Chapters
This course is archived!
While the concepts of this course are still largely applicable, it's built using an older version of Symfony (4) and React (16).
-
Course Code
Subscribe to download the code!Compatible PHP versions: ^7.2.0
Subscribe to download the code!Compatible PHP versions: ^7.2.0
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
The World of React + ESLint
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Hey friends! And welcome! Oh, I am so excited to talk about React. Developing in React feels great, and it's powerful! You can build any crazy frontend you want. But, honestly, writing this tutorial was a huge pain. Technically speaking, React is not hard. But, to even get started, you need to be comfortable with ES6 features and you need a build system, like Webpack Encore. That's why we covered both of those topics in previous tutorials.
But even then! The best practices around React are basically non-existent, especially for a backend developer, who instead of building a single page app, may just want to use React to power part of their frontend.
So our goal in this tutorial is clear: to master React, but also learn repeatable patterns you can follow to write high-quality code while getting your wonderful new app finished, and out the door. We won't hide anything: we'll attack the ugliest stuff and, as always, build something real.
Excited? Me too! And, a huge thanks to my co-author on this tutorial Frank de Jonge, who helped me navigate many of these important topics.
Project Setup
If you'd like free high-fives from Frank... or if you want to get the most out of this tutorial, you should totally code along with me. Download the course code from this page. When you unzip it, you'll find a start/
directory inside that holds the same code that you see here. Open up the README.md
file for winning lottery numbers and instructions on how to get the project setup.
The last steps will be to open a terminal, move into the project, and run:
php bin/console server:run
to start the built-in web server. Our project already uses Webpack Encore to compile its CSS and JS files. If you're new to Webpack or Encore, go watch our tutorial on that first.
To build those assets, pour some coffee, open a second terminal tab, and run:
yarn install
to download our Node dependencies. And... once that finishes:
yarn run encore dev --watch
That will build our assets, and rebuild when we change files.
Ok cool! Let's go check out the app: find your browser, go to http://localhost:8000 and say hello to the Lift Stuff App! Login with user ron_furgandy
password pumpup
.
In our effort to stay in shape... while sitting down and coding all day... we've built Lift Stuff: an app where we can record all the stuff we've lifted throughout the day. For example, before I started recording, I lifted my big fat cat 10 times... so let's totally log that!
In the previous tutorials, we built this JavaScript frontend using plain JavaScript and jQuery. In this tutorial, we'll re-build it with React.
Installing ESLint
But before we dive into React, I want to install another library that will make life much more interesting. Move back to your terminal, open a third terminal tab - we're getting greedy - and run:
yarn add eslint --dev
ESLint is a library that can detect coding standard violations in your JavaScript. We have similar tools in PHP, like PHP-CS-Fixer. To configure exactly which coding standard rules we want to follow, back in our editor, create a new file at the root of the project: .eslintrc.js
.
I'll paste in some basic configuration here: you can copy this from the code block on this page. We won't talk about ESLint in detail, but this basically imports the ESLint recommended settings with a couple of tweaks. This jsx
part is something we'll see very soon in React.
module.exports = { | |
extends: ['eslint:recommended'], | |
parserOptions: { | |
ecmaVersion: 6, | |
sourceType: 'module', | |
ecmaFeatures: { | |
jsx: true | |
} | |
}, | |
env: { | |
browser: true, | |
es6: true, | |
node: true | |
}, | |
rules: { | |
"no-console": 0, | |
"no-unused-vars": 0 | |
} | |
}; |
Thanks to this, we can now run a utility to check our code:
./node_modules/.bin/eslint assets
where assets/
is the directory that holds our existing JavaScript code. And... aw, boring! It looks like all of our code already follows the rules.
This utility is nice... but there's a more important reason we installed it. In PhpStorm, open the settings and search for eslint
to find an ESLint section. Click to Enable this and hit Ok. Yep, PhpStorm will now instantly tell us when we've written code that violates our rules.
Tip
If you have node
installed via Docker, you can configure PhpStorm to find and use it.
See https://www.jetbrains.com/help/phpstorm/node-with-docker.html#ws_node_docker_configure_interpreter.
Check this out: open assets/js/rep_log.js
: this is the file that runs our existing LiftStuff frontend. Here, add const foo = true
then if (foo)
, but leave the body of the if
statement empty. See that little red error? That comes from ESLint.
This may not seem very important, but it's going to be super helpful with React.
Adding a new Entry
As I mentioned, our app is already built in normal JavaScript. Instead of deleting our old code immediately, let's leave it here and build our React version right next to it. In the same directory as rep_log.js
, which holds the old code, create a new file: rep_log_react.js
. Log a top-secret, important message inside so that we can see if it's working. Don't forget the Emoji!
console.log('Oh hallo React peeps! ?️'); |
Now, open webpack.config.js
: we're going to configure this as a new "entry". Typically, you have one entry file per page, and that file holds all of the JavaScript you need for that page. Use addEntry('rep_log_react')
pointing to that file: ./assets/js/rep_log_react.js
.
Show Lines
|
// ... lines 1 - 3 |
Encore | |
Show Lines
|
// ... lines 5 - 12 |
.addEntry('rep_log_react', './assets/js/rep_log_react.js') | |
Show Lines
|
// ... lines 14 - 28 |
; | |
Show Lines
|
// ... lines 30 - 33 |
To build this, go back to your terminal, find the tab that is running Webpack Encore, press Ctrl+C to stop it, and run it again: you need to restart Webpack whenever you change its config file.
Finally, to add the new JavaScript file to our page, open templates/lift/index.html.twig
, find the javascripts
block, and add the script tag for rep_log_react.js
. You don't normally want two entry files on the same page like this. But when we finish, I plan to delete the old rep_log.js
file.
And just like that, we can find our browser, open the dev tools, go to the console, refresh and... Hello World!
Now, it's time to go say Hello to React!
62 Comments
Hey @xmontero!
I really appreciate the suggestions for notes - that's huge for us ❤️
About this first note/card, that's definitely a fair opinion! I've just added a note to the script (it should show up in a few minutes) and the video note will follow in a few days or weeks (that part happens async later).
Cheers!


