Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: ^7.1.3
Subscribe to download the code!Compatible PHP versions: ^7.1.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Subtitles
Subscribe to download the subtitles!
Subscribe to download the subtitles!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Bootstrapping the Bundle & Autoloading
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Heeeeey Symfony peeps! I'm excited! Because we're going to dive deep in to a super interesting topic: how to create your own bundles. This is useful if you need to share code between your own projects. Or if you want to share your great new open source library with the whole world. Actually, forget that! This tutorial is going to be awesome even if you don't need to do either of those. Why? Because we use third-party bundles every day. And by learning how to create one, we're going to become experts in how they work and really get a look at Symfony under the hood.
As always, you can earn free high-fives by downloading the source code from this page and coding along with me. After unzipping the file, you'll find a start/
directory with the same code that you see here. Follow the README.md
file for steps on how to get your project setup.
The last step will be to open a terminal, move into the project, sip your coffee, and run:
php bin/console server:run
to start the built-in PHP web server.
Introducing KnpUIpsum
Head to your browser and go to http://localhost:8000
. Say hello to The Space Bar! This is a Symfony application - the one we're building in our beginner Symfony series. Click into one of the articles to see a bunch of delightful, fake text that we're using to make this page look real. Each time you refresh, you get new random, happy content.
To find out where this is coming from, in the project, open src/Service/KnpUIpsum.php
. Yes! This is our new creation: it returns "lorem ipsum" dummy text, but with a little KnpUniversity flare: the classic latin is replaced with rainbows, unicorns, sunshine and more of our favorite things.
Show Lines
|
// ... lines 1 - 2 |
namespace App\Service; | |
Show Lines
|
// ... lines 4 - 9 |
class KnpUIpsum | |
{ | |
private $unicornsAreReal; | |
private $minSunshine; | |
public function __construct(bool $unicornsAreReal = true, $minSunshine = 3) | |
{ | |
$this->unicornsAreReal = $unicornsAreReal; | |
$this->minSunshine = $minSunshine; | |
} | |
/** | |
* Returns several paragraphs of random ipsum text. | |
* | |
* @param int $count | |
* @return string | |
*/ | |
public function getParagraphs(int $count = 3): string | |
{ | |
$paragraphs = array(); | |
for ($i = 0; $i < $count; $i++) { | |
$paragraphs[] = $this->addJoy($this->getSentences($this->gauss(5.8, 1.93))); | |
} | |
return implode("\n\n", $paragraphs); | |
} | |
Show Lines
|
// ... lines 37 - 338 |
} |
And, you know what? I think we all deserve more cupcakes, kittens & baguettes in our life. So I want to share this functionality with the world, by creating the KnpULoremIpsumBundle! Yep, we're going to extract this class into its own bundle, handle configuration, add tests, and do a bunch of other cool stuff.
Right now, we're using this code inside of ArticleController
: it's being passed to the constructor. Below, we use that to generate the content.
Show Lines
|
// ... lines 1 - 2 |
namespace App\Controller; | |
Show Lines
|
// ... lines 4 - 14 |
class ArticleController extends AbstractController | |
{ | |
/** | |
* Currently unused: just showing a controller with a constructor! | |
*/ | |
private $isDebug; | |
private $knpUIpsum; | |
public function __construct(bool $isDebug, KnpUIpsum $knpUIpsum) | |
{ | |
$this->isDebug = $isDebug; | |
$this->knpUIpsum = $knpUIpsum; | |
} | |
/** | |
* @Route("/", name="app_homepage") | |
*/ | |
public function homepage() | |
{ | |
return $this->render('article/homepage.html.twig'); | |
} | |
Show Lines
|
// ... lines 37 - 75 |
} |
Isolating into a new Bundle Directory
Ok, the first step to creating a new bundle is to move this code into its own location. Eventually, all the code for the bundle will live in its own completely separate directory & repository. But, sometimes, when you first start building, it's a bit easier to keep the code in your project: it let's you hack on things really quickly & test them in your app.
