Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Creating a Custom orderBy Query
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
Creating a Custom orderBy Query¶
Ok friends, the homepage lists every event in the order they were added to the database. We can do better! Head to EventController and replace the findAll method with a custom query that orders the events by the time property, so we can see the events that are coming up next first:
// src/Yoda/EventBundle/Controller/EventController.php
// ...
public function indexAction()
{
$em = $this->getDoctrine()->getManager();
$entities = $em
->getRepository('EventBundle:Event')
->createQueryBuilder('e')
->addOrderBy('e.time', 'ASC')
->getQuery()
->execute()
;
// ...
}
When we check the homepage, it looks about the same as before. Let’s complicate things by only showing upcoming events:
$entities = $em
->getRepository('EventBundle:Event')
->createQueryBuilder('e')
->addOrderBy('e.time', 'ASC')
->andWhere('e.time > :now')
->setParameter('now', new \DateTime())
->getQuery()
->execute()
;
This uses the parameter syntax we saw before and uses a \DateTime object to only show events after right now.
To test this, edit one of the events and set its time to a date in the past. When we head back to the homepage, we see that the event is now missing from the list!
Moving Queries to the Repository¶
This is great, but what if we want to reuse this query somewhere else? Instead of keeping the query in the controller, create a new method called getUpcomingEvents inside EventRepository and move it there:
// src/Yoda/EventBundle/Entity/EventRepository.php
// ...
/**
* @return Event[]
*/
public function getUpcomingEvents()
{
return $this
->createQueryBuilder('e')
->addOrderBy('e.time', 'ASC')
->andWhere('e.time > :now')
->setParameter('now', new \DateTime())
->getQuery()
->execute()
;
}
Now that we’re actually inside the repository, we just start by calling createQueryBuilder(). In the controller, continue to get the repository, but now just call getUpcomingEvents to use the method:
// src/Yoda/EventBundle/Controller/EventController.php
// ...
public function indexAction()
{
$em = $this->getDoctrine()->getManager();
$entities = $em
->getRepository('EventBundle:Event')
->getUpcomingEvents()
;
// ...
}
Note
The $em->getRepository('EventBundle:Event') returns our EventRepository object.
Whenever you need a custom query: create a new method in the right repository class and build it there. Don’t create queries in your controller, seriously! We want your fellow programmers to be impressed when you show them your well-organized Jedi ways.
6 Comments
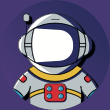
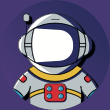
Ryan,
I'm sorry. I've found the answer. I should have just execute the stored procedure in the same way as I run in the SQL management studio.
Error I was getting was because of the permission issue. The passing credentials running Symfony to the Linked Server was not right user.
I'm using getEntityManager(), and Symfony says is deprecated since v2.1. Can you tell me the current method I should use for plain SQL query?
===
$conn = $container->get('doctrine')->getEntityManager()->getConnection();
$sql = 'SELECT * FROM yoda_event';
$stmt = $conn->prepare($sql);
$stmt->execute();
var_dump($stmt->fetchAll());die;
===
Hey!
Thanks for posting the solution to your question here for others :).
About getEntityManager() being deprecated, just use getManger() instead - it returns the same exact thing (so nothing else needs to change) - we just renamed the method in 2.1.
Cheers!
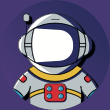
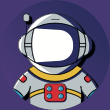
getManager() work. Thanks Ryan. :-)
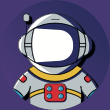
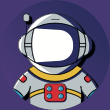
Hi everyone,
I can run a stored procedure or just plain query from my Symfony 2.6. I've written a stored procedure that calls another stored procedure that interacts with a historian. It looks the historian wants me provide a domain user. I can't make it work.
Do you know how I can use Windows domain user (my web server is IIS 7.5) as my database (pdo_sqlsrv) access credential?
I tried empty username/password and domain\user_account & password in the parameters without changing anything. It looks it's working.
Hi there!
I'll admit, I don't know anything about Windows and SQL server stuff :). But I will say that the stuff in parameters.yml (which is just being passed to the doctrine.dbal key in app/config/config.yml) is passed to the constructor of Doctrine Connection object: http://doctrine-dbal.readth.... So, if you're a bit brave, you might be able to debug it there. Also, in config.yml, here is the valid config you can put, in addition to dbname, password, etc: http://symfony.com/doc/curr... (look under doctrine.dbal.connections.default - all the keys you see there are valid for you to use).
I hope that helps, at least a little :).
Cheers!
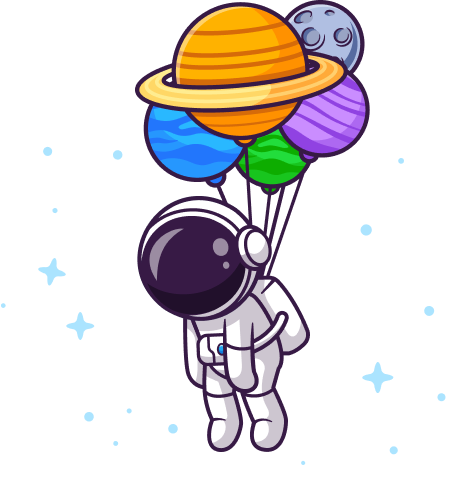
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1", // 4.1.0
"stof/doctrine-extensions-bundle": "~1.1.0" // v1.1.0
}
}
Hi Ryan,
How can I call a stored procedure in MSSQL database? Connection between my Symfony v2.6 and SQL Server 2008R2 has been established, and I can do some basics.