Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Handling Form Submissions
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
Handling Form Submissions¶
Ok, let’s get this form to actually submit! Since we’re submitting back to the same route and controller, we want to process things only if the request is a POST.
Getting the Request object¶
First, we’ll need Symfony’s Request object. To get this in a controller, add a $request argument that’s type-hinted with Symfony’s Request class:
// src/Yoda/UserBundle/Controller/RegisterController.php
// ...
use Symfony\Component\HttpFoundation\Request;
class RegisterController extends Controller
{
// ...
public function registerAction(Request $request)
{
// ...
}
}
Normally, if you have an argument here, Symfony tries to populate it from a routing wildcard with the same name as the variable. If it doesn’t find one, it throws a giant error. The only exception to that rule is this: if you type-hint an argument with the Request class, Symfony will give you that object. This doesn’t work for everything, only the Request class.
Using handleRequest¶
Use the form’s handleRequest method to actually process the data. Next, add an if statement that checks to see if the form was submitted and if all of the data is valid:
// src/Yoda/UserBundle/Controller/RegisterController.php
use Symfony\Component\HttpFoundation\Request;
// ...
public function registerAction(Request $request)
{
$form = $this->createFormBuilder()
// ...
->getForm()
;
$form->handleRequest($request);
if ($form->isSubmitted() && $form->isValid()) {
// do something in a moment
}
return array('form' => $form);
}
The handleRequest method grabs the POST’ed data from the request, processes it, and runs any validation. And actually, it only does this for POST requests so on a GET request, $form->isSubmitted() returns false.
Tip
If you have a form that’s submitted via a different HTTP method, set the method.
If the form is submitted because it’s a POST request and passes validation, let’s just print the submitted data for now. If the form is invalid, or if this is a GET request, it’ll just skip this block and re-render the form with errors if there are any:
if ($form->isSubmitted() && $form->isValid()) {
var_dump($form->getData());die;
}
Time to test it! We haven’t added validation yet, but the password fields have built-in validation if the values don’t match. When I submit, the form is re-rendered, meaning there was an error.
In fact, there’s now a little red box on the web debug toolbar. If we click it, we can see details about the form: what was submitted and options for each field.
Head back and fill in the form correctly. Now we see our dumped data:
array(
'username' => string 'foo' (length=3),
'email' => string 'foo@foo.com' (length=11),
'password' => string 'foo' (length=3),
)
Using the Submitted Data Array¶
Notice that the data is an array with a key and value for each field. Let’s take this data and build a new User object from it. There is an easier way to do this, and I’ll show you in a second:
// src/Yoda/UserBundle/Controller/RegisterController.php
use Yoda\UserBundle\Entity\User;
// ...
$form->handleRequest($request);
if ($form->isSubmitted() && $form->isValid()) {
$data = $form->getData();
$user = new User();
$user->setUsername($data['username']);
$user->setEmail($data['email']);
}
Encoding the User’s Password¶
We still need to encode and set the password. For now, let’s copy in some code from our user fixtures to help with this. We’ll make this much more awesome in the next screencast:
// src/Yoda/UserBundle/Controller/RegisterController.php
// ...
private function encodePassword(User $user, $plainPassword)
{
$encoder = $this->container->get('security.encoder_factory')
->getEncoder($user)
;
return $encoder->encodePassword($plainPassword, $user->getSalt());
}
Use this function, then finally persist and flush the new User:
// src/Yoda/UserBundle/Controller/RegisterController.php
// ...
if ($form->isValid()) {
$data = $form->getData();
$user = new User();
$user->setUsername($data['username']);
$user->setEmail($data['email']);
$user->setPassword($this->encodePassword($user, $data['password']));
$em = $this->getDoctrine()->getManager();
$em->persist($user);
$em->flush();
// we'll redirect the user next...
}
Redirecting after Success¶
The last step of any successful form submit is to redirect - we’ll redirect to the homepage. First, we need to generate a URL - just like we do with the path() function in Twig. In a controller, there’s a generateUrl function that works exactly the same way:
// src/Yoda/UserBundle/Controller/RegisterController.php
// ...
if ($form->isSubmitted() && $form->isValid()) {
// ...
