Chapters
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Symfony boasts some of the most epic debugging tools of all the Internet. But because Symfony apps start so small, we don't even have them installed yet. Time to fix that. Head over to your terminal and, like before, commit all of your changes so we can check out what the recipes will do. I already did that.
Installing the Debugging Tools
Then run:
composer require debug
Yup! That's another Flex alias. And... it installs a pack. This installs four different packages that add a variety of debugging goodness to our project. Spin over and open composer.json
.
{ | |
Show Lines
|
// ... lines 2 - 5 |
"require": { | |
Show Lines
|
// ... lines 7 - 14 |
"symfony/monolog-bundle": "^3.0", | |
Show Lines
|
// ... lines 16 - 20 |
}, | |
Show Lines
|
// ... lines 22 - 78 |
} |
Ok, the pack added one new line under the require
key for monolog-bundle
. Monolog is a logging library.
Then all the way at the bottom, it added three packages to a require-dev
section.
{ | |
Show Lines
|
// ... lines 2 - 73 |
"require-dev": { | |
"symfony/debug-bundle": "7.0.*", | |
"symfony/stopwatch": "7.0.*", | |
"symfony/web-profiler-bundle": "7.0.*" | |
} | |
} |
These are known as dev dependencies... which means they won't be downloaded when you deploy to production. But otherwise, they work the same as packages under the require
key. All three of these help power something called the profiler. We'll see that in just a minute.
Before we do, go back to your terminal and run
git status
so we can see what the recipes did. Ok: it updated the normal files, enabled a few new bundles and gave us three new configuration files for those bundles.
What's the end result of all this new stuff? Well, first, we now have a logging library. So, like magic, logs will start popping into a var/log/
directory.
Hello Web Debug Toolbar & Profiler
But the mind-blowing moment happens when we refresh the page. Woh! A beautiful new black bar at the bottom called the web debug toolbar.
This is bursting with info. Over here, we can see the route and controller for this page. That it makes it easy to go to any page on your site - maybe one you didn't even build - and quickly find the code behind it. We can also see how long this page took the load, how much memory it used, and even the twig template that was rendered and how long that took.
But the real magic of the web debug toolbar happens when you click any of these links: you hop into the profiler. This has ten times more info: details about the request and response, logs that occurred while loading that page, routing details, and even stats about which Twig templates were rendered. Apparently six templates were rendering: our main one, the base layout and a few others that power the web debug toolbar, which, by the way, won't be rendered or shown when we deploy to production. But we'll talk about that in the next tutorial.
Then there's probably my favorite section: Performance. This slices our entire page load time into different pieces. I love this. As you learn more about Symfony, you'll get more familiar with what these different pieces are. This section is useful for knowing which part of your code might be slowing down the page... but it's also a fantastic way to dive deeper into Symfony and understand all its moving pieces.
We're going to use the profiler throughout this series, but let's turn to another debugging tool: one that's been installed in our app this whole time!
Hello bin/console!
Head over to the command line and run:
php bin/console
Or, on most machines, you can just say ./bin/console
. This is Symfony's console, and it's packed with commands that can do all sorts of stuff! We'll learn about them along the way. You can also add your own commands, which we'll do at the end of the tutorial.
Notice that a bunch of these start with debug
- like debug:router
. Try that:
php bin/console debug:router
Cool! This shows us every route in our app: the homepage route at the bottom and a bunch of routes added by Symfony in the dev
environment that power the web debug toolbar and profiler.
Another command is debug:twig
:
php bin/console debug:twig
This tells us every Twig function, filter or other thing that exists in our app. This is like the Twig docs... except it also includes extra functions and filters that are added to Twig by bundles that we have installed. Pretty cool.
These debug
commands are super useful, and we'll keep trying more of them along the way.
Next, let's create our first API endpoint and learn about Symfony's powerful serializer component.
26 Comments
Hey @pasquale_pellicani ,
Thanks for this tip! Indeed, if you're using Apache instead of built-in Symfony web server that we're using in the screencast - you would need that symfony/apache-pack
package that will bring an .htaccess
file into your app.
Cheers!
Hello,
I have two questions for this topic:
- I am removing debug by command composer remove debug. But it is still exists. Is there any trick to apply it?
- In my symfony, by default there is debug. but var/log directory has a log.dev file. not direct log file.Is it important or does it depend to version of symfony or something else? I am using ubuntu server and I installed symfony via composer command.
Hi @Mahmut-A
- What do you mean still exist?
- Logging can be configured with monolog bundle
Can you provide more details what exactly you want to achieve?
Cheers
Hi Sadikoff,
- I used composer remove debug command. But all debug package is still in composer.json file. I expected it will be removed from there. Also, debug bar is still seen on top of page. I want to disable it.
- We ignore second question. thank you
Oh, I got it. That is a tricky moment debug
is an installation alias, so once you installed debug pack, you should forget about it, and if you want to uninstall it, you should uninstall each package separately.
Cheers!