So let's keep the code here for now, but isolate it from the app's code. To do that, create a new lib/
directory. And then, another called LoremIpsumBundle
: this will be the temporary home for our shiny bundle. Inside, there are a few valid ways to organize things, but I like to create a src/
directory.
mkdir lib
mkdir lib/LoremIpsumBundle
mkdir lib/LoremIpsumBundle/src
Tip
You can also just type one command instead of three:
mkdir -p lib/LoremIpsumBundle/src
Perfect! Now, move the KnpUIpsum
class into that directory. And yea, you could put this into a src/Service
directory, or anywhere else you want.
New Vendor Namespace
Oh, but this namespace will not work anymore. We need a namespace that's custom to our bundle. It could be anything, but usually it has a vendor part - like KnpU
and then the name of the library or bundle - LoremIpsumBundle
.
Show Lines
|
// ... lines 1 - 2 |
namespace KnpU\LoremIpsumBundle; | |
Show Lines
|
// ... lines 4 - 9 |
class KnpUIpsum | |
Show Lines
|
// ... lines 11 - 340 |
And, that's it! If we had decided to put KnpUIpsum
into a sub-directory, like Service
, then we would of course also add Service
to the end of the namespace like normal.
Next, back in ArticleController
, go up to the top, remove the use statement, and re-type it to get the new one.
Show Lines
|
// ... lines 1 - 2 |
namespace App\Controller; | |
Show Lines
|
// ... line 4 |
use KnpU\LoremIpsumBundle\KnpUIpsum; | |
Show Lines
|
// ... lines 6 - 77 |
Handling Autoloading
So... will it work! Yea... probably not - but let's try it! Nope! But I do love error messages:
Cannot autowire ArticleController argument $knpUIpsum... because the KnpUIpsum class was not found.
Of course! After creating the new lib/
directory, we need to tell Composer's autoloader to look for the new classes there. Open composer.json
, find the autoload
section, and add a new entry: the KnpU\\LoremIpsumBundle\\
namespace will live in lib/LoremIpsumBundle/src/
.
Show Lines
|
// ... lines 1 - 36 |
"autoload": { | |
"psr-4": { | |
"KnpU\\LoremIpsumBundle\\": "lib/LoremIpsumBundle/src/", | |
Show Lines
|
// ... line 40 |
} | |
}, | |
Show Lines
|
// ... lines 43 - 77 |
Then, open a new terminal tab. To make the autoload changes take effect, run:
composer dump-autoload
Registering the Service
Will it work now? Try it! Bah, not yet: but we're closer. The error changed: instead of "class not found", now it says that no KnpUIpsum
service exists. To solve this, open config/services.yaml
.
Thanks to the auto-registration code in here, we don't normally need to register our classes as services: that's automatic. But, it's only automatic for classes that live in src/
. Yep, as soon as we moved the class from src/
to lib/
, that service disappeared.
And that's ok! When you create a re-usable bundle, you actually don't want to rely on auto-registration or autowiring. Instead, as a best-practice, you should configure everything explicitly to avoid any surprises.
To do that, at the bottom of this file, add KnpU\LoremIpsumBundle\KnpUIpsum: ~
.
Show Lines
|
// ... lines 1 - 5 |
services: | |
Show Lines
|
// ... lines 7 - 37 |
KnpU\LoremIpsumBundle\KnpUIpsum: ~ |
Tip
If you're on Symfony 4.4 or higher, you can remove this KnpU\LoremIpsumBundle\KnpUIpsum: ~
configuration line from the config/services.yaml
file.
This adds a new service for that class. And because we don't need to pass any options or arguments, we can just set this to ~
. The class does have constructor arguments, but they have default values.
Ok, try it again! Yes! It finally works! We've successfully isolated our code into its own directory and we are ready to hack! Next, let's make this a bundle with a bundle class and start digging into how bundles can automatically register services.
