Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: ^7.4.1
Subscribe to download the code!Compatible PHP versions: ^7.4.1
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
make:docker:database
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Doctrine is installed! Woo! Now we need to make sure a database is running - like MySQL or PostgreSQL - and then update the DATABASE_URL
environment variable to point to it.
Show Lines
|
// ... lines 1 - 27 |
DATABASE_URL=mysql://db_user:db_password@127.0.0.1:3306/db_name?serverVersion=5.7 | |
Show Lines
|
// ... lines 29 - 30 |
So: you can absolutely start a database manually: you can download MySQL or PostgreSQL onto your machine and start it. Or you can use Docker, which is what we will do. OooOoooo.
Using Docker?
Now, hold on: if you're nervous about Docker... or you haven't used it much... or you used it and hated it, stay with me! Using Docker is optional for this tutorial, but we're going to use it in a very lightweight way.
The only requirement to get started is that you need to have Docker downloaded and running on your machine. I already have Docker running on my machine for Mac.
Docker is all about creating tiny containers - like a container that holds a MySQL instance and another that holds a PHP installation. Traditionally, when I think of Docker, I think of a full Docker setup: a container for PHP, a container for MySQL and another container for Nginx - all of which communicate to each other. In that situation, you don't have anything installed on your "local" machine except for Docker itself.
That "full Docker" setup is great - and, if you like it, awesome. But it also adds complexity: sharing source code with the containers can make your app super slow - especially on a Mac - and if you need to run a bin/console
command, you need to execute that from within a Docker container.
Our Simple Docker Plan
And so, instead, we're going to do something much simpler. First, we are going to have PHP installed on our local machine - I do have PHP installed on my Mac. Then, we're just going to use Docker to launch services like MySQL, Redis or Elasticsearch. Finally, we'll configure our local PHP app to communicate with those containers.
For me, it's kind of the best of both worlds: it makes it super easy to launch services like MySQL... but without the complexity that often comes with Docker.
Hello make:docker:database
Ok, ready? To manage our Docker containers, we need to create a docker-compose.yaml
file that describes what we need.
Tip
If you're using the latest version of Symfony Flex, then when you
ran composer require orm
, it asked you if you wanted Docker
configuration. If you answered yes, congrats! You can skip this
step because you already have a docker-compose.yml
file with
a database
service inside. Skip ahead to around 4:30 when we
run docker-compose up
.
That file is pretty simple but... let's cheat! Find your terminal and run:
php bin/console make:docker:database
This command comes from MakerBundle version 1.20... and I love it. A big thanks to community member Jesse Rushlow for contributing this!
Ok: it doesn't see a docker-compose.yaml
file, so it's going to create a new one. I'll use MySQL and, for the version - I'll use latest
- we'll talk more about that in a few minutes.
And... done! The database service is ready!
Well, in reality, the only thing this command did was create a docker-compose.yaml
file: it didn't communicate with Docker or start any containers - it just created this new docker-compose.yaml
file.
version: '3.7' | |
services: | |
database: | |
image: 'mysql:latest' | |
environment: | |
MYSQL_ROOT_PASSWORD: password | |
ports: | |
# To allow the host machine to access the ports below, modify the lines below. | |
# For example, to allow the host to connect to port 3306 on the container, you would change | |
# "3306" to "3306:3306". Where the first port is exposed to the host and the second is the container port. | |
# See https://docs.docker.com/compose/compose-file/#ports for more information. | |
- '3306' |
And... it's pretty basic: we have a service called database
- that's just an internal name for it - which uses a mysql
image at its latest
version. And we're setting an environment variable in the container that makes sure the root user password is... password
! At the bottom, the ports
config means that port 3306 of the container will be exposed to our host machine.
That last part is important: this will make it possible for our PHP code to talk directly to MySQL in that container. This syntax actually means that port 3306 of the container will be exposed to a random port on our host machine. Don't worry: I'll show you exactly what that means.
docker-compose up
So... yay! We have a docker-compose.yaml
file! To start all of the containers that are described in it... which is just one - run:
docker-compose up -d
The -d
means "run as a daemon" - it runs in the background instead of holding onto my terminal.
The first time you run this it will take a bit longer because it needs to download MySQL. But eventually.... yes! With one command, we now have a database running in the background!
So... how do we communicate with it? Next, let's learn a bit more about docker-compose
including how to connect to the MySQL instance and shut down the container.
43 Comments