$em->flush();
$url = $this->generateUrl('event');
}
To redirect, use the redirect function and pass it the URL:
if ($form->isSubmitted() && $form->isValid()) {
// ...
$url = $this->generateUrl('event');
return $this->redirect($url);
}
Tip
If you use Symfony 2.6 or newer, you have a redirectToRoute method that allows you to redirect based on the route name instead of having to generate the URL first. It’s a shortcut!
Remember that a controller always returns a Response object. redirect is just a shortcut to create a Response that’s all setup to redirect to this URL.
Ok, time to kick this proton torpedo! As expected, we end up on the homepage. We can even login as the new user!
You Don’t Need isSubmitted¶
Head back to the controller and remove the isSubmitted() call in the if statement:
// src/Yoda/UserBundle/Controller/RegisterController.php
$form->handleRequest($request);
if ($form->isValid()) {
// ...
}
This actually doesn’t change anything because isValid() automatically returns false if the form wasn’t submitted - meaning, if the request isn’t a POST. So either just do this, or keep the isSubmitted part in there if you want - I find it adds some clarity.
80 Comments
Hey Ganesh!
> How Can i check website is secure or not?
You can use Security Checker, see https://symfony.com/doc/cur... . Or you can upload your composer.lock file here to check it: https://security.symfony.com/
> My files are indexing google... How to Remove this one?
If you don't want to index some links - you need to disallow it in robots.txt file in your public/ directory. You can see this link for more info: http://www.robotstxt.org/ - but it's just a recommendation and robots might ignore it. You can go to Google Webmasters Tools and you'll find "Remove URLs" page where you can ask Google do not index some pages. But it needs time to apply.
Also, I'd recommend you to create a sitemap and specify link to it in that robots.txt file like:
Sitemap: https://example.com/sitemap...
I hope this helps!
Cheers!
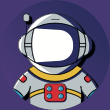
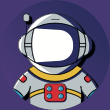
I have one doubt for htaccess
My Url is
http://localhost/example/web/Profile?user=ganesh&id=156
i Just want to change url for "http://localhost/example/web/ganesh/156/"
My htacess code is
RewriteRule ^([A-Za-z0-9-]+)/([0-9]+)/?$ Profile?user=$1&id=$2 [NC,L]
this is not working !
how can i solve this problem ?
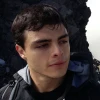
I'm not sure how to achieve it via htaccess, there must be some good docs about it but I don't like that way. I recommend you to use Symfony Router component, setting up pretty URL's is really simple
Cheers!
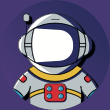
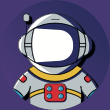
Hi Ryan,
I am working with Symfony Forms and jQuery sortable and ran into a weird issue, I was hoping to get your expertise as I am out of all solutions.
I have a form (it is a collection form) and inside that form a user can change the priority of their goals, to set the priority from top to bottom I am using jQuery sortable, i am able to send the sort order to controller just fine via ajax and I am able to save the data in db just fine as well. After completing the task of sorting and all, i reloaded the page and saw even though the sort order is saved in database but the goals are actually not being displayed in the correct order, I thought this should be easy, I already have relationship setup so an `orderBy` should solve this but this is where the problem started.
Here is the link to the yml file where i added the `orderBy`
https://gist.github.com/sha...
So after doing that as long as I do not sort the form, data gets saved just fine, but if i sort and then save i see the following error
Neither the property "id" nor one of the methods "addId()"/"removeId()", "setId()", "id()", "__set()" or "__call()" exist and have public access in class "MyBundle\Entity\Goals".
Why am I seeing this error? the only reason i am making use of Goal Entity Id is so that I know what sort order to assign to which id. https://gist.github.com/sha...
Is it possible to display the correct order from database without using orderBy in this case? At this point I am pretty clueless as to what I am doing wrong. Any help will be really appreciated.
Hey Shairyar!
Yes, this is tricky: creating new Goals and allowing them to be re-ordered all at once. Typically, I avoid the collection type when things get this complicated and instead save things via AJAX (e.g. the moment you create a new goal, I save it. The moment you re-order, I save that, etc). A few things:
1) The getId/setId() error is coming directly from you having an "id" field on your GoalType form. You should remove this, which makes sense - you don't really want the id of the Goal being changed by the user :).