Hi there,
I am trying to set things up for my own project. I followed the tutorial and I get a page that loads my js-file generated based on my assets/main.js. Opening this js in firefox developper tools shows me this:
var el = react__WEBPACK_IMPORTED_MODULE_0__.createElement('h2', null, "Heading 2");
console.log(el);
react_dom__WEBPACK_IMPORTED_MODULE_1__.render(el, document.getElementById('react-inhalt'));
I am not quite sure if this __WEBPACK.... is correct. I am wondering because I do NOT get any output from console.log nor in the browser. Any hints what I am successfully ignoring?
I think I am missing the part that tells the system to execute my js.
Thx
Oliver
Hey Oliver W.!
Hmm. First, all the crazy WEBPACK stuff is fine, but looks, yes, a bit nuts :). Internally, when Webpack parses all of your source files, it re-organizes them into one big output file... and then wraps it in a bunch of code to help it direct traffic internally. For example, ` reactWEBPACK_IMPORTED_MODULE_0__` is almost certainly a local variable Webpack creates in the file that is set to the "react" module.
Anyways, the question now is: why don't you see any logs (or errors)? I'm not sure. In theory, if you have a Webpack entry that points to assets/main.js
as your source file, then if you put a console.log(el)
directly inside that file, it should be executed. Webpack may wrap a bunch of things in a fancy way, but that line SHOULD be called. In other words, Webpack already knows that it should "execute assets/main.js", simply because that's your webpack entry.
So, does this help at all? What does your main.js file look like? Is the react and react-dom code you have directly inside this main.js file? Or is it somewhere else that's imported?
Cheers!