Had the same problem, but this worked fine, thanks!
However I can't get the reason, why you got such an error? I tested this course and it shouldn't be here at all =)
Hey Bartosz G.
Thanks for sharing you experience!
Cheers!
For anyone that might encounter the same issue I had, when running docker-compose up -d
and getting :zsh: command not found: docker-compose
try running:docker compose version
and if you get your version here, run:docker compose up -d
instead of docker-compose up -d
This worked for me, hope it helps


Here is the error thrown at me trying to make a docker database:
bin/console make:docker:database
PHP Fatal error: During class fetch: Uncaught ReflectionException: Class "Symfony\Component\Validator\Mapping\Loader\AutoMappingTrait" not found while loading "Symfony\Bridge\Doctrine\Validator\DoctrineLoader". in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php:181
Stack trace:
#0 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php(31): Symfony\Component\Config\Resource\ClassExistenceResource::throwOnRequiredClass('Symfony\\Compone...')
#1 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/error-handler/DebugClassLoader.php(337): include('/Users/ashatou/...')
#2 [internal function]: Symfony\Component\ErrorHandler\DebugClassLoader->loadClass('Symfony\\Bridge\\...')
#3 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php(84): class_exists('Symfony\\Bridge\\...')
#4 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/ContainerBuilder.php(348): Symfony\Component\Config\Resource\ClassExistenceResource->isFresh(0)
#5 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(337): Symfony\Component\DependencyInjection\ContainerBuilder->getReflectionClass('Symfony\\Bridge\\...', false)
#6 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(323): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableType(Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'doctrine.orm.de...', Object(Symfony\Component\DependencyInjection\Definition))
#7 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(433): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableTypes(Object(Symfony\Component\DependencyInjection\ContainerBuilder))
#8 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(407): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeAlternatives(Object(Symfony\Component\DependencyInjection\ContainerBuilder), Object(Symfony\Component\DependencyInjection\TypedReference))
#9 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(388): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeNotFoundMessage(Object(Symfony\Component\DependencyInjection\TypedReference), 'it', '.service_locato...')
#10 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Definition.php(911): Symfony\Component\DependencyInjection\Compiler\AutowirePass->Symfony\Component\DependencyInjection\Compiler\{closure}()
#11 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(843): Symfony\Component\DependencyInjection\Definition->getErrors()
#12 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(1019): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addService('.errored..servi...', Object(Symfony\Component\DependencyInjection\Definition))
#13 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(242): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addServices(NULL)
#14 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(715): Symfony\Component\DependencyInjection\Dumper\PhpDumper->dump(Array)
#15 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(543): Symfony\Component\HttpKernel\Kernel->dumpContainer(Object(Symfony\Component\Config\ConfigCache), Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'App_KernelDevDe...', 'Container')
#16 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(131): Symfony\Component\HttpKernel\Kernel->initializeContainer()
#17 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(168): Symfony\Component\HttpKernel\Kernel->boot()
#18 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(74): Symfony\Bundle\FrameworkBundle\Console\Application->registerCommands()
#19 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/console/Application.php(140): Symfony\Bundle\FrameworkBundle\Console\Application->doRun(Object(Symfony\Component\Console\Input\ArgvInput), Object(Symfony\Component\Console\Output\ConsoleOutput))
#20 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/bin/console(43): Symfony\Component\Console\Application->run(Object(Symfony\Component\Console\Input\ArgvInput))
#21 {main} in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php on line 31
Fatal error: During class fetch: Uncaught ReflectionException: Class "Symfony\Component\Validator\Mapping\Loader\AutoMappingTrait" not found while loading "Symfony\Bridge\Doctrine\Validator\DoctrineLoader". in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php:181
Stack trace:
#0 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php(31): Symfony\Component\Config\Resource\ClassExistenceResource::throwOnRequiredClass('Symfony\\Compone...')
#1 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/error-handler/DebugClassLoader.php(337): include('/Users/ashatou/...')
#2 [internal function]: Symfony\Component\ErrorHandler\DebugClassLoader->loadClass('Symfony\\Bridge\\...')
#3 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php(84): class_exists('Symfony\\Bridge\\...')
#4 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/ContainerBuilder.php(348): Symfony\Component\Config\Resource\ClassExistenceResource->isFresh(0)
#5 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(337): Symfony\Component\DependencyInjection\ContainerBuilder->getReflectionClass('Symfony\\Bridge\\...', false)
#6 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(323): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableType(Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'doctrine.orm.de...', Object(Symfony\Component\DependencyInjection\Definition))