53 Comments
Hey Andrada,
Woops, we're sorry about that! Actually, the concept of creating Symfony bundles remains the same in Symfony 5, so everything we're showing in this course should be valid for Symfony 5 applications. Could you clarify what exactly does not work? Because "nothings works" does not say much. If you have an error - please, share in the comment and we would be glad to help you handle it.
Cheers!
Well, some help here would really be appreciated! If I install symfony 5 and then I copy paste the course code then I cannot get it to work at all due to error after error.
I have cloned symfony 4 and now I try to figure out what is the best way to incorporate the course code as again I experienced errors and missing services like the slack one and then phpunit error and then composer error on doctrine which does not get fixed with package install. The symfony version is 4.4.7.
Please help!
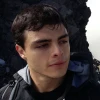
Hey andradacrisan
I got a bit lost among all the messages :)
Looks like you had just forgotten to copy the .env
file to your project, right?
About the autowiring error, that's because you need to copy the files inside config
directory as well
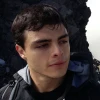
Hey andradacrisan
I believe something didn't go well when copying the course files to your Symfony4.4 project. I just tried installing the course code and it works (at least in my machine. I'm using PHP 7.4.3
This course uses Symfony4.0 but if you want to work with Symfony4.4 you can run
composer upgrade "symfony/*" --with-dependencies```
and everything should work fine
Please, give it a try and let us know if you keep getting problems. Cheers!
And I get this error when I try to paste course files only the public folder and the ones in src, only the needed ones in Symfony 4.4.7:
Cannot autowire service "App\Controller\ArticleController": argument "$isDebug" of method "__construct()" is type-hinted "bool", you should configure its value explicitly.
I have installed just the code from the course in the start folder and on running composer install this is what I get:
- Installing symfony/web-profiler-bundle (v4.0.6): Loading from cache
- Installing symfony/twig-bundle (v4.0.6): Loading from cache
- Installing symfony/profiler-pack (v1.0.3): Loading from cache
Package symfony/lts is abandoned, you should avoid using it. Use symfony/flex instead.
Generating autoload files
Executing script cache:clear [KO]
[KO]
Script cache:clear returned with error code 255
!!
!! Fatal error: Uncaught Symfony\Component\Dotenv\Exception\PathException: Unable to read the "/Users/andrada/Sites/test-symfony/bin/../.env" environment file. in /Users/andrada/Sites/test-symfony/vendor/symfony/dotenv/Dotenv.php:54
!! Stack trace:
!! #0 /Users/andrada/Sites/test-symfony/bin/console(22): Symfony\Component\Dotenv\Dotenv->load('/Users/andrada/...')
!! #1 {main}
!! thrown in /Users/andrada/Sites/test-symfony/vendor/symfony/dotenv/Dotenv.php on line 54
!! PHP Fatal error: Uncaught Symfony\Component\Dotenv\Exception\PathException: Unable to read the "/Users/andrada/Sites/test-symfony/bin/../.env" environment file. in /Users/andrada/Sites/test-symfony/vendor/symfony/dotenv/Dotenv.php:54
!! Stack trace:
!! #0 /Users/andrada/Sites/test-symfony/bin/console(22): Symfony\Component\Dotenv\Dotenv->load('/Users/andrada/...')
!! #1 {main}
!! thrown in /Users/andrada/Sites/test-symfony/vendor/symfony/dotenv/Dotenv.php on line 54
!!
Script @auto-scripts was called via post-install-cmd
Any ideas? I am running PHP 7.3.16
I have copied an .env file from another Symfony 4.4.7 version to the course files and now it is working. But...this file should be in the course files for the project to run correctly!!
oh sorry, another error just when I thought it all goes well:
Environment variable not found: "SLACK_WEBHOOK_ENDPOINT".
How to fix that?
Thank you!
Edit: Solved it by watching the course from Symfony 4 Fundamentals - Environment Variables


I'm getting this...