2) Very clever solution to set the orderBy - that looks good to me. I'm not sure why setting that would suddenly cause issues with sorting, however. The collection type isn't really built to handle ordering, it's possible that you could find some weird behavior with this. For example, I *think* if it lists 3 goals on the page, and you submit 3 goals, it updates the first goal in the DB with the data from the first submitted goal, etc. In other words, I think if you had 2 goals and you re-ordered them, the first would be updated with the second's data and the second with the first's data. Does that makes sense? In other words, changing the order might be causing weird issues - which is why there's no native support with "ordering" things with the collection type.
Let me know if this helps. You're in a complicated area, and my best advice is to consider simplifying by not using the collection type :).
Cheers!
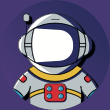
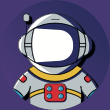
Hi Sir I am fresher in symfony.. I Have one doubt..How get id from controller redirecting new page to display that ID. Then i will Update current value and then new feild also update that form..
Hey Ganesh,
Could you explain a bit more what you're trying to do with a little example? If you wonder how to get an ID in a controller - the most common way is getting that ID from URL, e.g. when you request a page like "/blog/25", you're requesting a post which ID is "25", so if you have a Symfony route like "/blog/{postId}", then you can request "$postId" variable in the method to get this ID:
/**
* @Route("/blog/{postId}")
*/
public function showPostAction($postId)
{
dump($postId); die; // where $postId holds that ID, i.e. "25" in my example
}
I hope this example helps you.
Cheers!
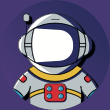
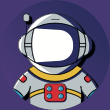
Thank You Victor
I have to display current id get and then display for that all row content with including update option
For Example
<-- Controller Page -->
public function JobseekerAction(Request $request) {
$candidate = $this->getDoctrine()->getRepository('CandidateCandidateBundle:Candidateprofile')->findAll();
dump($candidate);
return $this->render('HomeBundle:Default:welcome.html.twig', ['candidate' => $candidate]);
}
<-- View Page -->
{% for candidate in candidate %}
<form id="form" method="POST" action="{{path('welcome_homepage')}}">
</form>
% endfor %}
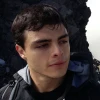
Hey Ganesh Lemoius
If I understood correctly, what you want to do is to show a candidate's information based on the given ID. If I'm right you can do something like this:
/**
* @Route("/candidates/{id}", name="candidate_show")
*/
public function showAction(Candidate $candidate, Request $request)
{
return $this->render('HomeBundle:Default:welcome.html.twig', ['candidate' => $candidate]);
}
This code makes use of the ParamConverter, so if the ID exists, you will get a candidate object, if it's not, a 404 error will be shown
Cheers!
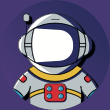
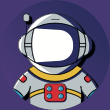
thank you @diego
Now it is working...
How Can I Create Rest Api json for Web App...
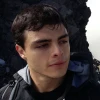
Hey Ganesh Lemoius
That's a great topic, you can find a lot of information, tricks, and useful tools, in our tutorials about RESTful API's https://knpuniversity.com/t...
Cheers!
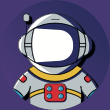
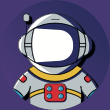
thank u @MolloKhan
i don't have to get current id from new page.
how can i change url for current user ?
For Example
https://knpuniversity.com ---> this is for current login page
https://knpuniversity.com/ (current id) new update page
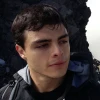
Oh, if getting the current logged in user is what you are after for, inside a controller you can do this:
// some controller.php
public function someAction()
{
$user = $this->getUser(); // If this method does not return null, then you got the logged in user
}
Cheers!
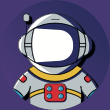
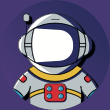
$user = $this->getUser(); this code is not working MolloKhan
I Want display current user for twig file...
FindAll() -> dispplay all rows
How can i get current inserting row ?
Hey Ganesh,
If you want to get the current user in Twig, you can use "{{ app.user.email }}". Or you can pass "$this->getUser()" into Twig templates when render them:
$user = $this->getUser();
$this->render('template-name.html.twig', [
'user' => $user,
]);
And then you can call just "{{ user.email }}"
Cheers!