#7 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(433): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableTypes(Object(Symfony\Component\DependencyInjection\ContainerBuilder))
#8 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(407): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeAlternatives(Object(Symfony\Component\DependencyInjection\ContainerBuilder), Object(Symfony\Component\DependencyInjection\TypedReference))
#9 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(388): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeNotFoundMessage(Object(Symfony\Component\DependencyInjection\TypedReference), 'it', '.service_locato...')
#10 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Definition.php(911): Symfony\Component\DependencyInjection\Compiler\AutowirePass->Symfony\Component\DependencyInjection\Compiler\{closure}()
#11 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(843): Symfony\Component\DependencyInjection\Definition->getErrors()
#12 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(1019): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addService('.errored..servi...', Object(Symfony\Component\DependencyInjection\Definition))
#13 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(242): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addServices(NULL)
#14 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(715): Symfony\Component\DependencyInjection\Dumper\PhpDumper->dump(Array)
#15 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(543): Symfony\Component\HttpKernel\Kernel->dumpContainer(Object(Symfony\Component\Config\ConfigCache), Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'App_KernelDevDe...', 'Container')
#16 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(131): Symfony\Component\HttpKernel\Kernel->initializeContainer()
#17 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(168): Symfony\Component\HttpKernel\Kernel->boot()
#18 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(74): Symfony\Bundle\FrameworkBundle\Console\Application->registerCommands()
#19 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/console/Application.php(140): Symfony\Bundle\FrameworkBundle\Console\Application->doRun(Object(Symfony\Component\Console\Input\ArgvInput), Object(Symfony\Component\Console\Output\ConsoleOutput))
#20 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/bin/console(43): Symfony\Component\Console\Application->run(Object(Symfony\Component\Console\Input\ArgvInput))
#21 {main} in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php on line 31
Symfony\Component\ErrorHandler\Error\FatalError {#5216
#message: """
Error: During class fetch: Uncaught ReflectionException: Class "Symfony\Component\Validator\Mapping\Loader\AutoMappingTrait" not found while loading "Symfony\Bridge\Doctrine\Validator\DoctrineLoader". in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php:181\n
Stack trace:\n
#0 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php(31): Symfony\Component\Config\Resource\ClassExistenceResource::throwOnRequiredClass('Symfony\\Compone...')\n
#1 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/error-handler/DebugClassLoader.php(337): include('/Users/ashatou/...')\n
#2 [internal function]: Symfony\Component\ErrorHandler\DebugClassLoader->loadClass('Symfony\\Bridge\\...')\n
#3 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php(84): class_exists('Symfony\\Bridge\\...')\n
#4 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/ContainerBuilder.php(348): Symfony\Component\Config\Resource\ClassExistenceResource->isFresh(0)\n
#5 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(337): Symfony\Component\DependencyInjection\ContainerBuilder->getReflectionClass('Symfony\\Bridge\\...', false)\n
#6 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(323): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableType(Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'doctrine.orm.de...', Object(Symfony\Component\DependencyInjection\Definition))\n
#7 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(433): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableTypes(Object(Symfony\Component\DependencyInjection\ContainerBuilder))\n
#8 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(407): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeAlternatives(Object(Symfony\Component\DependencyInjection\ContainerBuilder), Object(Symfony\Component\DependencyInjection\TypedReference))\n
#9 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(388): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeNotFoundMessage(Object(Symfony\Component\DependencyInjection\TypedReference), 'it', '.service_locato...')\n
#10 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Definition.php(911): Symfony\Component\DependencyInjection\Compiler\AutowirePass->Symfony\Component\DependencyInjection\Compiler\{closure}()\n
#11 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(843): Symfony\Component\DependencyInjection\Definition->getErrors()\n
#12 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(1019): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addService('.errored..servi...', Object(Symfony\Component\DependencyInjection\Definition))\n
#13 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(242): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addServices(NULL)\n
#14 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(715): Symfony\Component\DependencyInjection\Dumper\PhpDumper->dump(Array)\n
#15 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(543): Symfony\Component\HttpKernel\Kernel->dumpContainer(Object(Symfony\Component\Config\ConfigCache), Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'App_KernelDevDe...', 'Container')\n
#16 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(131): Symfony\Component\HttpKernel\Kernel->initializeContainer()\n
#17 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(168): Symfony\Component\HttpKernel\Kernel->boot()\n