Could you also please explain how to remove bundles or packs?
Hey @tomoguchi ,
How to remove? It's as easy as execute composer remove
command. If you use Symfony Flex - it will also remove config files. If no, you will need to do it manually. But basically, you just need to drop the package from your composer.json file, for this you leverage the command I mentioned above: composer remove ...
and then you need to disable the bundle in the config/bundles.php
- just literally drop the related namespace from there. Then clean up any related config files in the config/
dir. I think that's all :)
Cheers!


hello @Victor does that also removes the generated files, setting and autowirings composer require some-bundle
created?
Hey @tomoguchi ,
Yes, it has to remove those files too. Well, not the files you created manually but the files that were created by Symfony Flex recipe. But it will happen only if you have Symfony Flex enabled and allowed in your project.
Cheers!


adding this inside controller method:
$x = '1';
$y = '2';
dump($x);
dump($y);
each use of dump() is adding 2 seconds to execution time. So this takes 4seconds to load the script.
I intend to use it quite a lot to track things down is there a way to make it quicker ?
Hey @John-S, this is indeed odd. To confirm, removing one dump()
reduces the request time to ~2 seconds and adding a dump()
increases to ~6 seconds? Do you see the dump output as expected in the profiler?


1 dump() 2 seconds.
2 dump() 4 seconds.
3 uses of dump() profiler line:
dump 6078.2 ms / 2 MiB
probably something with the settings but just not sure what.
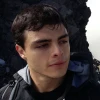
Does it happen on every request? In particular when you don't change the code
Do you experiment the same behavior with a different Symfony app?
I believe you may have added by mistake a sleep()
somewhere in your vendors. Try re-installing them
Cheers!


Is it ok that dump() increases every request time to 2seconds?
I haven't installed the monolog bundle but installed symfony/stopwatch --dev. I have short array on my controller and using dump($simpleArray) on it. It now it takes 2seconds to load every request (dump 2037.7 ms / 2 MiB). Is this ok ?
Hey John,
It depends on the data you're trying to dump. If you're dumping heavy objects, the serialization may really take long time. Also, sometimes it depends if you're using a virtualization tool like Docker or no. With virtualization tools numbers can be really much more increased.
But in short, that's OK, because you should use dump()
/dd()
features only in dev mode and never should use it on production.
Cheers!