(1/1) UndefinedMethodException
Attempted to call an undefined method named "hasBeenStarted" of class "Symfony\Component\HttpFoundation\Session\Session".
... just after downloading, "composer install" and "php bin/console server:run".
Any clue??
Hi Carlos
Nice catch! There was a little package incompatibility in course code. But now it's fixed. The best solution for you is to re-download course code and try again. Everything should work!
Cheers!


For people wanting to do this course but running into some installation problems, i found this the easiest solution:
Install docker and follow this:
https://blog.rafalmuszynski.pl/how-to-configure-docker-for-symfony-4-applications/
(or find another: running symfony with docker)
Then go into your container, run this in the Docker folder:
sudo docker-compose exec php bash
Now we are in the docker container and can run:
composer install.
We have a working application at http://localhost:8080/
Hey Wout,
Thank you for sharing this tip with others! Yes, unfortunately, this course code works only on PHP 7.x - you either have to install a legacy PHP 7 on your laptop and use it for this course, or use a Docker container with legacy PHP 7 instance to run the code from the downloaded resources.
Well, there's another 3rd option - you can install the latest new Symfony version and try to follow this course on it, i.e. ignoring downloading the course code here. We left some notes in this course that should help you follow this course on a newer version of Symfony and so on the newer PHP version, but if you get stuck somewhere following it on a newer Symfony version - feel free to leave a question below the video and our support team will help you as soon as we can!
So, choose the best path for you. I hope this helps!
Cheers!
root@Administrator:/var/www/Bundle# composer dump-autoload
Do not run Composer as root/super user! See https://getcomposer.org/root for details
Continue as root/super user [yes]?
Generating autoload files
Generated autoload files
root@Administrator:/var/www/Bundle#
namespace go\goBundle;
/**
* Generate random "lorem ipsum" text KnpUniversity style!
*
* @author Ryan Weaver <ryan@knpuniversity.com>
*/
class KnpUIpsum
{
private $unicornsAreReal;
private $minSunshine;
public function __construct(bool $unicornsAreReal = true, $minSunshine = 3)
{
$this->unicornsAreReal = $unicornsAreReal;
$this->minSunshine = $minSunshine;
}
}
Still Error:
(1/1) RuntimeException
Cannot autowire service "App\Controller\ArticleController": argument "$knpUIpsum" of method "__construct()" has type "go\goBundle\knpUIpsum" but this class was not found.
in DefinitionErrorExceptionPass.php (line 37)
at DefinitionErrorExceptionPass->processValue(object(Definition), true)
in AbstractRecursivePass.php (line 60)
Hey Jamey,
Please, try to clear the cache just in case, it might be just a cache problem. If the problem persist after the cache clear, please, double-check the paths and namespaces, make sure they are consistent and match each other.
Cheers!
Cannot autowire service "App\Controller\ArticleController": argument "$knpUIpsum" of method "__construct()" has type "go\goBundle\knpUIpsum" but this class was not found.
In Composer.json
"autoload": {
"psr-4":{
"go\\goBundle\\": "libsss/LoreBundle/src/" ,
"App\\": "src/"
}
},
Hey Jamey-C,
Make sure you have the correct namespace for that knpUIpsum
class, it seems like it should have namespace go\goBundle;
line there. Also, double-check the letter register. And double-check the path, LoreBundle
or LoremBundle
? Might be a possible typo because usually it's "lorem"
Cheers!


Hi guys, I have an issue with php bin/console server:run
I get the next error.
PHP Fatal error: Uncaught LogicException: Symfony Runtime is missing. Try running "composer require symfony/runtime". in /home/user/my_project/bin/console:8
Stack trace:
#0 {main}
thrown in /home/user/my_project/bin/console on line 8
After running composer require symfony/runtime
I get this one.
Problem 1
- Root composer.json requires nexylan/slack-bundle ^2.0,<2.2, found nexylan/slack-bundle[v2.0.0, v2.0.1, v2.1.0] but the package is fixed to v2.2.1 (lock file version) by a partial update and that version does not match. Make sure you list it as an argument for the update command.