#18 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(74): Symfony\Bundle\FrameworkBundle\Console\Application->registerCommands()\n
#19 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/console/Application.php(140): Symfony\Bundle\FrameworkBundle\Console\Application->doRun(Object(Symfony\Component\Console\Input\ArgvInput), Object(Symfony\Component\Console\Output\ConsoleOutput))\n
#20 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/bin/console(43): Symfony\Component\Console\Application->run(Object(Symfony\Component\Console\Input\ArgvInput))\n
#21 {main}
"""
#code: 0
#file: "./vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php"
#line: 31
-error: array:4 [
"type" => 1
"message" => """
During class fetch: Uncaught ReflectionException: Class "Symfony\Component\Validator\Mapping\Loader\AutoMappingTrait" not found while loading "Symfony\Bridge\Doctrine\Validator\DoctrineLoader". in /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php:181\n
Stack trace:\n
#0 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php(31): Symfony\Component\Config\Resource\ClassExistenceResource::throwOnRequiredClass('Symfony\\Compone...')\n
#1 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/error-handler/DebugClassLoader.php(337): include('/Users/ashatou/...')\n
#2 [internal function]: Symfony\Component\ErrorHandler\DebugClassLoader->loadClass('Symfony\\Bridge\\...')\n
#3 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/config/Resource/ClassExistenceResource.php(84): class_exists('Symfony\\Bridge\\...')\n
#4 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/ContainerBuilder.php(348): Symfony\Component\Config\Resource\ClassExistenceResource->isFresh(0)\n
#5 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(337): Symfony\Component\DependencyInjection\ContainerBuilder->getReflectionClass('Symfony\\Bridge\\...', false)\n
#6 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(323): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableType(Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'doctrine.orm.de...', Object(Symfony\Component\DependencyInjection\Definition))\n
#7 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(433): Symfony\Component\DependencyInjection\Compiler\AutowirePass->populateAvailableTypes(Object(Symfony\Component\DependencyInjection\ContainerBuilder))\n
#8 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(407): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeAlternatives(Object(Symfony\Component\DependencyInjection\ContainerBuilder), Object(Symfony\Component\DependencyInjection\TypedReference))\n
#9 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Compiler/AutowirePass.php(388): Symfony\Component\DependencyInjection\Compiler\AutowirePass->createTypeNotFoundMessage(Object(Symfony\Component\DependencyInjection\TypedReference), 'it', '.service_locato...')\n
#10 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Definition.php(911): Symfony\Component\DependencyInjection\Compiler\AutowirePass->Symfony\Component\DependencyInjection\Compiler\{closure}()\n
#11 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(843): Symfony\Component\DependencyInjection\Definition->getErrors()\n
#12 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(1019): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addService('.errored..servi...', Object(Symfony\Component\DependencyInjection\Definition))\n
#13 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/dependency-injection/Dumper/PhpDumper.php(242): Symfony\Component\DependencyInjection\Dumper\PhpDumper->addServices(NULL)\n
#14 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(715): Symfony\Component\DependencyInjection\Dumper\PhpDumper->dump(Array)\n
#15 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(543): Symfony\Component\HttpKernel\Kernel->dumpContainer(Object(Symfony\Component\Config\ConfigCache), Object(Symfony\Component\DependencyInjection\ContainerBuilder), 'App_KernelDevDe...', 'Container')\n
#16 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/http-kernel/Kernel.php(131): Symfony\Component\HttpKernel\Kernel->initializeContainer()\n
#17 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(168): Symfony\Component\HttpKernel\Kernel->boot()\n
#18 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/framework-bundle/Console/Application.php(74): Symfony\Bundle\FrameworkBundle\Console\Application->registerCommands()\n
#19 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/console/Application.php(140): Symfony\Bundle\FrameworkBundle\Console\Application->doRun(Object(Symfony\Component\Console\Input\ArgvInput), Object(Symfony\Component\Console\Output\ConsoleOutput))\n
#20 /Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/bin/console(43): Symfony\Component\Console\Application->run(Object(Symfony\Component\Console\Input\ArgvInput))\n
#21 {main}
"""
"file" => "/Users/ashatou/Work (Local)/2. Personal/11. Learning/Symfony/SymfonyCast/code-symfony5-doctrine/code-symfony5-doctrine/start/vendor/symfony/doctrine-bridge/Validator/DoctrineLoader.php"
"line" => 31
]
}
Yo @ashatou!
Yikes! That's an ugly error! This appears to be a bug in DoctrineBundle when used with php 8.1 and it was fixed in DoctrineBundle 2.4.3 - https://github.com/doctrine/DoctrineBundle/issues/1377
Unfortunately, upgrading the code from this project to that version of DoctrineBundle isn't as simple as a "composer update". I just checked, and to get compatible versions of everything, a bunch of things need to be updated, including (at least) parts of Symfony itself and the doctrine-extensions library.
I'd recommend checking out our new Symfony 6 Doctrine tutorial. Or, you can definitely use this downloaded code - but upgrade Symfony to version 5.4 and then running composer update
. To do this, you'd replace all of the 5.1.*
with 5.4.*
in composer.json
and then run composer update
. When I tried that on the "finish" code just now, it allowed DoctrineBundle to upgrade and the error went away on PHP 8.1 :).
Cheers!