Hey Ryan,
that's what my main.js looks like:
import React from 'react';
import ReactDom from 'react-dom';
const el = React.createElement('h2', null, "Heading 2");
console.log(el);
ReactDom.render(el, document.getElementById('react-inhalt'));```
And I still believe that my code is NOT executed. Even if I replace the `import React from 'react';` with `import React from 'reacter';` there is no error in the console or the browser.
By the way: is the content of package.json of any interest?
{
"devDependencies": {
"@hotwired/stimulus": "^3.0.0",
"@symfony/stimulus-bridge": "^3.0.0",
"@symfony/webpack-encore": "^1.7.0",
"core-js": "^3.0.0",
"eslint": "^8.10.0",
"react": "^17.0.2",
"react-dom": "^17.0.2",
"regenerator-runtime": "^0.13.2",
"webpack-notifier": "^1.6.0"
},
"license": "UNLICENSED",
"private": true,
"scripts": {
"dev-server": "encore dev-server",
"dev": "encore dev",
"watch": "encore dev --watch",
"build": "encore production --progress"
}
}`
Meanwhile I added a file to public/js with a console.log. This one is executed. My react-js is still NOT executed. And I learned: do not manually add a file to public/build. It will be deleted by yarn watch the next time it runs.
HTH
Oliver
HeyOliver W.!
<s>Hmm. With that app.js
file you SHOULD, at the very least, see your console.log(el)
. Since you're having such odd behavior, I would back up a bit. Try introducing a syntax error to app.js. Do you see the error in the console of your browser? What if you delete all of the content and just console.log('foo')
? Do you see that? If not, can you find that code inside one of your built files in public/?</s>
The above is still relevant, but I think I may have figured out the issue, so start down here :). Are you using encore_entry_script_tags('app')
in your base.html.twig file? If not, replace the script tags that point to the public/build directory with that one line. Reference: https://github.com/symfony/recipes/blob/master/symfony/twig-bundle/5.4/templates/base.html.twig
Explanation: this tutorial was built with (and the code download ships with) an old version of Encore where you could just add manual script tags. In newer versions, you need to use this fancy Twig function (which is easier anyways). If this wild guess is correct, you're using updated code (I can tell you are because of your package.json) but still the "old" way of adding the script and link tags in base.html.twig.
Let me know if I've guessed correctly!
Cheers!


Hi Ryan,
I am sorry, but your guess is wrong 8-(.
That's what my base.html.twig looks likie:
<!DOCTYPE html>
<html lang="de">
<head>
<meta charset="UTF-8">
<title>{% block title %}Welcome!{% endblock %}</title>
<link rel="icon" href="data:image/svg+xml,<svg xmlns=%22http://www.w3.org/2000/svg%22 viewBox=%220 0 128 128%22><text y=%221.2em%22 font-size=%2296%22>⚫️</text></svg>">
{# Run `composer require symfony/webpack-encore-bundle` to start using Symfony UX #}
{% block stylesheets %}
{{ encore_entry_link_tags('app') }}
{% endblock %}
{% block javascripts %}
{{ encore_entry_script_tags('app') }}
{% endblock %}
</head>
<body>
{% block body %}{% endblock %}
</body>
</html>```
And the template for the affected page:
{% extends 'base.html.twig' %}
{% block title %}Test{% endblock %}
{% block body %}
<h1>Test</h1>
<div class="test">
<div id="react-content"></div>
</div>
{% endblock %}
{% block javascripts %}
{{ parent() }}
<script src="{{ asset('js/test.js') }}"></script>{{ endblock }}```
The js/test.js is the js with just a console.log(). Nothing else. My console.log() shows up, the console.log() from app_test.js does not. Inside is nothing else than console.log() and alert(),
Here what the console shows:
GEThttp://react.local/test
[HTTP/1.1 200 OK 172ms]
GEThttp://react.local/build/app.css
[HTTP/1.1 200 OK 1ms]
GEThttp://react.local/build/runtime.js
[HTTP/1.1 200 OK 1ms]
GEThttp://react.local/build/vendors-node_modules_symfony_stimulus-bridge_dist_index_js-node_modules_core-js_modules_es_ob-7db861.js
[HTTP/1.1 304 Not Modified 4ms]
GEThttp://react.local/build/app.js
[HTTP/1.1 304 Not Modified 14ms]
GEThttp://react.local/js/test.js
[HTTP/1.1 200 OK 1ms]
Wir sind hier js/test.js test.js:1:```
Sorry for the bad news but somehow I am glad that it isn't that obvious ;-)
Oliver
Oh darn! And I was so excited to have "figured it out" ;). And yes, you're right that it doesn't appear to be anything obvious!
Well, your setup looks perfect: you have the proper functions in base.html.twig and your console tells me that all the JavaScript files I would expect to be downloaded ARE being downloaded, and successfully. I would do a force refresh just in case (I noticed the 304 Not Modified on the build/app.js), but I'm guessing you've already tried this and it's not the issue.
So, this is really just a question of Webpack/Encore: something is behaving badly. Just to be clear, you showed the source code for your main.js
file a few comments ago. You meant assets/app.js
correct? Could you also show me your webpack.config.js file (at least the "entries" part of it)?
And what if you introduce a syntax error into assets/app.js? Does that show up in your browser's console? Or does it still happily show nothing. The behavior is so weird that I'm suspect of some caching issue / some issue where your browser is not actually downloading the correct code. Are you using yarn watch
to build the code?
Cheers!