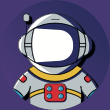
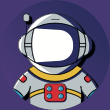
thank u victor
You are not allowed to access this file. Check app_dev.php for more information.
This error come online how to rectify this error
Hey Ganesh Lemoius!
Are you using a Virtual machine or developing on a server that is not your local computer? If so, you should open the web/app_dev.php
file and (temporary) comment out the big if block: https://github.com/symfony/symfony-standard/blob/d3e21e3394a84a61aa20a27ac406cf618703feeb/web/app_dev.php#L13-L19. This is a security mechanism to make sure nobody can access your dev environment on production. If you're developing from a non-local machine, you'll need to comment this out to access your dev environment.
Cheers!
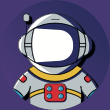
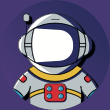
thank u weaverryan
Hey Ganesh Lemoius!
Hmm, it sounds like your version of Symfony might not be compatible with PHP 7. What version of Symfony are you using? If you're using 2.7.1 or older, you might need to upgrade.
Cheers!
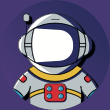
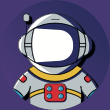
hey weaverryan
symfony upgrade online was possible ?
How can I Do this ?
Hey Ganesh,
What do you mean? You can upgrade your packages with "composer update", but first of all you need to do some tweaks for version constraints in composer.json. But of course there's could be more work. If you're really on Symfony 2.7, then we have a tutorial for you: https://knpuniversity.com/s...
Cheers!
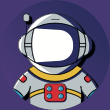
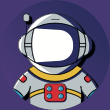
thank u victor
How Can I Create Rest Api json for Web App...
Hey Ganesh,
You can look at our REST track: https://knpuniversity.com/t... - if you're interested in creating REST API app
Cheers!
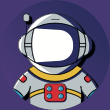
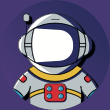
How can i send sms for using otp ? @victor
If U have any example ?
Hey Ganesh,
If the question is only in how to send SMS, then you need to find an SMS service, and most probably it will be a paid service (however some of them may have free limited sms for testing). There're plenty of services who provide sending SMS features via API, but unfortunately I do not know a good one, so can't suggest you something here. But you need to find a service who provide an API and it'd be good if they also have a PHP SDK library with nice docs, so you can able to install it with Composer, register your account on their website, setup your credentials and start using it whenever you need to send SMS.
Cheers!
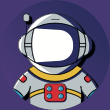
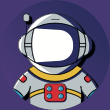
thank u victor
I Completed Otp Concept....
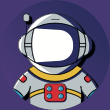
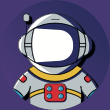
FOSMessageBundle chat application is working for symfony ?
Give me solution for this question..
Hey Ganesh,
Yes, FOSMessageBundle is a popular bundle for Symfony if you're looking for User-to-user messaging. I'm not sure, but probably this bundle is not a kind of chat application where you don't need to refresh the page to see new messages out of the box. To achieve this, you'll need to do some extra work. And unfortunately, it does not have Symfony 4 support yet, see related issue: https://github.com/FriendsO... .
Cheers!
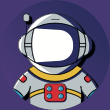
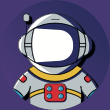
Just i Want to Chat n no of user and n no of admin any reference you have for chat
Hey Ganesh,
Well, if page refreshing is OK for you - I'd recommend to look at FOSMessageBundle, otherwise you can take a look at "cunningsoft/chat-bundle" package which is simple implementation. I have never used it, but looks like it allows you to chat without page refresh. If you're looking for more complex and innovative solution - probably look at NodeJS and NPM packages instead.
Cheers!
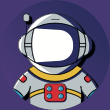
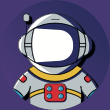
Full authentication is required to access this resource.
500 Internal Server Error - InsufficientAuthenticationException
1 linked Exception: AccessDeniedException »
InsufficientAuthenticationException: Full authentication is required to access this resource.