Sadly enough for what I am willing to do Docker is not an option I am willing to pay for. It would be appreciated that solutions get provided for common usecases like xampp and mamp
For those who don't want to use Docker and use xampp
# config/packages/doctrine.yaml
dbal:
override_url: true
url: '%env(resolve:DATABASE_URL)%'
driver: 'pdo_mysql'
server_version: '5.7'
charset: utf8
unix_socket: /Applications/XAMPP/xamppfiles/var/mysql/mysql.sock
default_table_options:
charset: utf8
collate: utf8_general_ci
And if you use mapp
change the unix_socet: to
unix_socket: /Applications/MAMP/tmp/mysql/mysql.sock
Hey Pieter-jan C.!
I'm really sorry you're unhappy - and I don't blame you :/. We need to do better. I did try to mention how things would be done without Docker, but clearly, I was too vague about that. Tahnks for posting your solution here. Most people (but not all!) will not need to mess with the unix_socket thing (I'm mentioning this for the benefit of others) - most PHP installs will already be looking in the correct place for it.
Cheers!


Well ok, but how many people will use docker on a live website? Most use cases will be a localy run db. All fun and games but docker costs money as soon as you want a private db, and i believe that anyone who wants a website online wants a private one :D. So maybe a idea. Make a video or 2 to explain a setup for a local db?
Hey Pieter-jan C.!
> Make a video or 2 to explain a setup for a local db?
We definitely need to do that :) - at least the basics so people can choose to run Docker locally if they want to.
> Well ok, but how many people will use docker on a live website? Most use cases will be a localy run db.
I completely agree! I mean, there are many people who do use in on production, but this chapter is *all* about using Docker locally as a development tool - it has nothing to do with using it on a live site. But maybe that also was unclear? I'd appreciate the feedback if that part was confusing as well.
Anyways, thanks for the conversation - I chatted about your feedback so far with the team earlier today :).
Cheers!
It looks like docker-compose down will destroy the container and the db, vs. stop which will allow it to persist?
How would you add a named volume to the docker-compose.yaml?
Howdy Brian!
When you run docker-compose down
it will destroy all of the containers, the docker network those containers use, and any data within the containers (e.g. the database) that are defined in the docker-compose.yaml
. If you run docker-compose stop
, all of the containers are preserved (including the data within them) but are "turned off". Think of it as turning off you laptop, docker-compose stop
would turn off your laptop until you were ready to fire it back up. docker-compose start database
would turn it back on again.
In regards to volumes, assuming your docker-compose.yaml
is in your projects root directory, you can add volumes to the container(s) by editing your docker-compose.yaml
file as follows.
// docker-compose.yaml
services:
database:
image: 'postgres:latest'
...
volumes:
- ./relative-path-on-host:/abusolute-path-in-container
The above code would add two separate volumes to your container. As a word of caution - when using volumes, the docker container has read/write access to the data on the host and vice versa. If you were to delete a file from within the container, it would also be removed from the host (assuming file permissions were allowed). However, docker-compose down
would <i><b>not</b></i> destroy the data within the volume on the host.
For database containers, if you want to preserve a modified database configuration using the native configuration files, you can add a volume to the container as follows. This assumes you have created a .data/config
directory within your project. The host directory name / location can be anywhere.
// MySql / MariaDB
- ./.data/config:/etc/mysql
// Postgres
- ./.data/config:/usr/share/postgresql
For the actual database data itself, you would add a volume like:
// MySql / MariaDB
- ./.data/var:/var/lib/mysql
// Postgres
- ./.data/var:/var/lib/postgres/data
A complete MySQL example with configuration and data volumes on the host:
services:
database:
image: 'postgres:latest'
...
volumes:
- ./.data/config:/etc/mysql
- ./.data/var:/var/lib/mysql
I hope this helps! If you have anymore questions - feel free to ask!
Thanks
Jesse
The block quote above does not preserve indentation - check out https://gist.github.com/jrushlow/853bc0822dd68064bf78ebb565b0ed37 for the complete example.
I got your indentation fixed up Jesse - it's super annoying to due right with Disqus 😠.
Thanks for sharing this. Do you know what the paths are for MySQL and Pgsql that should be shared? For pgsql, I know there is an example here: https://github.com/symfony/...
Cheers!
I just saw that @jesserushlow added the exact volume paths needed for MySQL above - thanks for that!