Problem 2
- Root composer.json requires symfony/runtime ^4.0, found symfony/runtime[v5.3.0-BETA1, ..., 5.4.x-dev, v6.0.0-BETA1, ..., 6.3.x-dev] but it does not match the constraint.
Use the option --with-all-dependencies (-W) to allow upgrades, downgrades and removals for packages currently locked to specific versions.
Hey @Oleg!
Unfortunately, the actual code behind this tutorial is getting a bit old :/. However, I'm not sure where your problem came from. If I download the course code from this page and run bin/console
, I do not get the error about symfony/runtime
. The bin/console
file is older than symfony/runtime
and doesn't use it... so I'm not sure why your version is trying to use it. Are you using the code download from this course? Or something different?
Cheers!


I have starting code modified for Symfony 5.4 - as the code is not available without subscription my repo is private. At least roughly looks working - anyone from SymfonyCast interested?
Hey Ldath,
Well done! And thank you for your interest in SymfonyCasts tutorials! Yeah, this course is based on Symfony 4, but concepts that we're teaching in this course are still valid for Symfony 5 and 6. Unfortunately, we don't have any plans to upgrade this tutorial to Symfony 5.4 yet, but it may change in the future :)
Cheers!


OK, but isn't a good idea to at least have staring code zipped for the Symfony 5.4 as an option to upload or you are expecting everyone going through painful process of upgrading code which was prepared for 4.0 and even have problem working with older lts 4.4 as far as I can see in the comments?
If you will approve I can just made my repository public and put link here as an comment but like I mentioned as the even existing version needs you to pay to download I do not know if doing this is even legal.
Best Regards
Hey Arkadiusz T.!
> OK, but isn't a good idea to at least have staring code zipped for the Symfony 5.4 as an option to upload or you are expecting everyone going through painful process of upgrading code which was prepared for 4.0 and even have problem working with older lts 4.4 as far as I can see in the comments?
For better or worse, if you're coding along, we expect you to not upgrade anything, but to code along with the version of Symfony that's in the download. It's a tough problem: if we provide upgraded, zipped code, then some of it won't match the video. It just becomes a mess.
> If you will approve I can just made my repository public and put link here as an comment but like I mentioned as the even existing version needs you to pay to download I do not know if doing this is even legal.
This is *perfectly* ok... and it would be awesome! We do put the code download behind the pay wall, but all of our code is technically open and available on GitHub - e.g. https://github.com/knpunive...
Cheers!


OK I can understand this reason.
Here is a link: https://gitlab.com/ldath-co...
Awesome, thanks!
Guys, what made you choose to put our main code inside *src/* instead of using *bundle/* or *lib/ folder* ? Why most of the open source Symfony bundles use *src/* to put the main code of the package! Is it a good practice ? I know that there's a story of *bundle-less, but we apply it of the project not of a sharable bundle.
Could someone explain to me why it's recommended to put the code under src/ ? Is it a psr recommendation. Does there a documentation reference explain this point of vue.
Best regards!
Cheers!
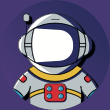
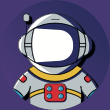
Yes, there is pds/skeleton standard https://github.com/php-pds/...
Hey ahmedbhs!
> what made you choose to put our main code inside *src/* instead of using *bundle/* or *lib/ folder* ?
Hmm, I don't really know :p. What I mean is, src/ and lib/ are really identical choices - I'm not sure why src/ seems to be more popular, at least in "our world". But both lib/ and src/ are fine, but src/ seems to be more popular.
About using bundle/... it probably doesn't make sense if your entire library IS a bundle (why have the extra subdirectory)? However, some pure libraries will come with a Symfony bundle embedded inside of them, and they *will* follow some convention like this. API Platform is one example: it is just a PHP library, but it has a bundle inside of it -
But mostly, there is no technical advantage or disadvantage of how you structure your bundle. Everyone is just trying to find a structure that feels nice and then standardize around that. Actually, most older bundle just put their code right at the root of the repository - e.g. https://github.com/knplabs/... - while newer bundles use the src/ structure - e.g. https://github.com/symfony/...
