (https://symfonycasts.com/sc... Let me know!
Cheers!


Please advise, same problem with me. I use the downloaded course code.
Hey Muslax,
In what directory of downloaded code you are: start/ or finish/? Btw, did you run "composer install" or "composer update" to bootstrap the project locally?
Cheers!


Same here, with
composer install
or
composer update
.Tried to remove vendor dir. I was doing courses one by one, reached "Symfony Security: Beautiful Authentication, Powerful Authorization", paused for 3-4 weeks, then tried to run `composer update`. Works when I commented `Nexy\Slack\Client: '@nexy_slack.client'` in services.yaml
Hey John!
I did some research on this and found out the problem. The issue is with nexylan/slack-bundle
2.2. They... did something a little silly... which broke things slightly :) ()https://github.com/nexylan/slack-bundle/pull/31/files#r334506538 However, you should not see the issue if you're using the same version of the bundle that ships with the code-download (which is 2.0). So basically, there are two ways to solve this :)
1) Just download the starting code from this tutorial and run composer install
. This will download version 2.0 of the bundle, which works just fine.
2) Or, if you did run a composer update
then (as you mentioned), you can comment-out this line in config/services.yaml
:
services:
# ...
# you can commnent this line out with NexySlackBundle 2.2, as this alias is already added by the bundle
# Nexy\Slack\Client: '@nexy_slack.client'
Let me know if this helps!
Cheers!


HI Im just being curious about how can i subscribe this video to download the course code..
Hey Su,
To download the course code - you need to be a paid subscriber (subscribe to our monthly or yearly subscription plan) or course owner (buy access to the exact this course only). Unfortunately, we do not provide partial access to separate videos only, you need to buy access to the entire course at least.
For your information, you can see "Buy Access to Course" above this video. Also, we always make a few first videos free, so you just need to go to further chapters to see the link how to buy the course instead of the video, e.g: https://symfonycasts.com/sc... . Or, just go to the course overview page: https://symfonycasts.com/sc... :)
To recap, if you want to get access to this course - here's the direct link to possible options:
https://symfonycasts.com/pr...
Cheers!

Hello Kenan here again, I want to share what happens in both Windows 10 x64 and Fedora 30
When I tried to run:
composer require doctrine
I got the following:
<blockquote>Installation failed, reverting ./composer.json to its original content.
[Composer\Downloader\TransportException]
The 'http://repo.packagist.org/p/ocramius/proxy-manager$23f10761724afd14523e6f6216bc5f5ee06873c35af1fdcb6c53a6f5c3ef05a8.json' URL could not be accessed: HTTP/1.1 400 Bad URI </blockquote>
After tons of searching entire web I found the solution by adding this to composer.json:
`"repositories": [
{
"type": "composer",
"url": "https://packagist.org"
},
{
"packagist": false
}
]`
I need to do that just once in any project.
So why that happened ?
Yo Kenan!
Well, I'm not sure about *your* fix, but I can explain what happened :) The problem is that with composer you should require packages in format: "vendor-name/package-name", i.e. separate vendor name and package name with "/". So, if you want to install Doctrine ORM pack for Symfony - you should execute *this* command:
$ composer require symfony/orm-pack
But! Symfony has a special package called Flex that is Composer plugin which has such feature as package aliases, you can check them here: https://flex.symfony.com/ - where you can see that "symfony/orm-pack" has a few aliases like "doctrine", "orm", etc. It means, that if you have that Flex installed in your project, you can require packages by aliases instead of typing the full package name.
The error you see means that you don't have Flex installed in your project. So, if you do the next first:
$ composer require symfony/flex
Then you would be able to execute this:
$ composer require doctrine
without that error.
Cheers!


Hello,
i am using mysql v.5.7 with dbal v.2.5 even if the is server_version: '5.7' but i am still unable to create the database with the command
[Doctrine\DBAL\Exception\ConnectionException]
An exception occurred in driver: SQLSTATE[HY000] [1049] Unknown database 'symfony'
Hey Ali
What command do you run? :) Have you tried:
$ bin/console doctrine:database:create
If so, what's the output of this command for you?
Please, don't confuse this command with another one that creates DB schema:
$ bin/console doctrine:schema:create
Cheers!