OMG, that's embarassing. The missing code is in app_test.js. But all I linked was app.js. I should have discussed that with my rubber druck before posting. As soon as I added{{ encore_entry_script_tags('app_test') }}
it worked.
So once again, an I/O-error (Idiot Operator).
But thanks to your help we found it.
Thx for your time and assistance. I appreciated it very much - as always.
Maybe I can return the favour - some day <gd&rvf>
And unfortunately I am not yet done with this all. My final goal is MUI 8-(.
Ha! Phew! I'm just glad it works - I was running out of ideas! :D
> Thx for your time and assistance. I appreciated it very much - as always.
My pleasure! And good luck with MUI: that looks cool!


Hi, Im using this with Symfony 5, where `composer require encore` gives me stimulus (as part of Symfony UX i think). I wish to build without stimulus, using only React and webpack-encore. Is it possible? If not, what is the best way to remove all stimulus thingies?
Hey Milan,
That should be pretty minor, and you can completely ignore it because you have no Stimulus controllers anyway, it's just set up a config that will help you to start faster with Stimulus if you want, but almost takes nothing if you do not use Stimulus. I'd recommend you to just ignore that config.
But in case you really want to remove everything related to Stimulus - you would need to remove the Stimulus from Yarn dependencies and a demo stimulus controller named hello_controller.js including controllers/ folder and all its related code as well, like stimulus_bootstrap.js files. You probably may want to leverage "git grep -i stimulus" to find all matches of it in your project and drop them.
But as I said, that's just a tiny config, and if you don't have any Stimulus controllers - you can just ignore it, you will be easier to update Webpack Encore Bundle's Symfony Flex recipe later. But it's totally up to you ;)
Cheers!


Hello, I am getting this error when I run php bin/console doctrine:database:create
"An exception occurred in driver: SQLSTATE[HY000] [2002] No connection could
be made because the target machine actively refused it."
Any advice?
I just answered you via email :)


hey man, just got the same problem
could you please help me out?
Thanks in advance :-)
Hey @yassine
Could you double-check that you have MySql up and running in your local machine, and also check for the PHP PDO module, it's required as well
Cheers!


I'm unable to log in.
I get the message Authentication request could not be processed due to a system problem.
Not really sure where to look, and although I've no idea if it would even matter, I get a 404 error for (the non existent) /build/manifest.js, and the placeholders for Username and Password are security.login.username and security.login.password respectively, not Username and Password as in the video.
The lack of manifest.js and the untranslated placeholders make me wonder if I'm missing something, but no errors occurred for any steps in README.md.
Hey Jim C.
If there isn't a manifest.js file it means that you haven't run yarn
. You have to install yarn in your computer first, then run
yarn install
yarn watch
I hope it helps. Let me know if you still have problems. Cheers!


I <i>have</i> run yarn
. Both yarn install
and yarn run encore dev --watch
As I said, I ran <i>all</i> steps in <b>README.md</b>. Also just tried yarn watch
, but no <b>manifest.js</b>
[edit]
There is a <b>manifest.json</b>
So there is a manifest.json file, interesting... let's debug it further. After you try to log in, click on the web debug toolbar and find for *that* request, so we can see the stack trace of it and find what's throwing that error


Aha!
Had a look at the request, found a 302 error which in turn mentioned a mysql driver error, which I've long since learned almost always means a credential error.
After a bit of head scratching realised that at some point after creating and populating the database, I'd gone back into my docker-compose.yml and made a typo in the db environment info.
Thanks very much for suggesting that, as otherwise I'd've spent ages trying to work out why there's no manifest.js, and if that was the problem. Checking the debug bar should have been the first thing I did, but no, had to make it harder for myself and bother other people -- like you -- into the bargain.
LOL - Don't worry man, as programmers we love to take the tougher path first. Cheers!


