Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Adding a Flash Message
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
Adding a Flash Message¶
After registration, let’s make the new user feel loved by giving them a big happy success message! Symfony has a feature called flash messages, which is perfect for this. A flash is a message that we set to the session, but that disappears after we access it exactly one time.
After registration, grab the session object from the request and get an object called a “flash bag”. Set a message on it using add:
// src/Yoda/UserBundle/Entity/Controller/RegisterController.php
// ...
if ($form->isValid()) {
// .. code that saves the user
$request->getSession()
->getFlashBag()
->add('success', 'Welcome to the Death Star, have a magical day!')
;
$url = $this->generateUrl('event');
return $this->redirect($url);
}
Rendering a Flash Message¶
That’s it! Now, if a flash message exists, we just need to print it on the page. Let’s do that in base.html.twig. The session object is available via app.session. Use it to check to see if we have any success flash messages. If we do, let’s print the messages inside a styled container. You’ll typically only have one message at a time, but the flash bag is flexible enough to store any number:
{# app/Resources/views/base.html.twig #}
<body>
{% if app.session.flashBag.has('success') %}
<div class="alert alert-success">
{% for msg in app.session.flashBag.get('success') %}
{{ msg }}
{% endfor %}
</div>
{% endif %}
<!-- ... -->
The success key is just something I made up. With this setup, whenever we need to show a happy message, we just need to set a success flash message and it’ll show up here!
Let’s test it out. We register, the flash message is set, and then it’s displayed after the redirect. Nice!
7 Comments
Hey Zuhayer Tahir!
Hmm, it should totally be possible. In fact, it looks like they should support "success", "warning" and "danger" types out of the box. Basically, they already render flash messages on their layout for certain flash "types", and you can configure it further: https://sonata-project.org/... (the "domain" refers to the translation domain to use for the flash message, if you care).
So basically, as far as I can see, it should already work! But, are you not seeing that?
Cheers!
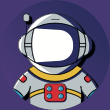
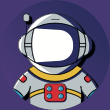
No I am not seeing the flash messages.
In my symfony controller the FlashBag has all the flash messages in queue:
https://pastebin.com/raw/Ty...
$request->getSession()->getFlashBag()->add('success', 'Welcome to the Death Star, have a magical day!');
dump($this->get('session'));
Session dump in Sonata (Entity)Admin Class:
https://pastebin.com/raw/iY...
But in Sonata admin neither the flash message is shown, nor the flash bag is emptied.
In Sonata admin I am browsing different pages to see if the flash messages are shown.
I also have used this code in custom dashboard twig file, but flash messages still not shown:
{% if app.session.flashBag.has('success') %}
<div class="alert alert-success">
{% for msg in app.session.flashBag.get('success') %}
{{ msg }}
{% endfor %}
</div>
{% endif %}
P.s. I have also tried:
$this->get('session')->getFlashBag()->add('sonata_flash_error', 'Welcome to the Death Star, have a magical day!!');
$this->get('session')->getFlashBag()->add('sonata_flash_success', 'Welcome to the Death Star, have a magical day!!');
But not joy.
Hey Zuhayer Tahir!
Hmmm. It's interesting that your second dump - from Sonata (Entity)Admin Class, does not contain the flash messages. It's also very interesting that the flash bag is not emptied when using Sonata. And it is *especially* interesting that adding code to the custom dashboard twig file *also* doesn't cause the messages to be shown. Honestly, it makes me think something very strange is happening! If you try to dump the session *anywhere* in SonataAdminBundle (e.g. in a template, hacked temporarily into a controller, etc) - do you *ever* see the flash messages?
Cheers!
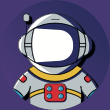
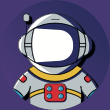
Project requirement changed. So leaving this issue. Thanks for the responses.
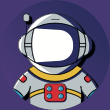
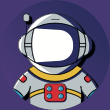
Hi Knp,
I hope you could help me with my flashbag issue.
I'm new with Symfony and I'm using FOSRESTBUNDLE in my sample project. I can't seem to get the flashbag to display.
Here is my code:
Controller
public function postGenderAction(Request $request)
{
$form = $this->newGenderAction();
$form->handleRequest($request);
$newGender = $form->getData();
$form->getErrorsAsString(true);
if ($form->isValid()) {
$em = $this->getDoctrine()->getManager();
$em->persist($newGender);
$em->flush();
// Redirect to the list of genders.
$nextAction = $form->get('saveAndAdd')->isClicked() ? 'api_1_new_gender' : 'api_1_get_genders';
// Adds a flashbag message.
$request->getSession()->getFlashbag()
->add('success', 'The new gender has been created successfully!');
$routeOptions = array(
'id' => $newGender->getId(),
'_format' => $request->get('_format')
);
return $this->routeRedirectView($nextAction, $routeOptions, Codes::HTTP_CREATED)
;
}
Twig
{#Flashbag#}
{% if app.session.flashbag.has('success') %}
<div class="alert alert-success" role="alert">
{% for msg in app.session.flashbag.get('success') %}
{{ msg }}
{% endfor %}
</div>
{% endif %}
Thanks in advance!
Hi Mel!
It looks ok to me! It's possible that something else is "consuming" your flash messages, which will erase them from the flash. One first tip is that if you click the web debug toolbar to go into the profiler, then you click into the Request tab, you can see a list of the flash messages that Symfony knows about.
Here's what I'd do to debug:
1) Add a var_dump($request->getSession()->getFlashbag()->get('success'));die; in your controller code that you pasted above right after you set the flash message. I'm pretty sure this will return the message, but let's just make sure nothing strange is happening.
2) Remove the above debug code, then put it in whatever controller you're redirecting to. Is it there? If it is, the nit *is* successfully being transferred across requests, but then something else might be reading/clearing it when you read the template.
3) Try a key other than "success" to see if that changes anything.
I'm sure it's something small - this is one of those "it just kinda works" type of features :).
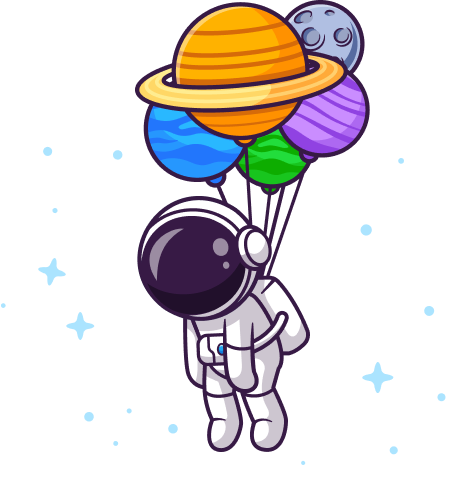
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1" // 4.1.0
}
}
Is it possible to add a success flash message in symfony controller and show it in Sonata Admin?
e.g.
I add a flash msg in DefaultController.php
$request->getSession()->getFlashBag()->add('success', 'Welcome to the Death Star, have a magical day!');
Or $this->get('session')->getFlashBag()->add('success', 'User had done a good thing');
and be able to get show it in Sonata admin as regular flash message?
Result: https://ibin.co/3TKAP5ofgPX...