Hey Victor,
thanks you for you answer i am using bin/console d:d:c but its not working at all in my app but i tried to install a clean symfony 3.4 on my pc and the cmmand worked so i guess somthing is wrong wth my setting.
if i creates the DB schema myself and drop it using d:d:d --force and recreate it again with the create command it does work so do you have any hint what is causing the problem ?
Thanks in advance
Hey Ali,
> if i creates the DB schema myself and drop it using d:d:d --force and recreate it again with the create command it does work
So, does it work for you now? It looks like you were able to recreate the DB schema after dropping it with "d:d:d --force" command, right?
Cheers!


What if I wanted to change the database connection after the application has started?
I have a project here where I will have several databases (or schemas), and I need to switch between each one taking into consideration the user that was logged in (in another symfony application with a static database connection to this "central" database).
I thought about using a subscriber for KernelRequest. But how would I make the connection of doctrine dynamically? And will each user really be totally isolated from others? Thanks!!
Hey Carlos,
Hm, I think you need to configure a few database connections and Entity Managers in your case, see this article in the docs for more information: https://symfony.com/doc/cur... . Then you'll just need to implement a custom logic that will decide what entity manager to use and that's it.
I hope this helps!
Cheers!


Hi Victor. Thank you, but in this case I would have only a fixed number of connections. What I'm intending to do is to have a completely dynamic structure, where clients could sign up and have I fresh new database. I don't know how much databases I would have at first. Thanks.
Hey Carlos,
Hm, I see, that's a tough question.
> And will each user really be totally isolated from others?
I don't think it's possible, though I'm not exactly sure, have never tried something similar before. You probably could look into direction of Symfony Voters or ACL where you will check if the current user is allowed to perform the action. But anyway, it might be tricky to check the actual query. I think to make every user really isolated - you need to create a separate DB for each user along with MySQL user with it's own credentials, and assign the MySQL user to a Symfony user so you could check if user is allowed to connect to the DB he intends to connect.
Cheers!


Hi Victor, yes, that's the idea. Each user will have his own database. But then I need to make every one to connect dynamically. I think that with a KernelRequest subscriber is the best option.
If anyone finds out a better solution, please, tell me. Thanks!!
Hey Carlos,
I like your initial idea with connecting to it dynamically on KernelRequest, you should try it. Unfortunately, don't know if there is any better solution. Good luck with it!
Cheers!


Just an info, this dialog seems to be new when installing doctrine via composer:
Symfony operations: 3 recipes (d1677be98e0980609549e3b67c08b1cb)
- WARNING doctrine/doctrine-cache-bundle (>=1.3): From github.com/symfony/recipes-...
The recipe for this package comes from the "contrib" repository, which is open to community contributions.
Review the recipe at https://github.com/symfony/...
Do you want to execute this recipe?
[y] Yes
[n] No
[a] Yes for all packages, only for the current installation session
[p] Yes permanently, never ask again for this project
(defaults to n):
If you get this, just hit y to confirm this dialog.
Hey Mike,
Thank you for this hint! Yes, it appears if you use Symfony Flex that may do routine things for you from the recipe for some bundles, you can check what will be done with the official repo for contrib bundles: https://github.com/symfony/... or just press y and validate the diff with "git diff" command. That's why it's recommended to commit changes before installing new bundles, it will help you to validate changes easier.
Cheers!
i am very dissapointed in this chapter the create database command do not work in all cases and for noobs like me you do not give options, very bad because i pay for a product that works and an advice is not so hard to make by experts like you at SymfonyCasts
Hey Jorge!
We're sorry to see you disappointed! Sometimes we do not explain simple things, like things that should be obvious at some point. In some cases we suppose that most of users should know these things already. But that's exactly why we have comments below *every* video and we track all the comments with our team and provide support for any screencast related questions that are not clear to users.
We would be happy to assist you with your problem, but could you clarify what problem exactly do you have? Do you see any errors? What exactly the errors? If you have an error with "doctrine:database:create" command - most probably it's due to credentials problem, but it's difficult to say more without knowing the error message you have.
Cheers!


For everyone that has the connection refused error:
Add the following parameter to doctrines.yaml: "unix_socket: /path/to/mysql.sock"
For MAMP is this: /Applications/MAMP/tmp/mysql/mysql.sock
Symfony doctrine docs:
https://symfony.com/doc/cur...
It took me hours to figure this out, hope this helps someone.
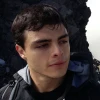
Hey Andy E.
Thanks for sharing it! But I believe this should not happen if you don't change the default install configuration of MySql
Cheers!