How do I connect directly to MySQL? I'm frustrated with getting
Docker to work as I've tried every solution listed here without success. It has completely stopped my progress with this course.


Nevermind. I had to enable the pdo_mysql extension in php.ini
Hey Will,
I'm glad you were able to fix it by yourself! That was an easy fix :)
Cheers!


I get this error:ERROR: Version in "./docker-compose.yaml" is unsupported. You might be seeing this error because you're using the wrong Compose file version. Either specify a supported version (e.g "2.2" or "3.3") and place your service definitions under the
services key, or omit the
version key and place your service definitions at the root of the file to use version 1.<br />For more on the Compose file format versions, see https://docs.docker.com/compose/compose-file/
my installed version is 3.7.
any ideas?
hey Maike L.
Can you double-check that you have this line version: '3.7'
at the top of your file? I just downloaded the course code and I can see it there
Cheers!


I think he didn't do good job in this lecture. he simply said I have "docker" installed already. but what about someone who hasn't? I am using ubuntu on windows and am really confused.
If you run docker-compose --version
what version do you see? If it's 3.7 then it should run but for some other reason it isn't. Try upgrading docker-compose and see if it fixes the problem.
I think he didn't do good job in this lecture...
Well, that's not so easy to achieve and especially because this is not a Docker tutorial. There're many OS with many possible setups, it would take a lot of time explaining how to install Docker and how to fix errors but thanks for your feedback we take into account messages like this one so we can improve our tutorial-making process.
Cheers!


I ran docker-compose --version and it says version 1.27.4
How do I upgrade?
EDIT: On docs.docker.com it says that 1.27.4 is the current stable version. So how did you get to 3.7?


edit 3.7 to 2 in docker-compose.yaml
Hey @Farry7!
The docker-compose version stuff is confusing:
> Docker Compose version file 3 was introduced in release 1.10.0 of Docker Compose and 1.13.0 release of Docker Engine
So basically, the "version: 3" in the docker-compose.yaml file is not referring to the version of docker-compose itself (I know, confusing) - but a bit more about "version 3 of the docker-compose API". But, your version - 1.27.4 - does tell us that it is plenty new enough to support version 3 (and also version 3.7... because you have the absolute newest version of docker-compose). It's also very likely that your Docker engine is new enough to support version 3.7 - it requires version 18.06.0+.
Are you getting a version that the docker-compose version is not supported by your Docker? If so, then probably your Docker Engine is too old... but you can also probably change the version to 3.0 in your docker-compose.yaml file - I don't believe we really use any "new" features in that file that require 3.7 (but I'm not 100% sure).
Anyways, let me know what you're seeing and we'll try to help - sorry for the troubles!
Cheers!