The reason was kind of to make the bundle look more like an application - with your php source in src/, tests in tests/ and, if you have them, templates in templates/ and so on. That consistency is nice.
Cheers!
Hey weaverryan thank you so mush I really appreciate your time, your explication. Every thing is clear for me.
Just one last function, for a reusable bundle, is it better to make properties private or protected, for example if we inject the entity manger service: protected $entityManger ?
Hey Jeremy,
It always depends if someone will need to override those properties or get access to them. But idea to start with private is always a good one. If you realize later that you need to give your users access to some properties - you can easily make them protected without possible BC breaks. But the reverse will be difficult to do, i.e. when you realize that users should not have access to that property and decided to make it private instead of protected - some users may already use those properties and it will be BC break for them.
Anyway, I think it's always a good idea to start defensively with private and give users access to things they really needed, but look at your code architecture of course, you may have valid cases for making things protected.
Cheers!
Cool this is so clear, Thank you so mush. Just one last question If you don't mind, I aways see "BC breaks" but I fact I didn't understand it. Sory :( Even when I submit pull request on symfony code, I always see BC, I thought that about "breaking something" and I always say NO and I'm not sure about the definition.
Cheers!
Hey Jeremy!
Haha, no problem! I made a wild guess you're already familiar with it :) Let me explain it a bit more. Actually, you did a good guess on this, that's about breaking something. First of all BC is stand for "Backward compatibility" term, you probably heard about it. So, BC breaks means that new changes you're going to add may brake existing code other devs already have in their projects. E.g. you initially called PHP class as MyService and then renamed it to AppService - that's a completely different name and if some devs already use your old code and upgraded to the new changes - their code will be broken unless they renamed old class to the new one in all over their project. I hope this explanation is clear for you, but that's just a one of possible examples, it's not only about class names.
And btw, you may probable know that Symfony has BC promise - you can read about it here: https://symfony.com/doc/cur... . That's mean that if you upgrade minor version of it - you will get new features but nothing in your code should be broken. That's why Symfony so care about possible BC breaks and if some changes require BC breaks - they should be included in major releases only or requires some additional legacy changes to avoid BC breaks.
Cheers!


Can someone please help me the sourcecode for download please.
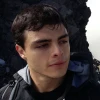
Hey Talent C.
You can download the course code by clicking on the "Download -> Course Code" button at the top right corner of this page
Cheers!
Just in case you run into the same. I got the error 'curl_multi_setopt(): CURLPIPE_HTTP1 is no longer supported' when i execute 'composer install'.
Following fix helped me: https://github.com/symfony/...
Hi,
I have to make a web application that allows to have several modules with different functionalities, this is because certain clients will have access to certain modules. In symfony 4 would this be done by creating bundles? or is there any other way to do it?
Thanks, I await your response.
Hey Luis,
In Symfony, bundles are mostly meant to be just reusable modules, i.e. some part of code that you can share between a few projects. For example, open source bundles that do some useful things are a good example of this, like KnpSnappyBundle that bring PDF generation into your project. So, instead of implementing this yourself you just can install a ready-to-use solution, i.e. bundle. But if you're not going to have a few projects where you want to share some code between them - you would not be interested in bundles. I'd recommend you to just implement it in your project using PHP logic and using Symfony instruments (components) etc.
Cheers!
Thank you very much for the recommendation!
It helped me clarify the concept of bundle and I already know how to implement my project.
Hey Luis,
Perfect! I'm really happy to hear it :)
Cheers and good luck with your project!


i'm using php 7.4, composer 1.9.1 and symfony cli 4.10.2 on microsofts linux sub system at the moment. and i have to update the doctrine/annotations package and the framework-extra-bundle
perhaps you can add "version": "v1.8.0" to your composer.lock files, this fixes the problem for the annotations to fix the issue for most people.
