Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.3.3
Subscribe to download the code!Compatible PHP versions: >=5.3.3
-
This Video
Subscribe to download the video!
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Subscribe to download the script!
Adding createdAt and updatedAt Timestampable Fields
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in!
Subscribe to get
access to this tutorial plus video, code and script downloads.
Adding createdAt and updatedAt Timestampable Fields¶
Let’s do more magic! I always like to have createdAt and updatedAt fields on my database tables. A lot of times, this helps me debug any weird behavior I may see in the future.
The DoctrineExtensions library does this for us. It’s called timestampable, enable it in config.yml:
# app/config/config.yml
# ...
stof_doctrine_extensions:
orm:
default:
sluggable: true
timestampable: true
Head to the timestampable section of the documentation to see how this works. We already have the Gedmo annotation, so just copy in the created and updated properties and rename them to createdAt and updatedAt, just because I like those names better:
// src/Yoda/EventBundle/Entity/Event.php
// ...
/**
* @Gedmo\Timestampable(on="create")
* @ORM\Column(type="datetime")
*/
private $createdAt;
/**
* @Gedmo\Timestampable(on="update")
* @ORM\Column(type="datetime")
*/
private $updatedAt;
And now we’ll generate getter methods for these:
/**
* @return \DateTime
*/
public function getCreatedAt()
{
return $this->createdAt;
}
/**
* @return \DateTime
*/
public function getUpdatedAt()
{
return $this->updatedAt;
}
We can also add setter methods if we want, but we don’t need them: the library will set these for us!
Next, update the database schema to add the two new fields and then reload the fixtures:
php app/console doctrine:schema:update --force
php app/console doctrine:fixtures:load
Query for the events again:
php app/console doctrine:query:sql "SELECT * FROM yoda_event"
Nice! Both the createdAt and updatedAt columns are properly set. To avoid sadness and regret add these fields to almost every table.
10 Comments
Hey Himanshu,
That error means that when you create a User entity - you do not set the createdAt properly. You should either do this manually somewhere where you do new User()
. Or you can leverage the Timestampable feature from the bundle we're talking about in this video. If it does not work for you automatically, then it means the bundle isn't installed or configured properly.
Cheers!
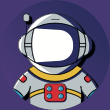
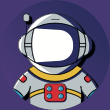
there is a typo in header:
updatdAt
Thanks! Fixed at https://github.com/knpunive...
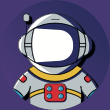
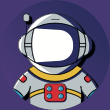
If you try to add createdAt
and updatedAt
fields to an existing database table which already has data (thus you don't want to purge and recreate schema), you will run into those errors:
[PDOException]
SQLSTATE[22007]: Invalid datetime format: 1292 Incorrect datetime value: '0000-00-00 00:00:00' for column 'created_at' at row 1
and this:
[Doctrine\DBAL\DBALException]
An exception occurred while executing 'ALTER TABLE building ADD created_at DATETIME NOT NULL, ADD updated_at DATETIME NOT NULL':
SQLSTATE[22007]: Invalid datetime format: 1292 Incorrect datetime value: '0000-00-00 00:00:00' for column 'created_at' at row 1
To avoid this problem you can communicate to MySQL default value of those columns by declaring fields like this:
@ORM\Column(name="updated_at", type="datetime", options={"default"="1970-01-01 00:00:00"})
Hey Dimitry,
You're right, thank you for sharing this! There's also another solution: use "nullable=true" for those fields.
Cheers!
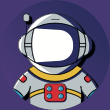
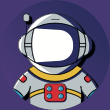
Hey Victor,
settings `nullable=true` is a good option as well (and shorter thus lazier). However I chose _not_ to use it and use "default" approach, because then I don't have to add to my codebase any special cases for when `createdAt` or `updatedAt` is null.
Hey Dimitry,
Agree! And there's another approach: if you use migrations, you can do some extra queries there and do not use `options={"default"="1970-01-01 00:00:00"} ` or `nullable=true` at all ;)
Cheers!
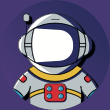
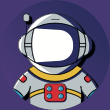
The bundle introduced here is not available for Symfony 3.x, is it?
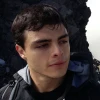
Hey Pascal Q (pascal.)
Yes, it still works, you can use it safely. You can find more information here: https://symfony.com/doc/cur...
Cheers!
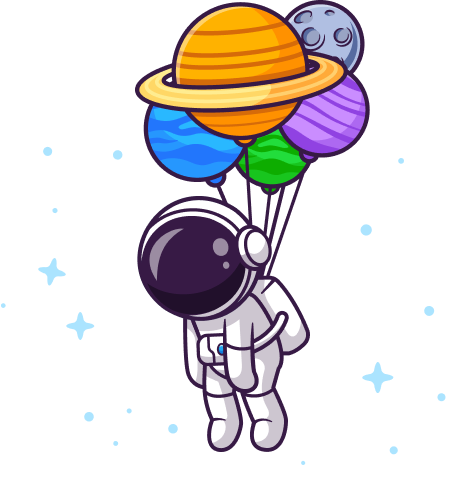
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.3.3",
"symfony/symfony": "~2.4", // v2.4.2
"doctrine/orm": "~2.2,>=2.2.3", // v2.4.2
"doctrine/doctrine-bundle": "~1.2", // v1.2.0
"twig/extensions": "~1.0", // v1.0.1
"symfony/assetic-bundle": "~2.3", // v2.3.0
"symfony/swiftmailer-bundle": "~2.3", // v2.3.5
"symfony/monolog-bundle": "~2.4", // v2.5.0
"sensio/distribution-bundle": "~2.3", // v2.3.4
"sensio/framework-extra-bundle": "~3.0", // v3.0.0
"sensio/generator-bundle": "~2.3", // v2.3.4
"incenteev/composer-parameter-handler": "~2.0", // v2.1.0
"doctrine/doctrine-fixtures-bundle": "~2.2.0", // v2.2.0
"ircmaxell/password-compat": "~1.0.3", // 1.0.3
"phpunit/phpunit": "~4.1", // 4.1.0
"stof/doctrine-extensions-bundle": "~1.1.0" // v1.1.0
}
}
I will use this
@ORM\Column(name="updated_at", type="datetime", options={"default"="1970-01-01 00:00:00"})
same error version latest symfony