AccessDeniedException: Must be logged in with a ParticipantInterface instance
Hey Ganesh Lemoius ,
Looks like you have "fos_user" key in your configuration but you don't have FOSUserBundle installed. Or maybe you just didn't enable it in your "app/AppKernel.php" file (or config/bundles.php if you're on Symfony 4). Please, make sure you have FOSUserBundle installed and enabled. Try to check their installation instruction if you needed.
Cheers!
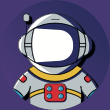
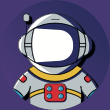
FOS Message bundle only to use our page is possible or not ?
What Purpose to use FOSUserBundle ?
Hey Ganesh,
From their installation page I see:
> Note FOSMessageBundle by default has a requirement for FOSUserBundle for some of its interfaces which can be swapped out if you're not using FOSUserBundle.
So if you do not have FOSUserBundle, look at https://github.com/FriendsO... for more information how to avoid FOSUserBundle. FYI, FOSUserBundle provides login, register, profile show/edit, and reset password features out of the box. We also have a screencast about it: https://knpuniversity.com/s... in case you're interested in it.
Cheers!
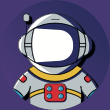
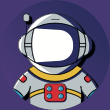
I got this error.
Exception detected!
[Semantical Error] line 0, col 70 near 'tm INNER JOIN': Error: Class FOS\MessageBundle\Entity\Thread has no association named metadata
500 Internal Server Error - QueryException
1 linked Exception: QueryException »
[2/2] QueryException: [Semantical Error] line 0, col 70 near 'tm INNER JOIN': Error: Class FOS\MessageBundle\Entity\Thread has no association named metadata +
[1/2] QueryException: SELECT t FROM FOS\MessageBundle\Entity\Thread t INNER JOIN t.metadata tm INNER JOIN tm.participant p WHERE p.id = :user_id AND t.isSpam = :isSpam AND tm.isDeleted = :isDeleted AND tm.lastMessageDate IS NOT NULL ORDER BY tm.lastMessageDate DESC +
How to solve this error ?
Hey Ganesh,
Looks like a Thread entity is missing metadata. I suppose if you look at Thread::$metadata field - you'll null there. Not sure what exactly you're doing when see this error, but looks like that metadata relation is required in this case. So you need to fill that in with a metadata object, I see it typehinted with "FOS\MessageBundle\Model\ThreadMetadata" class, so I suppose you should have an entity which extends this model in your system. So try to debug why that Thread entity does not have a related metadata and fix it.
Cheers!
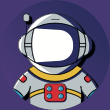
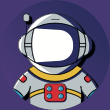
Error: Cannot instantiate abstract class FOS\MessageBundle\Entity\Thread
CRITICAL - Uncaught PHP Exception Symfony\Component\Debug\Exception\FatalErrorException: "Error: Cannot instantiate abstract class FOS\MessageBundle\Entity\Thread" at ..\vendor\friendsofsymfony\message-bundle\FOS\MessageBundle\ModelManager\ThreadManager.php line 24
How to solve this error?
Hey Ganesh,
Hm, I'd like to recommend you to watch our PHP OOP courses: https://knpuniversity.com/t... - the error you mention relates to the basics of PHP. From the error, I see you're trying to instantiate an abstract class: "FOS\MessageBundle\Entity\Thread" - but PHP does not allow it. You need to extend that class with our own, probably you also will need to implement some methods, PhpStorm could suggest you to do so, and then configure your system to use your custom class instead of the abstract.
Cheers!
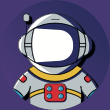
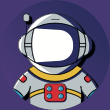
Fos Message Bundle Same as for whatsapp or its different ?
Hey Ganesh,
Well, that's a bit wide question, they are kinda different. First of all, WhatsApp is a mobile application, but with FOSMessageBundle we're talking about web application based on Symfony. And we're not talking about secured end-to-end encryption with FOSMessageBundle, at least, out of the box. I suppose you know WhatsApp well, so here's a few features that FOSMessageBundle supports:
- Support for both the Doctrine ORM and ODM for message storage
- Threaded conversations
- Spam detection support
- Soft deletion of threads
- Permissions for messaging.
I hope this helps you to understand the difference.
Cheers!