The problem is not docker-compose. I cant get the daemon running. I gave it up. Am trying to use XAMPP instead. Looks like im gonne be one of those guys who hate docker
Hey there!
Sorry about the troubles - we appreciate the feedback! Honestly, for some people Docker is the best thing ever. For others, it’s an unnecessary pain. That’s actually why we (A) make it an optional part of this tutorial and (B) we use a, kind of, “light” integration with Docker.
Anyways, skip Docker! And if you any trouble with the database config part, please let us know :).
Cheers!


Now when I run sudo php bin/console doctrine:database:create
I get this error:SQLSTATE[HY000] [2002] Connection refused
this is my .env
file:
`
APP_ENV=dev
APP_SECRET=85153b13c0dad127897ae85b27e6b47b
DATABASE_URL="mysql://root:MY_PASSWORD@localhost:3306/db_name?serverVersion=5.7"
`
any Ideas?


Better late then never, bet try the DATABASE_URL without quotes, i.e.
DATABASE_URL=mysql://root:MY_PASSWORD@localhost:3306/db_name?serverVersion=5.7
That worked for me, somehow my system does not like the variable inside double quotes...
I think the sudo
may have been a factor here as well. You should not have to use sudo for any of the Symfony console commands.
Hey Maike L.!
Hmm. The "Connection refused" usually means that the database isn't running... or that there is some other problem. It's... unfortunately, kind of a "generic" MySQL error that could mean a lot of things :/. Are you able to use the same connection parameters in, for example, some MySQL client like TablePlus https://tableplus.com/ to connect? Also, try 127.0.0.1
instead of localhost
. That usually does not make any difference, but it can in some edge cases. Btw, I can tell you for sure the problem is not aa bad password of anything like that - it's some sort of connection error to the server.
Cheers!