hello,
I'm having a hard time following which packages require "--dev" addon.
For example I see both the symfony/debug-bundle on docs and on packagist website recommended installation as "composer require symfony/debug-bundle" but here(composer.json) is under require-dev. My installation settled it under "require".
how to do it right ?
p.s. I know this is introductory tutorial but i really try to install all features individually and not packs so I can keep the track on and know what's what.
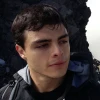
Hey @John-S
To make composer install things under the require-dev
key, you need to pass the --dev
flag. You can also manually move the dependencies from one key to another in case you forgot to add the flag.
Cheers!
Hello,
Could you please show how to remove debug from the bar? I did composer remove debug. but still I see the debug bar.
thank you
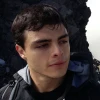
Hey @Mahmut-A
Can I ask why? You're the first one I know who doesn't like it - lol
Seriously, that debug toolbar is only loaded on the "dev" environment. You can remove it by deleting the bundle's entry in config/bundles.php
Cheers!
Hi,
the first reason is actually I wanted to test to remove a package from composer. so when I used composer remove command, composer.json did not delete this package. so I did not want to wrong thing to affect all symfony environment.
the second reason, I am building my project in web service apache. so I do not want show the debug to clients.
then, I understand that if any package is need to remove, I need to remove from config/bundle.php right?
thank you for your help
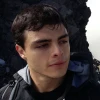
Ok, the bundle you want to remove is this one symfony/debug-bundle
- I recommend not removing it because it only works on the "dev" environment, so your clients won't see it, also, it should be in the require-dev
composer key and those dependencies should not be installed in your production server. You can do that by adding the flag composer install --no-dev
then, I understand that if any package is need to remove, I need to remove from config/bundle.php right?
That's true only for bundles
Cheers!
Hi MolloKhan,
Thank you.
I applied this command: composer install --no-dev but page gives below error:
Attempted to load class "DebugBundle" from namespace "Symfony\Bundle\DebugBundle".<br />Did you forget a "use" statement for another namespace?
because of that, ı added debug again by command composer require debug
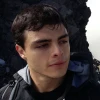
When installing composer deps using the --no-dev
flag your must run your application on the "prod" environment, otherwise it will try to load dev dependencies but those were not installed, hence, your error
Actually, how can we describe prod? Because my project under /var/www/html and it is working as a apache web.
I dont do in localhost.
Because of that, my expectation is when I do this —no-dev command, it should be disabled.
Thank you so much again for your kindly help
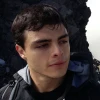
I mean the APP_ENV
environment variable. That should be set to "prod" - I recommend you to watch this video, it will give you a better idea of Symfony environments https://symfonycasts.com/screencast/symfony-fundamentals/environments
Cheers!
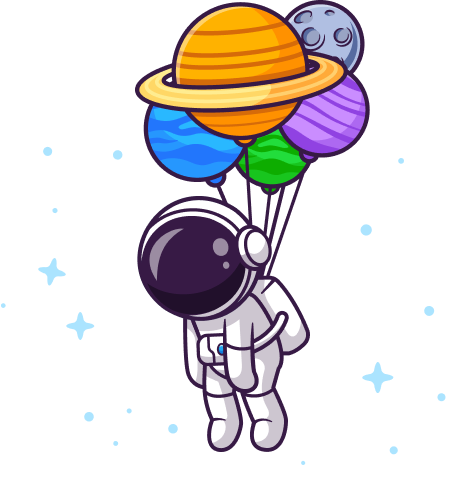
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=8.2",
"ext-ctype": "*",
"ext-iconv": "*",
"php-cs-fixer/shim": "^3.46", // v3.46.0
"phpdocumentor/reflection-docblock": "^5.3", // 5.3.0
"phpstan/phpdoc-parser": "^1.25", // 1.25.0
"symfony/asset": "7.0.*", // v7.0.3
"symfony/asset-mapper": "7.0.*", // v7.0.2
"symfony/console": "7.0.*", // v7.0.2
"symfony/dotenv": "7.0.*", // v7.0.2
"symfony/flex": "^2", // v2.4.3
"symfony/framework-bundle": "7.0.*", // v7.0.2
"symfony/monolog-bundle": "^3.0", // v3.10.0
"symfony/property-access": "7.0.*", // v7.0.0
"symfony/property-info": "7.0.*", // v7.0.0
"symfony/runtime": "7.0.*", // v7.0.0
"symfony/serializer": "7.0.*", // v7.0.2
"symfony/stimulus-bundle": "^2.13", // v2.13.3
"symfony/twig-bundle": "7.0.*", // v7.0.0
"symfony/ux-turbo": "^2.13", // v2.13.2
"symfony/yaml": "7.0.*", // v7.0.0
"symfonycasts/tailwind-bundle": "^0.7.1", // v0.7.1
"twig/extra-bundle": "^2.12|^3.0", // v3.8.0
"twig/twig": "^2.12|^3.0" // v3.8.0
},
"require-dev": {
"symfony/debug-bundle": "7.0.*", // v7.0.0
"symfony/maker-bundle": "^1.52", // v1.53.0
"symfony/stopwatch": "7.0.*", // v7.0.0
"symfony/web-profiler-bundle": "7.0.*" // v7.0.2
}
}
I add a suggestion that can avoid wasting an hour of attempts to make the call to the _wdt route work!
install this, after composer require debug command:
composer require symfony/apache-pack