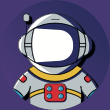
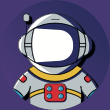
Thank u victor
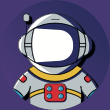
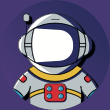
@victor
i have a four checbox category category
how can i choose multiple checkbox filter for onclick function ?
Always load all list not current filtering values..
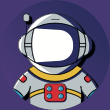
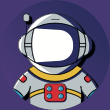
i have a four checbox category category
how can i choose multiple checkbox filter for onclick function ?
Category list
Category1(checkbox)
Category2(checkbox)
Category3(checkbox)
Experience
1 year(checkbox)
2 year(checkbox)
3 year(checkbox)
my twig file for
Checking
category name==filtering category value
my question was
1. checkbox currently choose only one (select multiple)
2. I want load only content not for all pages
3.if is it possible for without using ajax
Hey Ganesh,
See docs how to allow multiple values in form, you need to use "multiple => true": https://symfony.com/doc/cur...
So yes, it's totally possible without AJAX.
Cheers!
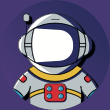
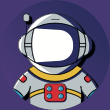
Thank U....
victor
my project was multiple user login with individual view page..unique value is mobile number...
i am login with mobile number get otp then go to home page ...
i was missing session all places.. how to include register controller and how can i include twig file "if page is current login"..
My problem was current user id in url..if url id changing automatically move to another user profile page ..
How to solve this ?
Hey Ganesh,
Wait, if it's an ID - it's unique value in the database and it is not changed over time. Why may this ID be changed? Anyway, if you need to redirect the user to another page, you need a redirect response. Here's the docs how to do it in controllers: https://symfony.com/doc/cur...
Cheers!
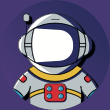
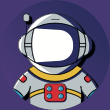
victor
Why may this ID be changed?
ans: i just want to session login.. so some developers check to change id page will be changing another user that was my problem...
i want redirect when url will be chaged
my url was
example.com /Home/user/ View?id=101
if id change 102 page moving to that page
How to solve this problem
Hey Ganesh,
So, that's just a business logic, but are we talking about Symfony? I see you use query parameters, but why? You better use placeholder in your route, so your URL will be much nicer like "example.com/home/user/view/101", i.e. your route definition will be:
/**
* @Route("/home/user/view/{userId}", name="home_user_view")
*/
public function cancellationSurveyAction(Request $request, $userId)
{
if ($this->getUser() && $this->getUser()->getId() !== $userId) {
return $this->redirectToRoute('home_user_view', ['userId' => $this->getUser()->getId()]);
}
// ...
}
It's a dead-simple, and that's the power of Symfony, but if you do not want to use placeholders - you can get that id from the request object like:
$userId = $request->query->get('id');
Cheers!
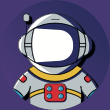
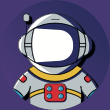
Thank U......
victor
How to remove “web/app_dev.php” from Symfony2 URLs? 7 answers
I want to remove "web" in URL. For example, I request such as the bottom URL.
http://localhost/symfony/web/mypage
But, I want to request such as the bottom URL.
http://localhost/symfony/mypage
How to solve this error ?
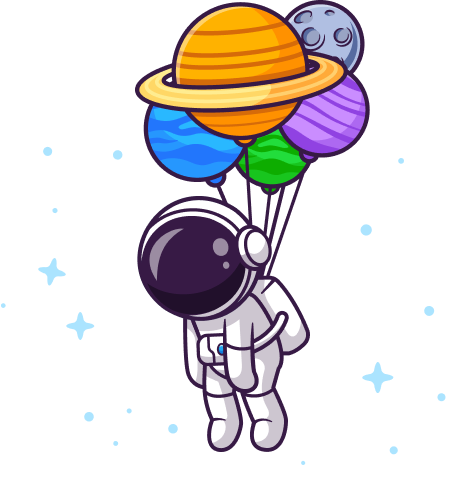
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1" // 4.1.0
}
}
@weaverryan
How Can i check website is secure or not ?
My files are indexing google ..
How to Remove this one ?
For Example !
http://example.com/foldername/
http://example.com/folderna...
http://example.com/folderna... " Here My files are listing.. How to Remove this one?"