Ok. I try to go on without this docker thing. Wish me luck


yes I have it


Hi there there ,
I would like to point out a problem, that may stop some windows users from using docker.
I have a bunch of vagrant boxes (using oracle vm virtualbox) for local developement on different projects.
Docker requires hyper-v to be activated, but on the other side Virtualbox hyper-v support is still experimental and in my case it causes a major performance issue that prevents me from working with vagrant.
Anyway, I will switch to my notebook, so i can follow along with your tutorial.
Cheers!
Hey mr_d
Thanks for sharing it with others. Windows is always tricky to work with as a programmer but we have hope that they will do a good job someday :)
Cheers!
i keep getting this error ERROR: no matching manifest for windows/amd64 10.0.18362 in the manifest list entries
after running docker-compose up -d , and i think the solution is to use docker linux container and in my case i can--t switch to linux container.
it by default a linux container but i never made it to start docker , so i switched to windows container because i am using windows 10 pro it worked
but now i am blocked and i really do wanna use docker
any help pleaaaaase
thank you
it working now , what i did is free up a lot of space on my machine and resize resources in docker
Hey hamzaAB!
Thanks for sharing your problem and solution! So it seems that you just needed more resources to be able to run Docker? That is super interesting...
Cheers!
Hey, thanks for this tutorial.
In the .env file of the course source code is defined 'DATABASE_URL=...?serverVersion=5.7' but the version installed by Docker seems to be 8.0, the serverVersion needs to match the actual MySQL version installed ?
Hey Tavo
That's a great question and technically yes, you should update the DATABASE_URL env var to match your database connection but in the next chapters you'll learn why, while using Symfony's webserver, you don't have to do that
Cheers!
So.. the only place I do have to replace the environment variables is while I'm running tests (env.test file) ? I'm not sure I understand how the config of the test database would be if I have a specific database for testing and another one for the dev environment.
How you deal with environment variables depends on your server. If you're running, let's say, on Apache, you can configure your host to set the values of the env vars you need. That would be a way to do it but Symfony gives you another way, through the .env
file. In theory, you should have one file for your production/development environment, and another one for the testing environment .env.test
. So, inside .env.test
you have to configure the DATABASE_URL
variable to point to your test database, and that's it. This post may help you out understanding how it works https://symfony.com/blog/new-in-symfony-4-2-define-env-vars-per-environment
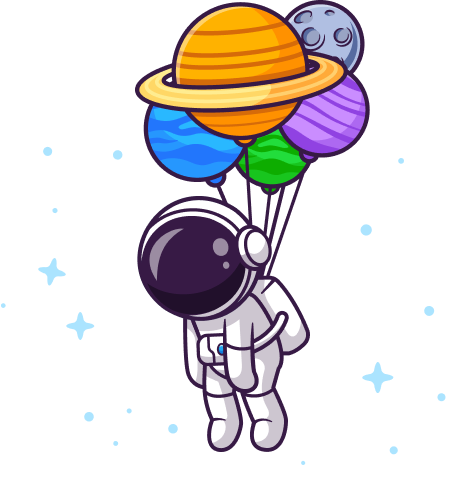
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.4.1",
"ext-ctype": "*",
"ext-iconv": "*",
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/doctrine-bundle": "^2.1", // 2.1.1
"doctrine/doctrine-migrations-bundle": "^3.0", // 3.0.2
"doctrine/orm": "^2.7", // 2.8.2
"knplabs/knp-markdown-bundle": "^1.8", // 1.9.0
"knplabs/knp-time-bundle": "^1.11", // v1.16.0
"sensio/framework-extra-bundle": "^6.0", // v6.2.1
"sentry/sentry-symfony": "^4.0", // 4.0.3
"stof/doctrine-extensions-bundle": "^1.4", // v1.5.0
"symfony/asset": "5.1.*", // v5.1.2
"symfony/console": "5.1.*", // v5.1.2
"symfony/dotenv": "5.1.*", // v5.1.2
"symfony/flex": "^1.3.1", // v1.21.6
"symfony/framework-bundle": "5.1.*", // v5.1.2
"symfony/monolog-bundle": "^3.0", // v3.5.0
"symfony/stopwatch": "5.1.*", // v5.1.2
"symfony/twig-bundle": "5.1.*", // v5.1.2
"symfony/webpack-encore-bundle": "^1.7", // v1.8.0
"symfony/yaml": "5.1.*", // v5.1.2
"twig/extra-bundle": "^2.12|^3.0", // v3.0.4
"twig/twig": "^2.12|^3.0" // v3.0.4
},
"require-dev": {
"doctrine/doctrine-fixtures-bundle": "^3.3", // 3.4.0
"symfony/debug-bundle": "5.1.*", // v5.1.2
"symfony/maker-bundle": "^1.15", // v1.23.0
"symfony/var-dumper": "5.1.*", // v5.1.2
"symfony/web-profiler-bundle": "5.1.*", // v5.1.2
"zenstruck/foundry": "^1.1" // v1.5.0
}
}
Hey,
I'll leave a comment to help anyone who takes this course and encountered this error after running make:docker:databas:
Could not check compatibility between Symfony\Bridge\ProxyManager\LazyProxy\PhpDumper\LazyLoadingValueHolderGenerator::generate(ReflectionClass$originalClass, Zend\Code\Generator\ClassGenerator $classGenerator): <br />void and ProxyManager\ProxyGenerator\LazyLoadingValueHolderGenerator::generate(ReflectionClass $originalClass, Laminas\Code\Generator\ClassGenerator $classGenerator),because class Zend\Code\Generator\ClassGenerator is not available in/home/nathan/dev/plateforme/facturation/vendor/symfony/proxy-manager-bridge/LazyProxy/PhpDumper/LazyLoadingValueHolderGenerator.php on line 33
Upgrading to symfony 5.3 fixed the problem, in order to do that you've got to update symofny as well as it's packages.
Head to composer.json and under "require:" change versions from 5.0. (for all packages) to 5.3. plus under "extra" change symfony version to 5.3.*
Code linked here works perfectly fine with symfony 5.3