Hi,
I have installed npm but i can not run npm in CMD.
I have got below error
npm ERR! missing script: encore
npm ERR! A complete log of this run can be found in:
Hey Michael P.!
Hmm. Are you using npm instead of yarn? In that case, try running npx run encore dev --watch
?
That should be the equivalent command. For both yarn and npm (with npx), this is just a shorcut for executing a binary that's located at ./node_modules/.bin/encore
.
Let me know if that helps!
Cheers!
Hi I just downloaded the code from this champter (fresh new project) and then run composer install in the START directory, and all was fine, but when I ran the comand yarn install, I got like 84 differents errors and 104 warnings ... any advice?? I'm using VSC text editor, on Windows 10... the final error just said that:`C:\Program Files (x86)\Microsoft Visual Studio\2017\BuildTools\MSBuild\15.0\Bin\MSBuild.exe
failed with exit code: 1` :(...Regards and thanks in advance!!
Hey Toshiro
What yarn version did you use?
Cheers
bah.. my bad I forgot to ask about nodejs version, sometimes latest versions of nodejs can throw errors.
Cheers and sorry for my bad attention to details :)
Hey Toshiro!
Hmm. Can you see exactly which part of it failed? One thing that fails for me frequently is the node-sass "build step", which is different on every operating system. It's a bit of a tricky thing - if your dependencies get even a little bit out-of-date, then suddenly that can fail on "yarn install". I've got a feeling that this is related to your problem... and we might need to bump our dependencies to help with this.
Try this to work around for now:
yarn upgrade node-sass
And if that doesn't work, go big with:
yarn upgrade
I be that will solve your problem. But let me know - we'll check into updating the dependencies in the download.
Cheers and sorry about the issue!
THANKS!!!! it work perfectly with the upgrade of node-sass!! all is good now!! thanks a lot!! Cheers!!
Hey I can't seem to log in to the webapp from the start code.
In var/log/dev.log after trying to log in with run_furgandy, pumpup (also tried other usernames)
Link to gist of logfile
So there are a bunch of info and debug level messages and deprecated notices.
EDIT: moved logfile to github gist!
Hey August S.
I don't see any errors in your logs. So nothing is failing. I'm assuming that you are getting the "Invalid credentials" message, if that's the case I believe you forgot to run the fixtures php bin/console doctrine:fixtures:load
Please do that and try again
Cheers!
Are you guys also planning on doing a course on Vue.js since some newer courses like Messenger or API Platform Security have their frontend built on it? I would love to build a single page app but it seems so hard to really build something good with react since it would require react router, redux and more complex stuff that's difficult to learn and find best practices, and in general it's not entirely clear to me how things should be done with react :(
Hey Tobias I.!
It's on our list... but no timeline yet for it... which means it won't be the next few tutorials. Personally, having studied both Vue and React quite a bit at this point, while they're very similar to each other (in the way that PHP frameworks all more or less do the same thing) I found Vue easier to get into and more forgiving. So, if you're looking to try something, try Vue. And... we'll do our best to get a tutorial out sometime ;).
Cheers!

Hello Guys,
I was wondering why we don't use {{ encore_entry_script_tags('app') }}
to insert the js files?
Hey Skylar
Simple answer, this function wasn't available when this course was created =) So it uses more oldschool file including!
Cheers!

Hey guys,
In this tutorial, it says to use the built-in server, by executing php bin/console server:run
and goto http://127.0.0.1:8000/
.
I get this error:
`Warning: SessionHandler::read(): open(/var/lib/php/sessions/sess_ee1fkh12mm054l54aip03ecpg3, O_RDWR) failed: Permission denied (13)
and in the console:
[Fri May 17 09:08:27 2019] 127.0.0.1:54223 Invalid request (Unsupported SSL request)<br />but if I use the new
symfony serve and use the secure link https:
https://127.0.0.1:8000` I have no problems.
hey Skylar
Feel free to use symfony-cli server instead of build-in server =) And your issue probably caused by symfony-cli server which you run for the first time. Browser saved http to https redirect, but built-in server cannot serve ssl connection. To run built-in server try to start it at another port probably 8080
it should run than.
Cheers!


Hello.
I have a project with interactive React JS games.
Tell me how to better organize the structure of such project? Create multiple entry points to Webpack for each game? Each game has its own script. There can be hundreds of such entry points. It's not very good.
It may be possible to generate the script in production for a separate game, save the source code in a safe place and proceed to the development of the next script from the same entry point.
I am completely confused. Help me please.
Hey Dmitriy !
Can you tell me about more about the games? Are you developing many different games in React, and each is playable on a different URL? If so, yea, you totally could create a different entry point for each one - that's 100% logic. But, I agree, it could be messy.
With the little I know, here's what I would do:
A) Create a single entry point that is able to "boot" a game
B) By parsing the URL (or using a Route, which is cleaner), determine which "game" the user wants to play.
C) Use a dynamic / AJAX import to "import" React component for the game that is being requested and render it. This page isn't for React, but this shows the async import I'm talking about: https://symfony.com/doc/cur...
This would allow you to have a big list of all the games inside your main entrypoint file. Then, via a router, if statement, or something similar, you determine which import() code to execute. Webpack will then import that component, and you'll render it.
I hope this helps!
Cheers!