1st: composer install (error message about annotation)
2nd: composer update doctrine/annotations
3rd: composer update sensio/framework-extra-bundle
i updated the framework bundle to fix some issues in productiv envoirment
and 1 deprecated message can be eliminated easily, remove symfony/lts, this is not needed if using flex
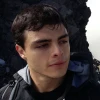
Hey Maik T.
Thanks for the detailed instructions. Can you tell me what error were you having?
Cheers!


Executing script cache:clear [KO]<br /> [KO]<br />Script cache:clear returned with error code 1<br />!!<br />!! // Clearing the cache for the dev environment with debug<br />!! // true<br />!!<br />!!<br />!! In FileLoader.php line 168:<br />!!<br />!! Notice: Trying to access array offset on value of type null in /mnt/d/proje<br />!! cts/php/learning/symfony4/test/config/routes/../../src/Controller/ (which i<br />!! s being imported from "/mnt/d/projects/php/learning/symfony4/test/config/ro<br />!! utes/annotations.yaml"). Make sure annotations are installed and enabled.<br />!!<br />!!<br />!! In DocParser.php line 995:<br />!!<br />!! Notice: Trying to access array offset on value of type null<br />!!<br />!!<br />!! cache:clear [--no-warmup] [--no-optional-warmers] [-h|--help] [-q|--quiet] [-v|vv|vvv|--verbose] [-V|--version] [--ansi] [--no-ansi] [-n|--no-interaction] [-e|--env ENV] [--no-debug] [--] <command><br />!!<br />!!<br />Script @auto-scripts was called via post-install-cmd
And at least the command composer update doctrine/annotations
fixes the problem.
Hey Maik,
It was a small BC break from the new PHP version in case some code abuses some extra check on array. Actually it comes from "doctrine/annotations", but in 1.7 they fixed it, see related issue: https://github.com/doctrine...
So, basically, you have two options: Downgrade your PHP version to 7.3 or continue using 7.4 but you would need to upgrade "doctrine/annotations", I suppose just executing "composer update doctrine/annotations" will do the trick. Make sure the downloaded version is 1.7 or higher in the console output.
I hope this helps!
Cheers!
Hi,
When I start the server, I've got the following errors :
PHP Warning: Unknown: failed to open stream: No such file or directory in Unknown on line 0
PHP Fatal error: Unknown: Failed opening required 'D:\XXXXX\symfony-bundle\vendor\symfony\web-server-bundle/Resources/router.php' (include_path='C:\xampp\php\PEAR') in Unknown on line 0
PHP Warning: Unknown: failed to open stream: No such file or directory in Unknown on line 0
PHP Fatal error: Unknown: Failed opening required 'D:\XXXXX\symfony-bundle\vendor\symfony\web-server-bundle/Resources/router.php' (include_path='C:\xampp\php\PEAR') in Unknown on line 0
The same config for my others Symfony projects is OK.
I tried to update the project "composer update" (one warning, symfony/lts is abandoned) but then "cache:clear" command failed :
Circular reference detected for service "nexy_slack.client", path: "nexy_slack.client -> Nexy\Slack\Client -> nexy_slack.client".


in composer.json say nexy slack client has to be version 2.1.0
"nexylan/slack-bundle": "^2.0<2.2",
the problem with the slack message is the new slack client that works a little bit different an is not compatible to the course codes
Hey Maik,
Thanks for this tip! Yes, we're recommending to do "composer install" to bootstrap the project, that's what we're saying in the README when you download the course code. But if you do "composer update" instead - it might install some newer versions that is not quite compatible out of the box and more actions need from your side.
Cheers!
Hey AmalricBzh!
Hmm, interesting - don't like that at all! Do you get this error when using the start/
directory of the course download from this page? Or something different? Also, you might try manually deleting the vendor/
directory then re-running composer install
. This is a weird error... because it looks like this one file (router.php) is... simply (mysteriously!) missing.