No problem, just an extra solution.


Hi,
I followed the course from step 1.
But when creating the database I get the next error:
$ php bin/console doctrine:database:create
In AbstractMySQLDriver.php line 106:
An exception occurred in driver: could not find driver
In PDOConnection.php line 31:
Could not find driver
In PDOConnection.php line 27:
Could not find driver
I've haven't got any clue what's going wrong..
Hey Maarten!
Ah! I know this pesky error! The issue is that you're missing a PHP extension - probably the pdo and/or pdo_mysql extensions. How you install these varies on every operating system, unfortunately. For example, if you're using Ubuntu, then it'll be something like sudo apt-get install php7.2-mysql
(depending on PHP version).
Let me know if that helps! And if you're having trouble finding how to install the extension, tell us your operating system and how you installed PHP and we'll do our best to help :).
Cheers!
Thank you Ryan, I was having this problem too :)
I have PHP 7.4 atm and running sudo apt-get install php7.4-mysql did the trick for me!
Hey Jelle,
Thank you for sharing your solution with others! Glad it helped you :)
Cheers!
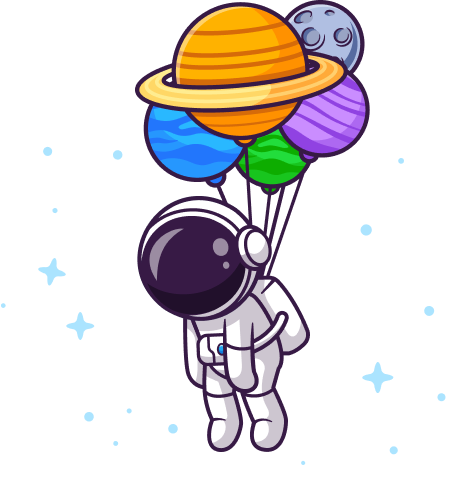
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.1.3",
"ext-iconv": "*",
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/doctrine-bundle": "^1.6.10", // 1.10.2
"doctrine/doctrine-migrations-bundle": "^1.3|^2.0", // v2.0.0
"doctrine/orm": "^2.5.11", // v2.7.2
"knplabs/knp-markdown-bundle": "^1.7", // 1.7.0
"knplabs/knp-time-bundle": "^1.8", // 1.8.0
"nexylan/slack-bundle": "^2.0,<2.2.0", // v2.0.0
"php-http/guzzle6-adapter": "^1.1", // v1.1.1
"sensio/framework-extra-bundle": "^5.1", // v5.1.4
"stof/doctrine-extensions-bundle": "^1.3", // v1.3.0
"symfony/asset": "^4.0", // v4.0.4
"symfony/console": "^4.0", // v4.0.14
"symfony/flex": "^1.0", // v1.21.6
"symfony/framework-bundle": "^4.0", // v4.0.14
"symfony/lts": "^4@dev", // dev-master
"symfony/twig-bundle": "^4.0", // v4.0.4
"symfony/web-server-bundle": "^4.0", // v4.0.4
"symfony/yaml": "^4.0" // v4.0.14
},
"require-dev": {
"doctrine/doctrine-fixtures-bundle": "^3.0", // 3.0.2
"easycorp/easy-log-handler": "^1.0.2", // v1.0.4
"fzaninotto/faker": "^1.7", // v1.7.1
"symfony/debug-bundle": "^3.3|^4.0", // v4.0.4
"symfony/dotenv": "^4.0", // v4.0.14
"symfony/maker-bundle": "^1.0", // v1.4.0
"symfony/monolog-bundle": "^3.0", // v3.1.2
"symfony/phpunit-bridge": "^3.3|^4.0", // v4.0.4
"symfony/stopwatch": "^3.3|^4.0", // v4.0.4
"symfony/var-dumper": "^3.3|^4.0", // v4.0.4
"symfony/web-profiler-bundle": "^3.3|^4.0" // v4.0.4
}
}
Hint for MariaDB Users, change server_version from this:
server_version: '5.7'
to your corresponding server version (By example 10.3.13):
server_version: 'mariadb-10.3.13'