Thanks Ryan. Yes, each game is playable on a different URL.
Idea with async code splitting is a really great. I will use it. What about a webpack in a development environment? It needs to follow the changes in a huge number of JavaScript files.
Is it possible to somehow make it follow and work only the script that is currently in development.
Maybe I need to comment entry points that are not currently used?
Hey Dmitriy!
Idea with async code splitting is a really great. I will use it.
Great!
What about a webpack in a development environment? It needs to follow the changes in a huge number of JavaScript files.
Nothing should be different in the dev environment - if you refresh, Webpack will AJAX-download the updated script tag and your page will have the updates. Are you having performance problems with --watch
because your app is so huge?
Cheers!


Thank you, Ryan. I understood. You really helped me. The project is still at the design stage. I will check in practice.
Hello, I have a problem at first step of readme. When i run composer install in the project I get:
[KO]
Script cache:clear returned with error code 1
!!
!! In UnitOfWork.php line 2718:
!!
!! Warning: "continue" targeting switch is equivalent to "break". Did you mean
!! to use "continue 2"?
!!
!!
!!
Script @auto-scripts was called via post-install-cmd
Hey!
Yea, this is a bug with PHP 7.3 and our version of the dependencies. We're going to upgrade the dependencies soon to avoid this. You can also do this on your own by running composer update doctrine/orm
- the bug was fixed in doctrine/orm 2.6.3.
Sorry about that! New versions of PHP bring in new little bugs sometimes :).
Cheers!


I have added ESLint, but I get the following warning:
[3/4] 🔗 Linking dependencies...
warning " > babel-loader@7.1.5" has unmet peer dependency "webpack@2 || 3 || 4".
warning " > sass-loader@6.0.7" has unmet peer dependency "webpack@^2.0.0 || ^3.0.0 || ^4.0.0".
But I already have Webpack installed?
Hey Shaun T.!
You can ignore these :). This is a little incompatibility with how npm packages work and how Webpack Encore works. In Encore, we require webpack as a dependency so that YOU don't have to. But, this means that you get these above warnings. They're not actually a problem, as these libraries will just use the Webpack provided by Encore internally, but they are annoying, and confusing. We may change Encore in the future to require *you* to install Webpack to avoid these - I'm not sure - something I think about :).
Cheers!