Let me know!
Cheers!
I cloned the repository
https://github.com/knpunive... from where I found this
page. Since that, I deleted the project and I followed the tutorial by
implementing a bundle in my own project (and it works well).
Now I just retry (clone + composer install + symfony serve) and.. it's OK ! Sorry for this false error...
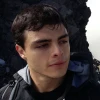
Dang! That was strange but I'm happy to hear that it's working now. Let's keep coding!


Hi SymfonyCasts team (or any users who stumble upon this thread before the code is updated) after downloading the code and trying to run `composer install` as per the instructions, I got the following error:
```
[ErrorException]
Declaration of Symfony\Flex\ParallelDownloader::getRemoteContents($originUrl, $fileUrl, $context) should be compat
ible with Composer\Util\RemoteFilesystem::getRemoteContents($originUrl, $fileUrl, $context, ?array &$responseHeade
rs = NULL)
```
Running `composer update symfony/flex --no-plugins` and then `composer install` fixes the problem.
security-checker also gave a 'ko' for known vulnerabilities in symfony/http-foundation (v4.0.6), though doesn't stop the project from installing.
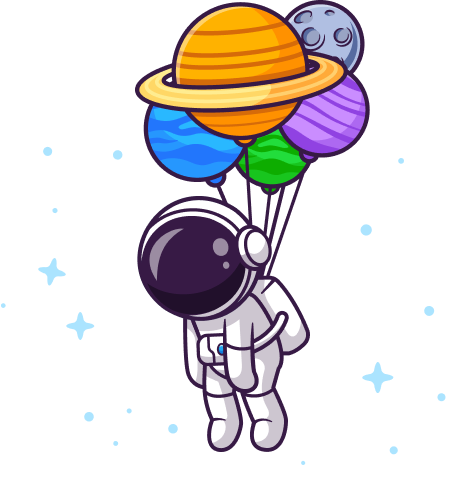
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": "^7.1.3",
"ext-iconv": "*",
"doctrine/annotations": "^1.8", // v1.8.0
"knplabs/knp-markdown-bundle": "^1.7", // 1.7.0
"knpuniversity/lorem-ipsum-bundle": "*@dev", // dev-master
"nexylan/slack-bundle": "^2.0,<2.2", // v2.0.1
"php-http/guzzle6-adapter": "^1.1", // v1.1.1
"sensio/framework-extra-bundle": "^5.1", // v5.1.6
"symfony/asset": "^4.0", // v4.0.6
"symfony/console": "^4.0", // v4.0.6
"symfony/flex": "^1.0", // v1.21.6
"symfony/framework-bundle": "^4.0", // v4.0.6
"symfony/lts": "^4@dev", // dev-master
"symfony/twig-bundle": "^4.0", // v4.0.6
"symfony/web-server-bundle": "^4.0", // v4.0.6
"symfony/yaml": "^4.0", // v4.0.6
"weaverryan_test/lorem-ipsum-bundle": "^1.0" // v1.0.0
},
"require-dev": {
"easycorp/easy-log-handler": "^1.0.2", // v1.0.4
"sensiolabs/security-checker": "^4.1", // v4.1.8
"symfony/debug-bundle": "^3.3|^4.0", // v4.0.6
"symfony/dotenv": "^4.0", // v4.0.6
"symfony/maker-bundle": "^1.0", // v1.1.1
"symfony/monolog-bundle": "^3.0", // v3.2.0
"symfony/phpunit-bridge": "^3.3|^4.0", // v4.3.3
"symfony/stopwatch": "^3.3|^4.0", // v4.0.6
"symfony/var-dumper": "^3.3|^4.0", // v4.0.6
"symfony/web-profiler-bundle": "^3.3|^4.0" // v4.0.6
}
}
This tutorial is listed under Symfony 5 when in fact it is a Symfony 3 or 4 tutorial!
Nothing works when the code is installed...