Thanks for the clarification weaverryan :)
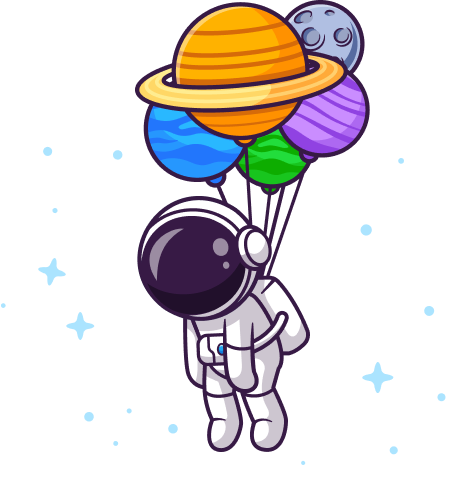
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.2.0",
"ext-iconv": "*",
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"doctrine/annotations": "^1.0", // v1.8.0
"doctrine/doctrine-bundle": "^1.6", // 1.9.1
"doctrine/doctrine-cache-bundle": "^1.2", // 1.3.3
"doctrine/doctrine-fixtures-bundle": "~3.0", // 3.0.2
"doctrine/doctrine-migrations-bundle": "^1.2", // v1.3.1
"doctrine/orm": "^2.5", // v2.7.2
"friendsofsymfony/jsrouting-bundle": "^2.2", // 2.2.0
"friendsofsymfony/user-bundle": "dev-master#4125505ba6eba82ddf944378a3d636081c06da0c", // dev-master
"phpdocumentor/reflection-docblock": "^3.0|^4.0", // 4.3.0
"sensio/framework-extra-bundle": "^5.1", // v5.2.0
"symfony/asset": "^4.0", // v4.1.4
"symfony/cache": "^3.3|^4.0", // v4.1.4
"symfony/console": "^4.0", // v4.1.4
"symfony/flex": "^1.0", // v1.21.6
"symfony/form": "^4.0", // v4.1.4
"symfony/framework-bundle": "^4.0", // v4.1.4
"symfony/lts": "^4@dev", // dev-master
"symfony/monolog-bundle": "^3.1", // v3.3.0
"symfony/polyfill-apcu": "^1.0", // v1.9.0
"symfony/property-access": "^3.3|^4.0", // v4.1.4
"symfony/property-info": "^3.3|^4.0", // v4.1.4
"symfony/serializer": "^3.3|^4.0", // v4.1.4
"symfony/swiftmailer-bundle": "^3.1", // v3.2.3
"symfony/twig-bundle": "^4.0", // v4.1.4
"symfony/validator": "^4.0", // v4.1.4
"symfony/yaml": "^4.0", // v4.1.4
"twig/twig": "2.10.*" // v2.10.0
},
"require-dev": {
"easycorp/easy-log-handler": "^1.0.7", // v1.0.7
"symfony/debug-bundle": "^3.3|^4.0", // v4.1.4
"symfony/dotenv": "^4.0", // v4.1.4
"symfony/maker-bundle": "^1.5", // v1.5.0
"symfony/phpunit-bridge": "^4.0", // v4.1.4
"symfony/stopwatch": "^3.3|^4.0", // v4.1.4
"symfony/var-dumper": "^3.3|^4.0", // v4.1.4
"symfony/web-profiler-bundle": "^3.3|^4.0", // v4.1.4
"symfony/web-server-bundle": "^4.0" // v4.1.4
}
}
What JavaScript libraries does this tutorial use?
// package.json
{
"dependencies": {
"@babel/plugin-proposal-object-rest-spread": "^7.12.1" // 7.12.1
},
"devDependencies": {
"@babel/preset-react": "^7.0.0", // 7.12.5
"@symfony/webpack-encore": "^0.26.0", // 0.26.0
"babel-plugin-transform-object-rest-spread": "^6.26.0", // 6.26.0
"babel-plugin-transform-react-remove-prop-types": "^0.4.13", // 0.4.13
"bootstrap": "3", // 3.3.7
"copy-webpack-plugin": "^4.4.1", // 4.5.1
"core-js": "2", // 1.2.7
"eslint": "^4.19.1", // 4.19.1
"eslint-plugin-react": "^7.8.2", // 7.8.2
"font-awesome": "4", // 4.7.0
"jquery": "^3.3.1", // 3.3.1
"promise-polyfill": "^8.0.0", // 8.0.0
"prop-types": "^15.6.1", // 15.6.1
"react": "^16.3.2", // 16.4.0
"react-dom": "^16.3.2", // 16.4.0
"sass": "^1.29.0", // 1.29.0
"sass-loader": "^7.0.0", // 7.3.1
"sweetalert2": "^7.11.0", // 7.22.0
"uuid": "^3.2.1", // 3.4.0
"webpack-notifier": "^1.5.1", // 1.6.0
"whatwg-fetch": "^2.0.4" // 2.0.4
}
}
Hi, I just bougth this amazing course. Congrats Ryan and Frank.
As time elapses, things evolve, I find 2 things that could be worth nothing in "cards" in the videos one for this video and the other for video 2. Will post separate comments.
For this video...
When configuring eslint inside PhpStorm a notice about "Hey, if you run your server remotely, for example in a docker, you still can configure PHP to interact with eslint seamlessly. Just configure a "remote node.js" following this https://www.jetbrains.com/help/phpstorm/node-with-docker.html#ws_node_docker_configure_interpreter. It's as simple as telling PhpStorm "use this docker engine and run a disposable container of this image that contains the node binary at this path" and PhpStorm will start linting your files via eslint exactly as described in the video".
I never configured a remote node.js before and just knowing "it is possible" will relax when watching your videos. If no comment is done it's clear it's a "local installation" and stress arises while watching the video thinking "hey, my setup is different". Following PhpStorm docs is super-straigh forward. Mentioning the entry-point here would raise value.